C# 5.0 Programmer's Reference / Edition 1 available in Paperback, eBook
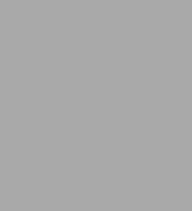
- ISBN-10:
- 1118847288
- ISBN-13:
- 9781118847282
- Pub. Date:
- 04/28/2014
- Publisher:
- Wiley
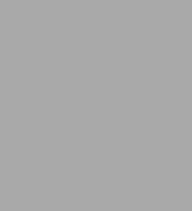
Buy New
$49.99Buy Used
$15.99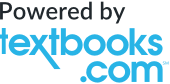
-
SHIP THIS ITEM— This item is available online through Marketplace sellers.
-
PICK UP IN STORE
Your local store may have stock of this item.
Available within 2 business hours
This item is available online through Marketplace sellers.
-
SHIP THIS ITEM
Temporarily Out of Stock Online
Please check back later for updated availability.
Overview
Well-known C# expert Rod Stephens gives novice and experienced developers a comprehensive tutorial and reference to standard C#. This new title fully covers the latest C# language standard, C# 5.0, as well as its implementation in the 2013 release of Visual Studio. The author provides exercises and solutions; and his C# Helper website will provide readers and students with ongoing support. This resource is packed with tips, tricks, tutorials, examples, and exercises and is the perfect professional companion for programmers who want to stay ahead of the game.
Author Rod Stephens is a well-known programming authority and has written more than 25 programming books covering C#, Java, VB, and other languages. His books have sold more than 150,000 copies in multiple editions. This book's useful exercises and solutions are designed to support training and higher education adoptions.
- Learn the full range of C# programming language features
- Quickly locate information for specific language features in the reference section
- Familiarize yourself with handling data types, variables, constants, and much more
- Experiment with editing and debugging code and using LINQ
Beginning through intermediate-level programmers will benefit from the accessible style of C# 5.0 Programmer's Reference and will have access to its comprehensive range of more advanced topics. Additional support and complementary material are provided at the C# Helper website, www.csharphelper.com. Stay up-to-date and improve your programming skills with this invaluable resource.
Product Details
ISBN-13: | 9781118847282 |
---|---|
Publisher: | Wiley |
Publication date: | 04/28/2014 |
Pages: | 960 |
Product dimensions: | 7.20(w) x 9.20(h) x 1.90(d) |
About the Author
Read an Excerpt
Table of Contents
Introduction xxxiiiPart I: The C# Ec osystem
Chapter 1: The C# Environment 3
Visual Studio 3
The C# Compiler 4
The CLR 6
The .NET Framework 8
Summary 9
Exercises 10
Chapter 2: Writing a First Program 11
Types of Projects 11
Console Applications 14
Windows Forms Applications 16
WPF Applications 19
Windows Store Applications 21
Summary 23
Exercises 24
Chapter 3: Program and Code File Structure 27
Hidden Files 28
Preprocessor Directives 31
#define and #undef 31
#if, #else, #elif, and #endif 33
#warning and #error 34
#line 34
#region and #endregion 35
#pragma 36
Code File Structure 37
The using Directive 38
The namespace Statement 40
Class Definitions 42
Comments 43
End-of-line and Multiline Comments 43
XML Comments 45
Summary 48
Exercises 48
Part II: C# Language Elements
Chapter 4: Data Types, Variables, and Constants 53
Data Types 54
Value Versus Reference Types 57
The var Keyword 58
Variable Declaration Syntax 59
Name 62
Attributes 62
Accessibility 63
Static, Constant, and Volatile Variables 64
Initialization 65
Classes and Structures 66
Arrays 67
Collections 68
Literal Type Characters 69
Data Type Conversion 72
Implicit Conversion 73
Casting 74
Using the as Operator 76
Casting Arrays 77
Parsing 77
Using System.Convert 78
Using System.BitConverter 78
ToString 79
Scope 79
Block Scope 79
Method Scope 80
Class Scope 81
Restricting Scope 81
Parameter Declarations 82
By Value 82
By Reference 83
For Output 84
Unusual Circumstances and Exceptions 85
Properties 86
Enumerations 88
Nullable Types 92
Delegates 93
Summary 95
Exercises 96
Chapter 5: Operators 99
Arithmetic Operators 100
Result Data Type 100
Shift Operators 101
Increment and Decrement Operators 101
Comparison Operators 102
Logical Operators 103
Bitwise Operators 105
Conditional and Null-coalescing Operators 106
Assignment Operators 107
Operator Precedence 108
The StringBuilder Class 110
DateTime and TimeSpan Operations 111
Operator Overloading 112
Comparison Operators 113
Logical Operators 115
Type Conversion Operators 115
Summary 117
Exercises 118
Chapter 6: Methods 121
Method Declarations 122
Attributes 122
Accessibility 124
Modifiers 124
Name 128
Return Type 128
Parameters 129
Implementing Interfaces 133
Extension Methods 135
Lambda Expressions 136
Expression Lambdas 136
Statement Lambdas 137
Async Lambdas 138
Variance 139
Asynchronous Methods 140
Calling EndInvoke Directly 140
Handling a Callback 141
Using Async and Await 144
Summary 146
Exercises 147
Chapter 7: Program Control Statements 151
Decision Statements 151
if-else Statements 152
switch Statements 153
Enumerated Values 156
Conditional and Null-coalescing Operators 157
Looping Statements 157
for Loops 157
Noninteger for Loops 159
while Loops 160
do Loops 161
foreach Loops 161
Enumerators 163
Iterators 164
break Statements 165
continue Statements 165
Summary 166
Exercises 166
Chapter 8: LINQ 169
Introduction to LINQ 171
Basic LINQ Query Syntax 173
from 173
where 174
orderby 175
select 175
Using LINQ Results 177
Advanced LINQ Query Syntax 178
join 178
join into 179
group by 179
Aggregate Values 181
Set Methods 182
Limiting Results 183
Other LINQ Methods 184
LINQ Extension Methods 185
Method-Based Queries 185
Method-Based Queries with Lambda Functions 187
Extending LINQ 188
LINQ to Objects 189
LINQ to XML 189
XML Literals 190
LINQ into XML 191
LINQ out of XML 192
LINQ to ADO.NET 194
LINQ to SQL and LINQ to Entities 194
LINQ to DataSet 195
PLINQ 198
Summary 200
Exercises 201
Chapter 9: Error Handling 205
Bugs Versus Undesirable Conditions 206
Catching Bugs 206
Code Contracts 209
Catching Undesirable Conditions 213
Global Exception Handling 216
try catch Blocks 220
Exception Objects 223
Throwing Exceptions 224
Rethrowing Exceptions 226
Custom Exceptions 227
Summary 229
Exercises 229
Chapter 10: Tracing and Debugging 231
The Debug Menu 232
The Debug ➪ Windows Submenu 234
The Breakpoints Window 235
The Immediate Window 237
Trace Listeners 238
Summary 240
Exercises 241
Part III: Object-Oriented Programming
Chapter 11: OO P Concepts 245
Classes 245
Encapsulation 248
Inheritance 250
Inheritance Hierarchies 251
Refinement and Abstraction 252
Has-a and Is-a Relationships 257
Adding and Modifying Class Features 257
Hiding and Overriding 258
abstract 261
sealed 262
Polymorphism 263
Summary 266
Exercises 266
Chapter 12: Classes and Structures 269
Classes 270
attributes 270
accessibility 271
abstract | sealed | static 272
partial 273
Structures 275
Value Versus Reference Types 275
Memory Requirements 276
Heap and Stack Performance 277
Object Assignment 277
Parameter Passing 277
Boxing and Unboxing 281
Constructors 282
Structure Instantiation Details 285
Garbage Collection 286
Destructors 286
Dispose 288
Events 290
Declaring Events 290
Raising Events 292
Catching Events 292
Using Event Delegate Types 292
Using Static Events 296
Hiding and Overriding Events 296
Raising Parent Class Events 296
Implementing Custom Events 297
Static Methods 298
Summary 300
Exercises 301
Chapter 13: Namespaces 303
Collisions in .NET 304
The using Directive 304
Project Templates 307
Item Templates 309
The Default Namespace 311
Making Namespaces 311
Resolving Namespaces 313
The global Namespace 314
Summary 315
Exercises 315
Chapter 14: Collection Classes 317
Arrays 318
Dimensions 318
Lower Bounds 318
Resizing 319
Speed 320
Other Array Class Features 320
System.Collections 321
ArrayList 321
StringCollection 324
NameValueCollection 325
Dictionaries 326
ListDictionary 327
Hashtable 328
HybridDictionary 329
StringDictionary 329
SortedList 329
CollectionsUtil 331
Stacks and Queues 331
Stack 331
Queue 333
Generic Collections 335
Collection Initializers 337
Iterators 338
Summary 339
Exercises 340
Chapter 15: Generics 343
Advantages of Generics 344
Defining Generics 344
Generic Constructors 345
Multiple Types 346
Constrained Types 348
Default Values 352
Instantiating Generic Classes 352
Generic Collection Classes 352
Generic Methods 352
Generics and Extension Methods 353
Summary 354
Exercises 355
Part IV: Interacting with the Environment
Chapter 16: Printing 359
Windows Forms Printing 359
Basic Printing 360
Drawing Basics 365
WPF Printing 380
Using a Paginator 381
Creating Documents 385
Summary 390
Exercises 390
Chapter 17: Configuration and Resources 393
Environment Variables 394
Setting Environment Variables 394
Using System.Environment 395
Registry 397
Configuration Files 402
Resource Files 405
Application Resources 405
Embedded Resources 406
Localization Resources 407
Summary 408
Exercises 408
Chapter 18: Streams 411
Stream 412
FileStream 414
MemoryStream 415
BinaryReader and BinaryWriter 416
TextReader and TextWriter 418
StringReader and StringWriter 419
StreamReader and StreamWriter 421
Exists, OpenText, CreateText, and AppendText 422
Custom Stream Classes 423
Summary 423
Exercises 424
Chapter 19: File System Objects 425
Filesystem Permissions 426
.NET Framework Classes 426
Directory 426
File 428
DriveInfo 430
DirectoryInfo 431
FileInfo 432
FileSystemWatcher 434
Path 436
Using the Recycle Bin 438
Using the FileIO.FileSystem Class 438
Using API Functions 439
Using Shell32.Shell 440
Summary 443
Exercises 444
Chapter 20: Networking 445
Networking Classes 446
Downloading Information 448
Downloading with WebClient 448
Downloading with WebRequest 451
Uploading Information 455
Uploading with WebClient 455
Uploading with WebRequest 455
Getting FTP Information 456
Sending E‑mail 458
Sending Text Messages 460
Summary 462
Exercises 463
Part V: Advanced Topics
Chapter 21: Regular Expressions 469
Building Regular Expressions 470
Character Escapes 471
Character Classes 472
Anchors 473
Grouping Constructs 474
Quantifiers 475
Alternation Constructs 476
Sample Regular Expressions 476
Using Regular Expressions 478
Matching Patterns 479
Finding Matches 480
Making Replacements 481
Parsing Input 482
Summary 483
Exercises 483
Chapter 22: Parallel Programming 485
Interacting with the User Interface 488
BackgroundWorker 491
TPL 492
Parallel.For 492
Parallel.ForEach 494
Parallel.Invoke 495
Tasks 496
Threads 498
Coordinating Tasks 499
Race Conditions 499
Deadlocks 501
Thread-Safe Objects 503
Summary 504
Exercises 505
Chapter 23: ADO .NET 509
Selecting a Database 510
Using Bound Controls 511
Making a Data Source 511
Making a DataGridView Interface 516
Making a Details Interface 518
Making a DataGrid Interface 518
Loading DataSets 525
Using ADO.NET 527
Summary 530
Exercises 531
Chapter 24: XML 533
Basic XML Syntax 534
Writing XML Data 538
XmlWriter 538
Document Object Model 541
XML Literals 547
Reading XML Data 547
XmlTextReader 547
Document Object Model 550
Related Technologies 551
XPath 552
XSLT 555
Summary 559
Exercises 559
Chapter 25: Serialization 563
XML Serialization 564
Performing Serialization 565
Controlling Serialization 569
JSON Serialization 573
Performing Serialization 574
Controlling Serialization 575
Binary Serialization 576
Summary 578
Exercises 578
Chapter 26: Reflection 581
Learning About Classes 582
Getting and Setting Properties 585
Getting Assembly Information 589
Invoking Methods 591
Running Scripts 593
Summary 597
Exercises 597
Chapter 27: Cryptography 601
Cryptographic Operations 602
Randomness 603
Using Random Numbers for Encryption 604
Using Encryption for Random Numbers 604
Cryptographically Secure Randomness 604
Generating Random Numbers 605
Symmetric Key Encryption 608
Simple Encryption and Decryption 608
Keys and Initialization Vectors 612
Generating Key and IV Values 613
Asymmetric Key Encryption 614
Creating, Saving, and Retrieving Keys 615
Encrypting Data 616
Decrypting Data 616
Example Encryption 617
Summary 619
Exercises 619
Part VI: Appendices
Appendix A: Solutions to Exercises 625
Chapter 1 625
Chapter 2 626
Chapter 3 627
Chapter 4 629
Chapter 5 631
Chapter 6 635
Chapter 7 638
Chapter 8 642
Chapter 9 648
Chapter 10 655
Chapter 11 659
Chapter 12 665
Chapter 13 667
Chapter 14 668
Chapter 15 673
Chapter 16 678
Chapter 17 682
Chapter 18 685
Chapter 19 689
Chapter 20 694
Chapter 21 702
Chapter 22 706
Chapter 23 711
Chapter 24 713
Chapter 25 720
Chapter 26 725
Chapter 27 728
Appendix B: Data Types 733
Casting and Converting Values 734
Widening and Narrowing Conversions 735
Converting Objects 735
The as Operator 735
Casting Arrays 736
Parsing Values 736
Appendix C: Variable Declarations 737
Initialization Expressions 738
Using 739
Enumerated Type Declarations 739
Appendix d: Constant Declarations 741
Appendix e: Operators 743
Arithmetic Operators 743
Comparison Operators 744
Logical Operators 744
Bitwise Operators 745
Assignment Operators 745
Conditional and Null-coalescing Operators 745
Operator Precedence 745
DateTime and TimeSpan Operators 747
Operator Overloading 748
Appendix f: Method Declarations 749
Methods 749
Property Procedures 750
Lambda Functions and Expressions 750
Extension Methods 751
Appendix g: Useful Attributes 753
Useful XML Serialization Attributes 753
Useful JSON Serialization Attributes 754
Binary Serialization Attributes 754
Other Useful Attributes 755
Appendix h: Control Statements 757
Decision Statements 757
if-else Statements 757
switch 757
Conditional and Null-coalescing Operators 758
Looping Statements 758
for Loops 758
while Loops 759
do Loops 759
foreach Loops 759
Enumerators 760
Iterators 760
break and continue Statements 760
Appendix i: Error Handling 761
Throwing Exceptions 762
Appendix j: LINQ 763
Basic LINQ Query Syntax 763
from 763
where 764
orderby 764
select 764
join 765
group by 765
Aggregate Values 766
Limiting Results 766
LINQ Functions 767
LINQ to XML 768
XML Literals 768
LINQ into XML 768
LINQ out of XML 769
LINQ to ADO.NET 770
PLINQ 771
Appendix k: Classes and Structures 773
Classes 773
Structures 774
Constructors 774
Destructors 774
Events 775
Appendix l: Collection Classes 777
Arrays 777
Simple Arrays 777
Array Objects 778
Collections 779
Specialized Collections 779
Generic Collections 779
Collection Initializers 780
Iterators 780
Appendix m: Generic Declarations 783
Generic Classes 783
Generic Methods 784
Appendix n: Printing and Graphics 785
Windows Forms Printing 785
Printing Steps 785
Graphics Namespaces 786
Drawing Graphics 787
WPF Printing 795
Using a Paginator 795
Creating Documents 796
Appendix o: Useful Exception Classes 799
Standard Exception Classes 799
Custom Exception Classes 802
Appendix p: Date and Time Format Specifiers 803
Standard Format Specifiers 803
Custom Format Specifiers 804
Appendix q: Other Format Specifiers 807
Standard Numeric Format Specifiers 807
Custom Numeric Format Specifiers 809
Numeric Formatting Sections 809
Composite Formatting 810
Enumerated Type Formatting 811
Appendix r: Streams 813
Stream Class Summary 813
Stream 814
BinaryReader and BinaryWriter 815
TextReader and TextWriter 817
StringReader and StringWriter 818
StreamReader and StreamWriter 818
Text File Stream Methods 818
Appendix s: Filesystem Classes 821
Framework Classes 821
Directory 821
File 823
DriveInfo 825
DirectoryInfo 826
FileInfo 827
FileSystemWatcher 829
Path 830
Special Folders 832
Recycle Bin 832
FileIO.FileSystem 833
API Functions 833
Shell32.Shell 834
Appendix t: Regular Expressions 835
Creating Regular Expressions 835
Character Escapes 835
Character Classes 836
Anchors 836
Regular Expression Options 837
Grouping Constructs 838
Quantifiers 838
Alternation Constructs 838
Sample Regular Expressions 839
Using Regular Expressions 839
Matching Patterns 840
Finding Matches 840
Making Replacements 841
Appendix u: Parallel Programming 843
Interacting with the User Interface 843
PLINQ 843
BackgroundWorker 844
TPL 844
Parallel.For 845
Parallel.ForEach 845
Parallel.Invoke 845
Tasks 845
Threads 847
Appendix v: XML 849
Special Characters 849
Writing XML Data 849
XmlWriter 850
Document Object Model 851
XML Literals 854
Reading XML Data 854
XmlTextReader 854
Document Object Model 856
Related Technologies 856
XPath 857
XSLT 858
Appendix w: Serialization 859
XML Serialization 859
Controlling Serialization 860
JSON Serialization 861
Performing Serialization 861
Controlling Serialization 862
Binary Serialization 863
Appendix x: Reflection 865
Type 865
MemberInfo 870
EventInfo 870
MethodInfo 871
FieldInfo 873
PropertyInfo 874
ParameterInfo 874
Index 877