iOS 7 Programming Cookbook / Edition 1 available in Paperback
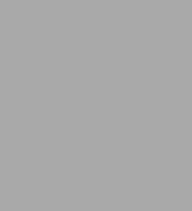
iOS 7 Programming Cookbook / Edition 1
- ISBN-10:
- 1449372422
- ISBN-13:
- 9781449372422
- Pub. Date:
- 10/30/2013
- Publisher:
- O'Reilly Media, Incorporated
- ISBN-10:
- 1449372422
- ISBN-13:
- 9781449372422
- Pub. Date:
- 10/30/2013
- Publisher:
- O'Reilly Media, Incorporated
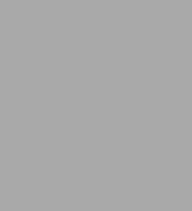
iOS 7 Programming Cookbook / Edition 1
Paperback
Buy New
$54.99Buy Used
$38.69-
-
SHIP THIS ITEM
Temporarily Out of Stock Online
Please check back later for updated availability.
-
Overview
Overcome the vexing issues you’re likely to face when creating apps for the iPhone, iPad, or iPod touch. With new and thoroughly revised recipes in this updated cookbook, you’ll quickly learn the steps necessary to work with the iOS 7 SDK, including solutions for bringing real-world physics and movement to your apps with UIKit Dynamics APIs.
You’ll learn hundreds of techniques for storing and protecting data, sending and receiving notifications, enhancing and animating graphics, managing files and folders, and many other options. Each recipe includes sample code you can use right away.
- Create vibrant and lifelike user interfaces with UIKit Dynamics
- Use the Keychain to protect your app’s data
- Develop location-aware and multitasking-aware apps
- Work with iOS 7’s audio and video APIs
- Use Event Kit UI to manage calendars, dates, and events
- Take advantage of the accelerometer and the gyroscope
- Integrate iCloud into your apps
- Define the layout of UI elements with Auto Layout
- Get working examples for implementing gesture recognizers
- Retrieve and manipulate contacts and groups from the Address Book
- Determine a camera’s availability and access the Photo Library
Product Details
ISBN-13: | 9781449372422 |
---|---|
Publisher: | O'Reilly Media, Incorporated |
Publication date: | 10/30/2013 |
Edition description: | Second Edition |
Pages: | 1056 |
Product dimensions: | 7.00(w) x 9.20(h) x 1.90(d) |
About the Author
Vandad Nahavandipoor is currently an iOS programmer for a leading digital media distributor in London, United Kingdom. He has led an international team of more than 30 iOS developers in his previous job. Some of the projects he has lead include the NatWest and the RBS iOS apps running on millions of iPhones and iPads in the UK. Vandad received his B.Sc and M.Sc in Information Technology for E-Commerce from the University of Sussex in England.
Vandad's programming experience started when he first learned Basic on his father's Commodore 64. He then took this experience and applied it on his uncle's Intel 186 computer, running Basic on DOS. At this point, he found programming for personal computers exciting indeed and moved on to learn Object Pascal. This allowed him to learn Borland Delphi quite easily. He wrote a short 400 pages book on Borland Delphi and dedicated the book to Borland. From then, he picked up x86 Assembly programming and wrote a hobby 32-bit operating system named Vandior. It wasn't until late 2007 when iOS programming became his main focus.
Aside from programming, Vandad is a road cyclist, enjoying friendly competition in cyclosportive events in England and traveling long distance on his bicycle to other European countries every now and then. He also enjoys tinkering with his piano and playing electric guitar. Some of his electric guitar performances are available on YouTube.
Table of Contents
Preface;
Audience;
Organization of This Book;
Additional Resources;
Conventions Used in This Book;
Using Code Examples;
Safari® Books Online;
How to Contact Us;
Acknowledgments;
Chapter 1: Implementing Controllers and Views;
1.1 Introduction;
1.2 Displaying Alerts with UIAlertView;
1.3 Creating and Using Switches with UISwitch;
1.4 Customizing the UISwitch;
1.5 Picking Values with the UIPickerView;
1.6 Picking the Date and Time with UIDatePicker;
1.7 Implementing Range Pickers with UISlider;
1.8 Customizing the UISlider;
1.9 Grouping Compact Options with UISegmentedControl;
1.10 Presenting and Managing Views with UIViewController;
1.11 Presenting Sharing Options with UIActivityViewController;
1.12 Presenting Custom Sharing Options with UIActivityViewController;
1.13 Implementing Navigation with UINavigationController;
1.14 Manipulating a Navigation Controller’s Array of View Controllers;
1.15 Displaying an Image on a Navigation Bar;
1.16 Adding Buttons to Navigation Bars Using UIBarButtonItem;
1.17 Presenting Multiple View Controllers with UITabBarController;
1.18 Displaying Static Text with UILabel;
1.19 Customizing the UILabel;
1.20 Accepting User Text Input with UITextField;
1.21 Displaying Long Lines of Text with UITextView;
1.22 Adding Buttons to the User Interface with UIButton;
1.23 Displaying Images with UIImageView;
1.24 Creating Scrollable Content with UIScrollView;
1.25 Loading Web Pages with UIWebView;
1.26 Displaying Progress with UIProgressView;
1.27 Constructing and Displaying Styled Texts;
1.28 Presenting Master-Detail Views with UISplitViewController;
1.29 Enabling Paging with UIPageViewController;
1.30 Displaying Popovers with UIPopoverController;
Chapter 2: Creating Dynamic and Interactive User Interfaces;
2.1 Introduction;
2.2 Adding Gravity to Your UI Components;
2.3 Detecting and Reacting to Collisions Between UI Components;
2.4 Animating Your UI Components with a Push;
2.5 Attaching Multiple Dynamic Items to Each Other;
2.6 Adding a Dynamic Snap Effect to Your UI Components;
2.7 Assigning Characteristics to Your Dynamic Effects;
Chapter 3: Auto Layout and the Visual Format Language;
3.1 Introduction;
3.2 Placing UI Components in the Center of the Screen;
3.3 Defining Horizontal and Vertical Constraints with the Visual Format Language;
3.4 Utilizing Cross View Constraints;
3.5 Configuring Auto Layout Constraints in Interface Builder;
Chapter 4: Constructing and Using Table Views;
4.1 Introduction;
4.2 Populating a Table View with Data;
4.3 Using Different Types of Accessories in a Table View Cell;
4.4 Creating Custom Table View Cell Accessories;
4.5 Enabling Swipe Deletion of Table View Cells;
4.6 Constructing Headers and Footers in Table Views;
4.7 Displaying Context Menus on Table View Cells;
4.8 Moving Cells and Sections in Table Views;
4.9 Deleting Cells and Sections from Table Views;
4.10 Utilizing the UITableViewController for Easy Creation of Table Views;
4.11 Displaying a Refresh Control for Table Views;
Chapter 5: Building Complex Layouts with Collection Views;
5.1 Introduction;
5.2 Constructing Collection Views;
5.3 Assigning a Data Source to a Collection View;
5.4 Providing a Flow Layout to a Collection View;
5.5 Providing Basic Content to a Collection View;
5.6 Feeding Custom Cells to Collection Views Using .xib Files;
5.7 Handling Events in Collection Views;
5.8 Providing a Header and a Footer in a Flow Layout;
5.9 Adding Custom Interactions to Collection Views;
5.10 Providing Contextual Menus on Collection View Cells;
Chapter 6: Storyboards;
6.1 Introduction;
6.2 Adding a Navigation Controller to a Storyboard;
6.3 Passing Data from One Screen to Another;
6.4 Adding a Tab Bar Controller to a Storyboard;
6.5 Introducing Custom Segue Transitions to Your Storyboard;
6.6 Placing Images and Other UI Components on Storyboards;
Chapter 7: Concurrency;
7.1 Introduction;
7.2 Constructing Block Objects;
7.3 Accessing Variables in Block Objects;
7.4 Invoking Block Objects;
7.5 Performing UI-Related Tasks with GCD;
7.6 Executing Non-UI Related Tasks Synchronously with GCD;
7.7 Executing Non-UI Related Tasks Asynchronously with GCD;
7.8 Performing Tasks after a Delay with GCD;
7.9 Performing a Task Only Once with GCD;
7.10 Grouping Tasks Together with GCD;
7.11 Constructing Your Own Dispatch Queues with GCD;
7.12 Running Tasks Synchronously with Operations;
7.13 Running Tasks Asynchronously with Operations;
7.14 Creating Dependency Between Operations;
7.15 Creating Timers;
7.16 Creating Concurrency with Threads;
7.17 Invoking Background Methods;
7.18 Exiting Threads and Timers;
Chapter 8: Security;
8.1 Introduction;
8.2 Enabling Security and Protection for Your Apps;
8.3 Storing Values in the Keychain;
8.4 Finding Values in the Keychain;
8.5 Updating Existing Values in the Keychain;
8.6 Deleting Exiting Values in the Keychain;
8.7 Sharing Keychain Data Between Multiple Apps;
8.8 Writing to and Reading Keychain Data from iCloud;
8.9 Storing Files Securely in the App Sandbox;
8.10 Securing Your User Interface;
Chapter 9: Core Location and Maps;
9.1 Introduction;
9.2 Creating a Map View;
9.3 Handling the Events of a Map View;
9.4 Pinpointing the Location of a Device;
9.5 Displaying Pins on a Map View;
9.6 Displaying Pins with Different Colors on a Map View;
9.7 Displaying Custom Pins on a Map View;
9.8 Converting Meaningful Addresses to Longitude and Latitude;
9.9 Converting Longitude and Latitude to a Meaningful Address;
9.10 Searching on a Map View;
9.11 Displaying Directions on the Map;
Chapter 10: Implementing Gesture Recognizers;
10.1 Introduction;
10.2 Detecting Swipe Gestures;
10.3 Detecting Rotation Gestures;
10.4 Detecting Panning and Dragging Gestures;
10.5 Detecting Long-Press Gestures;
10.6 Detecting Tap Gestures;
10.7 Detecting Pinch Gestures;
Chapter 11: Networking, JSON, XML, and Sharing;
11.1 Introduction;
11.2 Downloading Asynchronously with NSURLConnection;
11.3 Handling Timeouts in Asynchronous Connections;
11.4 Downloading Synchronously with NSURLConnection;
11.5 Modifying a URL Request with NSMutableURLRequest;
11.6 Sending HTTP GET Requests with NSURLConnection;
11.7 Sending HTTP POST Requests with NSURLConnection;
11.8 Sending HTTP DELETE Requests with NSURLConnection;
11.9 Sending HTTP PUT Requests with NSURLConnection;
11.10 Serializing Arrays and Dictionaries into JSON;
11.11 Deserializing JSON into Arrays and Dictionaries;
11.12 Integrating Social Sharing into Your Apps;
11.13 Parsing XML with NSXMLParser;
Chapter 12: Audio and Video;
12.1 Introduction;
12.2 Playing Audio Files;
12.3 Handling Interruptions While Playing Audio;
12.4 Recording Audio;
12.5 Handling Interruptions While Recording Audio;
12.6 Playing Audio over Other Active Sounds;
12.7 Playing Video Files;
12.8 Capturing Thumbnails from Video Files;
12.9 Accessing the Music Library;
Chapter 13: Address Book;
13.1 Introduction;
13.2 Requesting Access to the Address Book;
13.3 Retrieving a Reference to an Address Book;
13.4 Retrieving All the People in the Address Book;
13.5 Retrieving Properties of Address Book Entries;
13.6 Inserting a Person Entry into the Address Book;
13.7 Inserting a Group Entry into the Address Book;
13.8 Adding Persons to Groups;
13.9 Searching the Address Book;
13.10 Retrieving and Setting a Person's Address Book Image;
Chapter 14: Files and Folder Management;
14.1 Introduction;
14.2 Finding the Paths of the Most Useful Folders on Disk;
14.3 Writing to and Reading from Files;
14.4 Creating Folders on Disk;
14.5 Enumerating Files and Folders;
14.6 Deleting Files and Folders;
14.7 Saving Objects to Files;
Chapter 15: Camera and the Photo Library;
15.1 Introduction;
15.2 Detecting and Probing the Camera;
15.3 Taking Photos with the Camera;
15.4 Taking Videos with the Camera;
15.5 Storing Photos in the Photo Library;
15.6 Storing Videos in the Photo Library;
15.7 Retrieving Photos and Videos from the Photo Library;
15.8 Retrieving Assets from the Assets Library;
15.9 Editing Videos on an iOS Device;
Chapter 16: Multitasking;
16.1 Introduction;
16.2 Detecting the Availability of Multitasking;
16.3 Completing a Long-Running Task in the Background;
16.4 Adding Background Fetch Capabilities to Your Apps;
16.5 Playing Audio in the Background;
16.6 Handling Location Changes in the Background;
16.7 Saving and Loading the State of Multitasking Apps;
16.8 Handling Network Connections in the Background;
16.9 Opting Out of Multitasking;
Chapter 17: Notifications;
17.1 Introduction;
17.2 Sending Notifications;
17.3 Listening for and Reacting to Notifications;
17.4 Listening and Reacting to Keyboard Notifications;
17.5 Scheduling Local Notifications;
17.6 Listening for and Reacting to Local Notifications;
17.7 Handling Local System Notifications;
17.8 Setting Up Your App for Push Notifications;
17.9 Delivering Push Notifications to Your App;
17.10 Reacting to Push Notifications;
Chapter 18: Core Data;
18.1 Introduction;
18.2 Creating a Core Data Model with Xcode;
18.3 Generating Class Files for Core Data Entities;
18.4 Creating and Saving Data Using Core Data;
18.5 Reading Data from Core Data;
18.6 Deleting Data from Core Data;
18.7 Sorting Data in Core Data;
18.8 Boosting Data Access in Table Views;
18.9 Implementing Relationships in Core Data;
18.10 Fetching Data in the Background;
18.11 Using Custom Data Types in Your Core Data Model;
Chapter 19: Dates, Calendars, and Events;
19.1 Introduction;
19.2 Requesting Permission to Access Calendars;
19.3 Retrieving Calendar Groups on an iOS Device;
19.4 Adding Events to Calendars;
19.5 Accessing the Contents of Calendars;
19.6 Removing Events from Calendars;
19.7 Adding Recurring Events to Calendars;
19.8 Retrieving the Attendees of an Event;
19.9 Adding Alarms to Calendars;
19.10 Handling Event Changed Notifications;
19.11 Presenting Event View Controllers;
19.12 Presenting Event Edit View Controllers;
Chapter 20: Graphics and Animations;
20.1 Introduction;
20.2 Enumerating and Loading Fonts;
20.3 Drawing Text;
20.4 Constructing, Setting, and Using Colors;
20.5 Drawing Images;
20.6 Constructing Resizable Images;
20.7 Drawing Lines;
20.8 Constructing Paths;
20.9 Drawing Rectangles;
20.10 Adding Shadows to Shapes;
20.11 Drawing Gradients;
20.12 Moving Shapes Drawn on Graphic Contexts;
20.13 Scaling Shapes Drawn on Graphic Contexts;
20.14 Rotating Shapes Drawn on Graphic Contexts;
20.15 Animating and Moving Views;
20.16 Animating and Scaling Views;
20.17 Animating and Rotating Views;
20.18 Capturing a Screenshot of Your View into an Image;
Chapter 21: Core Motion;
21.1 Introduction;
21.2 Detecting the Availability of an Accelerometer;
21.3 Detecting the Availability of a Gyroscope;
21.4 Retrieving Accelerometer Data;
21.5 Detecting Shakes on an iOS Device;
21.6 Retrieving Gyroscope Data;
Chapter 22: iCloud;
22.1 Introduction;
22.2 Setting Up Your App for iCloud;
22.3 Storing and Synchronizing Dictionaries in iCloud;
22.4 Creating and Managing Folders for Apps in iCloud;
22.5 Searching for Files and Folders in iCloud;
22.6 Storing User Documents in iCloud;
22.7 Managing the State of Documents in iCloud;
Chapter 23: Pass Kit;
23.1 Introduction;
23.2 Creating Pass Kit Certificates;
23.3 Creating Pass Files;
23.4 Providing Icons and Images for Passes;
23.5 Preparing Your Passes for Digital Signature;
23.6 Signing Passes Digitally;
23.7 Distributing Passes Using Email;
23.8 Distributing Passes Using Web Services;
23.9 Enabling Your iOS Apps to Access Passes on iOS Devices;
23.10 Interacting with Passbook Programmatically;
Colophon;