5
1
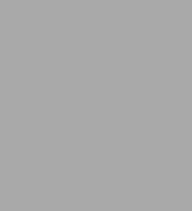
Building Automated Trading Systems: With an Introduction to Visual C++.NET 2005
336
by Benjamin Van Vliet
Benjamin Van Vliet
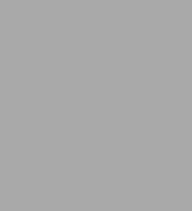
Building Automated Trading Systems: With an Introduction to Visual C++.NET 2005
336
by Benjamin Van Vliet
Benjamin Van Vliet
eBook
$72.99
$96.95
Save 25%
Current price is $72.99, Original price is $96.95. You Save 25%.
Available on Compatible NOOK devices, the free NOOK App and in My Digital Library.
WANT A NOOK?
Explore Now
Related collections and offers
72.99
In Stock
Overview
Over the next few years, the proprietary trading and hedge fund industries will migrate largely to automated trade selection and execution systems. Indeed, this is already happening. While several finance books provide C++ code for pricing derivatives and performing numerical calculations, none approaches the topic from a system design perspective. This book will be divided into two sections—programming techniques and automated trading system ( ATS ) technology—and teach financial system design and development from the absolute ground up using Microsoft Visual C++.NET 2005. MS Visual C++.NET 2005 has been chosen as the implementation language primarily because most trading firms and large banks have developed and continue to develop their proprietary algorithms in ISO C++ and Visual C++.NET provides the greatest flexibility for incorporating these legacy algorithms into working systems. Furthermore, the .NET Framework and development environment provide the best libraries and tools for rapid development of trading systems.
The first section of the book explains Visual C++.NET 2005 in detail and focuses on the required programming knowledge for automated trading system development, including object oriented design, delegates and events, enumerations, random number generation, timing and timer objects, and data management with STL.NET and .NET collections. Furthermore, since most legacy code and modeling code in the financial markets is done in ISO C++, this book looks in depth at several advanced topics relating to managed/unmanaged/COM memory management and interoperability. Further, this book provides dozens of examples illustrating the use of database connectivity with ADO.NET and an extensive treatment of SQL and FIX and XML/FIXML. Advanced programming topics such as threading, sockets, as well as using C++.NET to connect to Excel are also discussed at length and supported by examples.
The second section of the book explains technological concerns and design concepts for automated trading systems. Specifically, chapters are devoted to handling real-time data feeds, managing orders in the exchange order book, position selection, and risk management. A .dll is included in the book that will emulate connection to a widely used industry API ( Trading Technologies, Inc.’s XTAPI ) and provide ways to test position and order management algorithms. Design patterns are presented for market taking systems based upon technical analysis as well as for market making systems using intermarket spreads.
As all of the chapters revolve around computer programming for financial engineering and trading system development, this book will educate traders, financial engineers, quantitative analysts, students of quantitative finance and even experienced programmers on technological issues that revolve around development of financial applications in a Microsoft environment and the construction and implementation of real-time trading systems and tools.
* Teaches financial system design and development from the ground up using Microsoft Visual C++.NET 2005.
* Provides dozens of examples illustrating the programming approaches in the book
* Chapters are supported by screenshots, equations, sample Excel spreadsheets, and programming code.
The first section of the book explains Visual C++.NET 2005 in detail and focuses on the required programming knowledge for automated trading system development, including object oriented design, delegates and events, enumerations, random number generation, timing and timer objects, and data management with STL.NET and .NET collections. Furthermore, since most legacy code and modeling code in the financial markets is done in ISO C++, this book looks in depth at several advanced topics relating to managed/unmanaged/COM memory management and interoperability. Further, this book provides dozens of examples illustrating the use of database connectivity with ADO.NET and an extensive treatment of SQL and FIX and XML/FIXML. Advanced programming topics such as threading, sockets, as well as using C++.NET to connect to Excel are also discussed at length and supported by examples.
The second section of the book explains technological concerns and design concepts for automated trading systems. Specifically, chapters are devoted to handling real-time data feeds, managing orders in the exchange order book, position selection, and risk management. A .dll is included in the book that will emulate connection to a widely used industry API ( Trading Technologies, Inc.’s XTAPI ) and provide ways to test position and order management algorithms. Design patterns are presented for market taking systems based upon technical analysis as well as for market making systems using intermarket spreads.
As all of the chapters revolve around computer programming for financial engineering and trading system development, this book will educate traders, financial engineers, quantitative analysts, students of quantitative finance and even experienced programmers on technological issues that revolve around development of financial applications in a Microsoft environment and the construction and implementation of real-time trading systems and tools.
* Teaches financial system design and development from the ground up using Microsoft Visual C++.NET 2005.
* Provides dozens of examples illustrating the programming approaches in the book
* Chapters are supported by screenshots, equations, sample Excel spreadsheets, and programming code.
Product Details
ISBN-13: | 9780080476254 |
---|---|
Publisher: | Elsevier Science |
Publication date: | 03/07/2007 |
Series: | Financial Market Technology |
Sold by: | Barnes & Noble |
Format: | eBook |
Pages: | 336 |
File size: | 7 MB |
About the Author
Ben Van Vliet is a Lecturer at the Illinois Institute of Technology (IIT), where he also serves as the Associate Director of the M.S. Financial Markets program. At IIT he teaches courses in quantitative finance, C++ and .NET programming, and automated trading system design and development. He is vice chairman of the Institute for Market Technology, where he chairs the advisory board for the Certified Trading System Developer (CTSD) program. He also serves as series editor of the Financial Markets Technology series for Elsevier/Academic Press and consults extensively in the financial markets industry.
Mr. Van Vliet is also the author of "Modeling Financial Markets" with Robert Hendry (2003, McGraw Hill) and "Building Automated Trading Systems"(2007, Academic Press. Additionally, he has published several articles in the areas of finance and technology, and presented his research at several academic and professional conferences.
Table of Contents
Acknowledgments xiii
Introduction 1
ISO C++ 2
Structure of This Book 2
Introduction to Visual C++.NET 2005
The .NET Framework 5
MS Visual Studio 2005 Project Structure 5
What is C++/CLI? 5
Why Visual C++.NET? 6
The VC++.NET Compiler 7
What About Speed? 7
The .NET Framework 7
Sample Code: MessageBox_Example 9
Sample Code: StringConcat_Example 11
Sample Code: Debug_Example 12
Versioning 14
Summary 14
Tracking References 15
Sample Code: TrackingReference_Example 15
Sample Code: TemplateFunction_Example 16
^Managed Handle 16
Sample Code: RefType_Example 17
Summary 17
Classes and Objects 19
Abstraction 19
Encapsulation 21
Inheritance 21
Polymorphism 21
Memory Management in .NET 21
.NET Types 22
Unmanaged Types 23
Mixed Assemblies 23
Summary 23
Reference Types 25
Sample Code: RefType_Example 26
Delete and Dispose 27
Finalize 28
Sample Code: Finalize_Example 29
Stack Semantics for Ref Types 30
Nullptr Reference 30
This is Important 31
Summary 31
Value Types 33
Sample Code: ValueTypes_Example 34
Sample Code: PassingValueTypes_Example 35
Summary 36
Unmanaged Objects 37
Sample Code: UnmanagedObject_Example 37
Summary 39
Composition 41
Sample Code: Composition_Example 41
Sample Code: UnmanagedComposition_Example 44
Sample Code: ManagedComposition_Example 46
Summary 48
Properties 49
Sample Code: Properties_Example 49
Summary 50
Structures and Enumerations 51
Sample Code: ValueStructure_Example 51
Sample Code: ReferenceStructure_Example 52
Sample Code: Enums_Example 53
Summary 53
Inheritance 55
Access Modifiers 55
Object Class 56
Abstract and Sealed Classes 56
Sample Code: Inheritance_Example 56
Interfaces 58
Sample Code: Interface_Example 59
Runtime Callable Wrapper 60
Summary 60
Converting and Casting 61
Converting 61
Sample Code: Convert_Example 61
Static Casting 62
Sample Code: StaticCast_Example 62
Dynamic Casting 62
Sample Code: DynamicCast_Example 62
Safe Casting 64
Sample Code: SafeCast_Example 64
Summary 65
Operator Overloading 67
Sample Code: OpOverload_Example 67
Summary 69
Delegates and Events 71
Delegates 71
Sample Code: Delegates_Example 72
Multicasting 73
Sample Code: Multicast_Example 73
Events 75
Sample Code: Event_Example 76
Wrappers 78
Sample Code: Wrapper_Example 78
Asynchronous Method Calls 80
Sample Code: AsynchEvent_Example 80
Summary 82
Arrays 83
Sample Code: ManagedArray_Example 83
Sample Code: PassingArrays_Example 84
Summary 85
Generating Random Numbers 87
Sample Code: Random_Example 87
Sample Code: StdNormRandom_Example 87
Summary 89
Time and Timers 91
Sample Code: Milliseconds_Example 91
Stopwatch 92
Sample Code: Stopwatch_Example 93
Timers 93
Sample Code: FormsTimer_Example 93
Sample Code: ThreadingTimer_Example 95
Sample Code: TimersTimer_Example 96
Summary 98
Input and Output Streams 99
FileStream Class 99
StreamWriter Class 99
File and Directory Classes 99
Application Class 100
FileMode Enumeration 100
Sample Code: StreamWriter_Example 100
Sample Code: StreamReader_Example 101
Summary 101
Exception Handling 103
Sample Code: Exceptions_Example 103
Catching Unmanaged C++ Types 104
Summary 105
Collections 107
Sample Code: Hashtable_Example 107
Sorted List Class 109
Sample Code: SortedList_Example 109
Thread Safety 110
Generics 110
Sample Code: LinkedList_Example 110
Sample Code: Generics_Example 111
Summary 112
STL/STL.NET 113
Sample Code: STL.NET_Example 113
Sample Code: STL_Example 114
Summary 114
DataSets 115
Sample Code: DataSet_Example 116
Rows, DataRowCollections, and DataRows 116
Summary 117
Connecting to Databases 119
Database Connection 119
DataAdapter 120
Sample Code: ADO.NET_Example 120
Enumerating Through All the Data in a DataSet 122
Using Excel as a Data Source 123
Writing XML from a DataSet 123
Updating a Database with Changes in a Dataset 123
Retrieving Data with a DataReader 124
Summary 124
Structured Query Language 125
Data Manipulation Language 125
Updating a Database with Changes in a DataSet 138
Data Definition Language 138
Summary 140
XML 141
Well-Formed XML Documents 141
Valid XML Documents 141
XML Schema Documents 142
Parsers 142
Sample Code: Traders.xsd 142
Sample Code: XmlWriter_Example 144
Sample Code: XmlReader_Example 144
Summary 146
Financial Information Exchange Protocol 147
XML Protocols in Financial Markets 147
Overview of FIX 148
Summary 151
Serialization 153
Serialization_Example 153
Summary 154
Windows Services 155
Sample Code: WindowsService_Example 155
Summary 159
Setup and Installation Packages 161
Sample Code: Installation_Example 161
Summary 162
Concurrency
Threading 165
Threading Namespace 166
Sample Code: Thread_Example 166
Sample Code: ThreadAbort_Example 167
Thread Priority 169
Sample Code: ThreadPriority_Example 170
ThreadState Enumeration 170
ThreadPool Class 171
Sample Code: ThreadPool_Example 171
Updating Forms from Other Threads 172
Sample Code: FormUpdate_Example 172
Thread Safety 174
Summary 175
Synchronization Classes 177
Sample Code: Synchronize_Example 177
Mutex Class 178
Example Code: Mutex_Example 178
Semaphore Class 180
Sample Code: Spmaphore_Example 180
Monitor Class 182
Sample Code: Monitor 182
Summary 182
Sockets 183
Sample Code: SynchronousServer_Example 184
Sample Code: SynchronousClient_Example 187
Summary 189
Interoperability and Connectivity
Marshaling 193
Marshal Class 193
Sample Code: StringToCharArray_Example 194
Summary 194
Interior and Pinning Pointers 195
Sample Code: InteriorPointer_Example 195
Pinning Pointers 196
Sample Code: Pinning_Example 196
Summary 198
Connecting to Managed DLLs 199
Example Code: DLL_Example 199
Summary 201
Connecting to Component Object Model (COM) DLLs with COM Interop 203
Sample Code: MyCOMLibrary 203
Sample Code: UsingCOMDLL_Example 207
Summary 207
Connecting to C++ DLLs with Platform Invocation Services 209
Calling C-Style Functions 209
Sample Code: MyWin32Library 209
Sample Code: UsingWin32DLL_Example 211
Creating Objects 212
Sample Code: MyWin32ClassLibrary 212
Sample Code: UsingWin32Class_Example 214
CallingConventionEnumeration 215
Summary 216
Connecting to Excel 217
Sample Code: ControllingExcel_Example 217
Sample Code: ExcelChart_Example 220
Summary 221
Connecting to TraderAPI 223
TraderAPI Overview 223
FillObj 224
InstrObjClass 224
InstrNotifyClass 225
OrderObj 225
OrderProfileClass 225
OrderSetClass 226
Sample Code: TraderAPIConnection_Example 227
Summary 230
Connecting to XTAPI 231
Sample Code: XTAPIConnection_Example 231
Summary 233
Automated Trading Systems
Building Trading Systems 237
Buy vs. Build 237
Data Mapping 239
Speed of Development 240
Ten Things that Affect the Speed of a Trading System 241
Getting It Right 242
Logic Leaks 243
Ten Things that Affect the Profitability of a Trading System 244
Summary 245
K|V Trading System Development Methodology 247
The Money Document 249
Research and Document Calculations 249
Back Test 252
Implement 253
Manage Portfolio and Risk 255
Summary 257
Automated Trading System Classes 259
Instrument Class 259
Order Class 263
Order Book 264
Bracket 264
Tick 264
Tick or Bar Collection 264
Bar 264
System Manager 265
Graphical User Interface 265
Summary 265
Single-Threaded, Technical Analysis System 267
Sample Code: TechincalSystem_Example 268
Summary 277
Producer/Consumer Design Pattern 279
Sample Code: ProducerConsumer_Example 279
Summary 287
Multithreaded, Statistical Arbitrage System 289
Sample Code: Spreader_Example 291
Summary 304
Conclusion 304
What People are Saying About This
From the Publisher
Illustrates how to take quantitative finance methods and implement them in the real-world, real-time, electronic markets using Microsoft’s .NET platform
From the B&N Reads Blog
Page 1 of