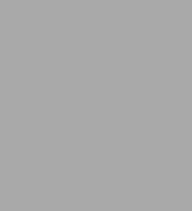
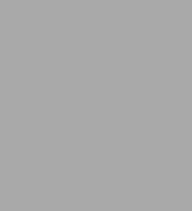
Paperback
-
PICK UP IN STORECheck Availability at Nearby Stores
Available within 2 business hours
Related collections and offers
Overview
In ASP.NET Core Security, you will learn how to:
Understand and recognize common web app attacks
Implement attack countermeasures
Use testing and scanning tools and libraries
Activate built-in browser security features from ASP.NET
Take advantage of .NET and ASP.NET Core security APIs
Manage passwords to minimize damage from a data leak
Securely store application secrets
ASP.NET Core Security teaches you the skills and countermeasures you need to keep your ASP.NET Core apps secure from the most common web application attacks. With this collection of practical techniques, you will be able to anticipate risks and introduce practices like testing as regular security checkups. You’ll be fascinated as the author explores real-world security breaches, including rogue Firefox extensions and Adobe password thefts. The examples present universal security best practices with a sharp focus on the unique needs of ASP.NET Core applications.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the technology
Your ASP.NET Core applications are under attack now. Are you ready? Th ere are specific countermeasures you can apply to keep your company out of the headlines. This book demonstrates exactly how to secure ASP.NET Core web applications, including safe browser interactions, recognizing common threats, and deploying the framework’s unique security APIs.
About the book
ASP.NET Core Security is a realistic guide to securing your web applications. It starts on the dark side, exploring case studies of cross-site scripting, SQL injection, and other weapons used by hackers. As you go, you’ll learn how to implement countermeasures, activate browser security features, minimize attack damage, and securely store application secrets. Detailed ASP.NET Core code samples in C# show you how each technique looks in practice.
What's inside
Understand and recognize common web app attacks
Testing tools, helper libraries, and scanning tools
Activate built-in browser security features
Take advantage of .NET and ASP.NET Core security APIs
Manage passwords to minimize damage from a data leak
About the reader
For experienced ASP.NET Core web developers.
About the author
Christian Wenz is a web pioneer, consultant, and entrepreneur.
Table of Contents
PART 1 FIRST STEPS
1 On web application security
PART 2 MITIGATING COMMON ATTACKS
2 Cross-site scripting (XSS)
3 Attacking session management
4 Cross-site request forgery
5 Unvalidated data
6 SQL injection (and other injections)
PART 3 SECURE DATA STORAGE
7 Storing secrets
8 Handling passwords
PART 4 CONFIGURATION
9 HTTP headers
10 Error handling
11 Logging and health checks
PART 5 AUTHENTICATION AND AUTHORIZATION
12 Securing web applications with ASP.NET Core Identity
13 Securing APIs and single page applications
PART 6 SECURITY AS A PROCESS
14 Secure dependencies
15 Audit tools
16 OWASP Top 10
Product Details
ISBN-13: | 9781633439986 |
---|---|
Publisher: | Manning |
Publication date: | 07/26/2022 |
Pages: | 368 |
Product dimensions: | 7.38(w) x 9.25(h) x 1.60(d) |
About the Author
Table of Contents
Preface xiii
Acknowledgments xv
About this book xvi
About the author xix
About the cover illustration xx
Part 1 First steps 1
1 On web application security 3
1.1 ASP.NET Core: History and options 4
ASP.NET Core version history 4
MVC 5
Razor Pages 7
Web API 8
Blazor 10
1.2 Identifying and mitigating threats 11
Web application components 11
Defense in depth 12
1.3 Security-related APIs 13
1.4 Security is important 14
Part 2 Mitigating common attacks 15
2 Cross-site scripting (XSS) 17
2.1 Anatomy of a cross-site scripting attack 18
2.2 Preventing cross-site scripting 24
Understanding the same-origin policy 25
Escaping HTML 26
Escaping in a different context 29
2.3 Content Security Policy 32
Sample application 33
How Content Security Policy works 34
Refactoring applications for Content Security Policy 36
Content Security Policy best practices 43
Content Security Policy Level 3 features 46
2.4 More browser safeguards 48
3 Attacking session management 51
3.1 Anatomy of a session management attack 52
Stealing session cookies 54
Cookies and session management 55
3.2 ASP.NET Core cookie and session settings 58
3.3 Enforcing HTTPS 61
3.4 Detecting session hijacking 65
4 Cross-site request forgery 67
4.1 Anatomy of a cross-site request forgery attack 68
4.2 Cross-site request forgery countermeasures 75
Making the HTTP request unpredictable 76
Securing the session cookie 79
4.3 Clickjacking 80
4.4 Cross-origin resource sharing 84
5 Unvalidated data 91
5.1 Looking at HTTP 92
5.2 ASP.NET Core validation 95
5.3 Mass assignment 99
5.4 Secure deserialization 106
6 SQL injection (and other injections) 110
6.1 Anatomy of an SQL injection attack 111
6.2 Prepared statements 113
6.3 Entity Framework Core 116
6.4 XML external entities 118
6.5 Other injections 120
Part 3 Secure data storage 123
7 Storing secrets 125
7.1 On encryption 126
7.2 Secret Manager 128
7.3 The appsettings.json file 131
7.4 Storing secrets in the cloud 134
Storing secrets in Azure 135
Storing secrets in AWS 138
Storing secrets in Google Cloud 141
7.5 Using the data protection API 143
7.6 Storing secrets locally with Blazor 147
8 Handling passwords 151
8.1 From data leak to password theft 152
8.2 Implementing password hashing 156
MD5 (and why not to use it) 157
PBKDF2 160
Argon2 163
Scrypt 165
Bcrypt 167
8.3 Analyzing ASP.NET Core templates 168
Part 4 Configuration 173
9 HTTP headers 175
9.1 Hiding server information 177
9.2 Browser security headers 179
Referrer Policy 179
Feature and permissions policy 182
Preventing content sniffing 185
Cross-origin policies 185
Further headers 186
10 Error handling 189
10.1 Error pages for web applications 190
Custom error pages 192
Status code error pages 194
10.2 Handling errors in APIs 196
11 Logging and health checks 200
11.1 Health checks 201
Health check setup 201
Advanced heath checks 204
Formatting the output 206
Health checks UI 206
11.2 Logging 211
Creating log entries 211
Log levels 213
Log scopes 215
Part 5 Authentication and Authorization 219
12 Securing web applications with ASP.NET Core Identity 221
12.1 ASP.NET Core Identity setup 222
12.2 ASP.NET Core Identity fundamentals 226
12.3 Advanced ASP.NET Core Identity features 234
Password options 234
Cookie options 236
Locking out users 237
Working with claims 238
Two-factor authentication 241
Authenticating with external providers 245
13 Securing APIs and single page applications 251
13.1 Securing APIs with tokens 252
13.2 OAuth and OpenID Connect 262
OAuth vs. OpenID Connect 262
OAuth flows 264
13.3 Securing applications 266
Third-party tools 267
Client credentials 269
Authorization code + PKCF 274
SPAs and BFF 282
Part 6 Security as a process 295
14 Secure dependencies 297
14.1 Using npm audit 298
14.2 Keeping NuGet dependencies up-to-date 302
15 Audit tools 308
15.1 Finding vulnerabilities 309
15.2 OWASPZAP 310
15.3 Security Code Scan 317
15.4 GitHub Advanced Security 320
16 OWASP Top JO 325
16.1 OWASP Top 10 326
Top 10 creation process 326
#1 Broken access control 328
#2 Cryptographic failures 328
#3 Injection 329
#4 Insecure design 329
#5 Security misconfiguration 330
#6 Vulnerable and outdated components 330
#7 Identification and authentication failures 330
#8 Software and data integrity failures 330
#9 Security logging and monitoring failures 331
#10 Server-side request forgery 331
16.2 OWASP API Top 10 332
16.3 Other lists 333
Index 337