Bare Metal C: Embedded Programming for the Real World
Bare Metal C teaches you to program embedded systems with the C programming language. You’ll learn how embedded programs interact with bare hardware directly, go behind the scenes with the compiler and linker, and learn C features that are important for programming regular computers.
Bare Metal C will teach you how to program embedded devices with the C programming language. For embedded system programmers who want precise and complete control over the system they are using, this book pulls back the curtain on what the compiler is doing for you so that you can see all the details of what's happening with your program.
The first part of the book teaches C basics with the aid of a low-cost, widely available bare metal system (the Nucleo Arm evaluation system), which gives you all the tools needed to perform basic embedded programming. As you progress through the book you’ll learn how to integrate serial input/output (I/O) and interrupts into your programs. You’ll also learn what the C compiler and linker do behind the scenes, so that you’ll be better able to write more efficient programs that maximize limited memory. Finally, you’ll learn how to use more complex, memory hungry C features like dynamic memory, file I/O, and floating-point numbers.
Topic coverage includes:The basic program creation process Simple GPIO programming (blink an LED) Writing serial device drivers The C linker and preprocessor Decision and control statements Numbers, arrays, pointers, strings, and complex data types Local variables and procedures Dynamic memory File and raw I/O Floating-point numbers Modular programming
1140907155
Bare Metal C will teach you how to program embedded devices with the C programming language. For embedded system programmers who want precise and complete control over the system they are using, this book pulls back the curtain on what the compiler is doing for you so that you can see all the details of what's happening with your program.
The first part of the book teaches C basics with the aid of a low-cost, widely available bare metal system (the Nucleo Arm evaluation system), which gives you all the tools needed to perform basic embedded programming. As you progress through the book you’ll learn how to integrate serial input/output (I/O) and interrupts into your programs. You’ll also learn what the C compiler and linker do behind the scenes, so that you’ll be better able to write more efficient programs that maximize limited memory. Finally, you’ll learn how to use more complex, memory hungry C features like dynamic memory, file I/O, and floating-point numbers.
Topic coverage includes:
Bare Metal C: Embedded Programming for the Real World
Bare Metal C teaches you to program embedded systems with the C programming language. You’ll learn how embedded programs interact with bare hardware directly, go behind the scenes with the compiler and linker, and learn C features that are important for programming regular computers.
Bare Metal C will teach you how to program embedded devices with the C programming language. For embedded system programmers who want precise and complete control over the system they are using, this book pulls back the curtain on what the compiler is doing for you so that you can see all the details of what's happening with your program.
The first part of the book teaches C basics with the aid of a low-cost, widely available bare metal system (the Nucleo Arm evaluation system), which gives you all the tools needed to perform basic embedded programming. As you progress through the book you’ll learn how to integrate serial input/output (I/O) and interrupts into your programs. You’ll also learn what the C compiler and linker do behind the scenes, so that you’ll be better able to write more efficient programs that maximize limited memory. Finally, you’ll learn how to use more complex, memory hungry C features like dynamic memory, file I/O, and floating-point numbers.
Topic coverage includes:The basic program creation process Simple GPIO programming (blink an LED) Writing serial device drivers The C linker and preprocessor Decision and control statements Numbers, arrays, pointers, strings, and complex data types Local variables and procedures Dynamic memory File and raw I/O Floating-point numbers Modular programming
Bare Metal C will teach you how to program embedded devices with the C programming language. For embedded system programmers who want precise and complete control over the system they are using, this book pulls back the curtain on what the compiler is doing for you so that you can see all the details of what's happening with your program.
The first part of the book teaches C basics with the aid of a low-cost, widely available bare metal system (the Nucleo Arm evaluation system), which gives you all the tools needed to perform basic embedded programming. As you progress through the book you’ll learn how to integrate serial input/output (I/O) and interrupts into your programs. You’ll also learn what the C compiler and linker do behind the scenes, so that you’ll be better able to write more efficient programs that maximize limited memory. Finally, you’ll learn how to use more complex, memory hungry C features like dynamic memory, file I/O, and floating-point numbers.
Topic coverage includes:
49.99
In Stock
5
1
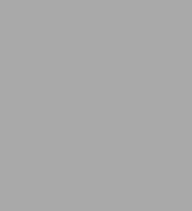
Bare Metal C: Embedded Programming for the Real World
304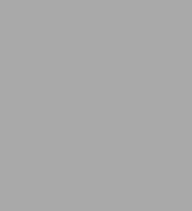
Bare Metal C: Embedded Programming for the Real World
304
49.99
In Stock
Product Details
ISBN-13: | 9781718501621 |
---|---|
Publisher: | No Starch Press |
Publication date: | 08/02/2022 |
Pages: | 304 |
Product dimensions: | 6.90(w) x 9.10(h) x 0.80(d) |
About the Author
From the B&N Reads Blog