C++ for Java Programmers is a concise, well-written text that provides authoritative and up-to-date coverage of key features and details of C++, with a special focus on how C++ compares to Java. The book's approach shows knowledgeable students or professionals how to grasp the complexities of C++ and harness its power by mutually addressing the benefits and the pitfalls of the two languages. By highlighting the features and comparative elements of each language, and building on the reader's existing knowledge of object-oriented programming, C++ for Java Programmers enables users to master the essentials of C++ quickly and thoroughly.
Key Features- Includes insightful comparisons of the two programming languages throughout the text and points out the subtleties of C++
- Succinctly covers the pertinent highlights of STL (Standard Template Library) and the most effective use of templates
- Explains the use of the powerful JNI (Java Native Interface) for combining Java and C++
- Includes a summary of key C++ features and issues with each chapter
- Provides extensive treatment of C details the programmer is likely to encounter in C++
- Companion Website for complete online source code at:
http://www.prenhall.com/weiss - Available Instructors Resource CD-ROM
C++ for Java Programmers is a concise, well-written text that provides authoritative and up-to-date coverage of key features and details of C++, with a special focus on how C++ compares to Java. The book's approach shows knowledgeable students or professionals how to grasp the complexities of C++ and harness its power by mutually addressing the benefits and the pitfalls of the two languages. By highlighting the features and comparative elements of each language, and building on the reader's existing knowledge of object-oriented programming, C++ for Java Programmers enables users to master the essentials of C++ quickly and thoroughly.
Key Features- Includes insightful comparisons of the two programming languages throughout the text and points out the subtleties of C++
- Succinctly covers the pertinent highlights of STL (Standard Template Library) and the most effective use of templates
- Explains the use of the powerful JNI (Java Native Interface) for combining Java and C++
- Includes a summary of key C++ features and issues with each chapter
- Provides extensive treatment of C details the programmer is likely to encounter in C++
- Companion Website for complete online source code at:
http://www.prenhall.com/weiss - Available Instructors Resource CD-ROM
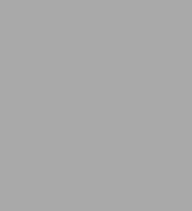
C++ for Java Programmers
304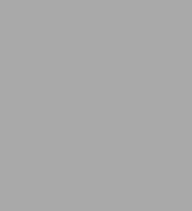
C++ for Java Programmers
304Paperback(New Edition)
Product Details
ISBN-13: | 9780139194245 |
---|---|
Publisher: | Pearson Education |
Publication date: | 10/28/2003 |
Edition description: | New Edition |
Pages: | 304 |
Product dimensions: | 6.90(w) x 9.20(h) x 0.80(d) |