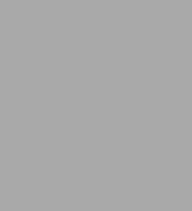
C# Essentials: Programming the .NET Framework
216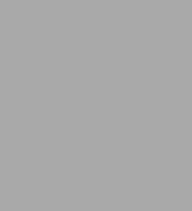
C# Essentials: Programming the .NET Framework
216Paperback(Second Edition)
-
PICK UP IN STORECheck Availability at Nearby Stores
Available within 2 business hours
Related collections and offers
Overview
- Every C# language element and its syntax, in reference format, including new keywords
- The major C# datatypes, with code examples
- Common C# programming tasks
- Interoperation with legacy Win32 APIs and COM components, and the use of C/C++ style pointers within the managed context of the CLR
- Common development issues
Product Details
ISBN-13: | 9780596003159 |
---|---|
Publisher: | O'Reilly Media, Incorporated |
Publication date: | 02/28/2002 |
Edition description: | Second Edition |
Pages: | 216 |
Product dimensions: | 6.00(w) x 9.00(h) x 0.53(d) |
About the Author
Peter Drayton is an independent consultant, helping early-stage companies define and build systems that take advantage of technologies such as .NET, SOAP, XML, and COM+. Peter is also an instructor for DevelopMentor, where he teaches Essential C#.NET. Originally from Cape Town, South Africa, Peter now lives in the San Francisco Bay Area with his wife, Julie. He spends his spare time researching .NET and tinkering with a small flotilla of computers cluttering up their a partment. He can be reached at peter@razorsoft.com.
Brad Merrill works as a software engineer in the .NET Framework Integration team at Microsoft. He previously worked as a software engineer at Digital Equipment Corporation and Sybase. His areas of expertise are in distributed systems, transaction processing, operating systems, and compiler technology. Brad lives in Redmond, Washington, and is an avid tournament chess player and bridge player. He can be reached at zbrad@gte.net or http://www.cybercom.net/~zbrad.
Read an Excerpt
Chapter 1: Introduction
C# is a language built specifically to program the new Microsoft .NET Framework. The .NET Framework consists of a runtime environment called the Common Language Runtime (CLR), and a set of base class libraries, which provide a rich development platform that can be exploited by a variety of languages and tools.
C# Language
Programming languages have strengths in different areas. Some languages are powerful but can be bug-prone and difficult to work with, while others are simpler but can be limiting in terms of functionality or performance. C# is a new language designed to provide an optimum blend of simplicity, expressiveness, and performance.
Many features of C# were designed in response to the strengths and weaknesses of other languages, particularly Java and C++. The C# language specification was written by Anders Hejlsberg and Scott Wiltamuth. Anders Hejlsberg is famous in the programming world for creating the Turbo Pascal compiler and leading the team that designed Delphi.
Key features of the C# language include the following:
Component orientation
An excellent way to manage complexity in a program is to subdivide it into several interacting components, some of which can be used in multiple scenarios. C# has been designed to make component building easy and provides component-oriented language constructs such as properties, events, and declarative constructs called attributes.One-stop coding
Everything pertaining to a declaration in C# is localized to the declaration itself, rather than being spread across several source files or several places within a source file. Types do not require additional declarations in separate header or Interface Definition Language (IDL) files, a property'sget/set
methods are logically grouped, documentation is embedded directly in a declaration, etc. Furthermore, because declaration order is irrelevant types don't require a separate stub declaration to be used by another type.Versioning
C# provides features such as explicit interface implementations, hiding inherited members, and read-only modifiers, which help new versions of a component work with older components that depend on it.Safe type system
C# is type-safe, which ensures that a variable can be accessed only through the type associated with that variable. This encapsulation encourages good programming design and eliminates potential bugs or security breaches by making it impossible for one variable to inadvertently or maliciously overwrite another.
All C# types (including primitive types) derive from a single base type, providing a unified type system. This means all types--structs, interfaces, delegates, enums, and arrays--share the same basic functionality, such as the ability to be converted to a string, serialized, or stored in a collection.Automatic memory management
C# relies on a runtime that performs automatic memory management. This frees programmers from disposing objects, which eliminates problems such as dangling pointers, memory leaks, and coping with circular references.
However, C# does not eliminate pointers: it merely makes them unnecessary for most programming tasks. For performance-critical hotspots and interoperability, pointers may be used, but they are only permitted inunsafe
blocks that require a high security permission to execute.Leveraging of the CLR
A big advantage of C# over other languages, particularly traditionally compiled languages such as C++, is its close fit with the .NET CLR. Many aspects of C# alias the CLR, especially its type system, memory-management model, and exception-handling mechanism.
Common Language Runtime
Of fundamental importance to the .NET framework is the fact that programs are executed within a managed execution environment provided by the Common Language Runtime. The CLR greatly improves runtime interactivity between programs, portability, security, development simplicity, cross-language integration, and provides an excellent foundation for a rich set of class libraries.
Absolutely key to these benefits is the way .NET programs are compiled. Each language targeting .NET compiles source code and produces metadata and Microsoft Intermediate Language (MSIL) code. Metadata includes a complete specification for a program including all its types, apart from the actual implementation of each function. These implementations are stored as MSIL, which is machine-independent code that describes the instructions of a program. The CLR uses this "blueprint" to bring a .NET program to life at runtime, providing services far beyond what is possible with the traditional approach--compiling code directly to assembly language.
Key features of the CLR include the following:
Runtime interactivity
Programs can richly interact with each other at runtime through their metadata. A program can search for new types at runtime, then instantiate and invoke methods on those types.Portability
Programs can be run without recompiling on any operating system and processor combination that supports the CLR. A key element of this platform independence is the runtime's JIT ( Just-In-Time Compiler), which compiles the MSIL code it is fed to native code that runs on the underlying platform.Security
Security considerations permeate the design of the .NET Framework. Key to making this possible is CLR's ability to analyze MSIL instructions as being safe or unsafe.Simplified deployment
An assembly is a completely self-describing package that contains all the metadata and MSIL of a program. Deployment can be as easy as copying the assembly to the client computer.Versioning
An assembly can function properly with new versions of assemblies it depends on without recompilation. Key to making this possible is the ability to resolve all type references though metadata.Simplified development
The CLR provides many features that greatly simplify development, including services such as garbage collection, exception handling, debugging, and profiling.Cross language integration
The Common Type System (CTS) of the CLR defines the types that can be expressed in metadata and MSIL and the possible operations that can be performed on those types. The CTS is broad enough to support many different languages, including Microsoft languages, such as C#, VB.NET, and VC.NET, and such third party languages as COBOL, Eiffel, Haskell, Mercury, ML, Oberon, Perl, Python, Smalltalk, and Scheme.
The Common Language Specification (CLS) defines a subset of the CTS, which provides a common standard that enables .NET languages to share and extend each other's libraries. For instance, an Eiffel programmer can create a class that derives from a C# class and override its virtual methods.Interoperability with legacy code
The CLR provides interoperation with the vast base of existing software written in COM and C. .NET types can be exposed as COM types, and COM types can be imported as .NET types. In addition, the CLR provides PInvoke, which is a mechanism that enables C functions, structs, and callbacks to be easily used from within in a .NET program.
Base Class Libraries
The .NET Framework provides the Base Class Libraries (BCL), which can be used by all languages. These libraries range from those that are a portal to core functionality of the runtime, such as threading and runtime manipulation of types (reflection), to libraries that provide high-level functionality, such as data access, rich client support, and web services (whereby code can even be embedded in a web page). C# has almost no built-in libraries; it uses the BCL instead.
A Minimal C# Program
A minimal C# program is implemented like this:
class Test {
static void Main( ) {
System.Console.WriteLine("Welcome to C#!");
}
}
All C# statements are scoped to methods (or special forms of methods), and all methods are scoped to types, such as the class Test
. The Main
method is recognized as the default entry point of execution, static
means the method is an ordinary procedure that does not require an instance of Test
to execute, and void
means the method does not return a value. System
is a namespace containing many related types, one of which is Console
. Console
is the class that encapsulates standard input/output functionality and has many methods including WriteLine
. The brackets, braces, and semicolons are necessary to group and state things unambiguously, and the indentation is there for readability.
To compile this program into an executable, paste it into a text file, save it as Test.cs, then type cs
Test.cs
in the command prompt. This compiles the program into an executable called Test.exe....
Table of Contents
- Preface
- Chapter 1: Introduction
- Chapter 2: C# Language Reference
- Chapter 3: Programming the.NET Framework
- Chapter 4: Framework Class Library Overview
- Chapter 5: Essential .NET Tools
- C# Keywords
- Regular Expressions
- Format Specifiers
- Data Marshaling
- Working with Assemblies
- Namespaces and Assemblies
- Colophon