Classic Computer Science Problems in Python
”Highly recommended to everyone interested in deepening their understanding of Python and practical computer science.” | Key Features > Master formal techniques taught in college computer science classes > Connect computer science theory to real-world applications, data, and performance > Prepare for programmer interviews > Recognize the core ideas behind most “new” challenges > Covers Python 3.7 Note: Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About The Book
Programming problems that seem new or unique are usually rooted in well-known engineering principles. Classic Computer Science Problems in Python guides you through time-tested scenarios, exercises, and algorithms that will prepare you for the “new” problems you’ll face when you start your next project.
In this amazing book, you'll tackle dozens of coding challenges, ranging from simple tasks like binary search algorithms to clustering data using k-means. As you work through examples for web development, machine learning, and more, you'll remember important things you've forgotten and discover classic solutions that will save you hours of time.
What You Will Learn
• Search algorithms
• Common techniques for graphs
• Neural networks
• Genetic algorithms
• Adversarial search
• Uses type hints throughout
This Book Is Written For
For intermediate Python programmers.
About The Author
David Kopec is an assistant professor of Computer Science and Innovation at Champlain College in Burlington, Vermont. He is the author of Dart for Absolute Beginners (Apress, 2014), Classic Computer Science Problems in Swift (Manning, 2018), and Classic Computer Science Problems in Java (Manning, 2020)
Table of Contents
1. Small problems
2. Search problems
3. Constraint-satisfaction problems
4. Graph problems
5. Genetic algorithms
6. K-means clustering
7. Fairly simple neural networks
8. Adversarial search
9. Miscellaneous problems
1129201775
About The Book
Programming problems that seem new or unique are usually rooted in well-known engineering principles. Classic Computer Science Problems in Python guides you through time-tested scenarios, exercises, and algorithms that will prepare you for the “new” problems you’ll face when you start your next project.
In this amazing book, you'll tackle dozens of coding challenges, ranging from simple tasks like binary search algorithms to clustering data using k-means. As you work through examples for web development, machine learning, and more, you'll remember important things you've forgotten and discover classic solutions that will save you hours of time.
What You Will Learn
• Search algorithms
• Common techniques for graphs
• Neural networks
• Genetic algorithms
• Adversarial search
• Uses type hints throughout
This Book Is Written For
For intermediate Python programmers.
About The Author
David Kopec is an assistant professor of Computer Science and Innovation at Champlain College in Burlington, Vermont. He is the author of Dart for Absolute Beginners (Apress, 2014), Classic Computer Science Problems in Swift (Manning, 2018), and Classic Computer Science Problems in Java (Manning, 2020)
Table of Contents
1. Small problems
2. Search problems
3. Constraint-satisfaction problems
4. Graph problems
5. Genetic algorithms
6. K-means clustering
7. Fairly simple neural networks
8. Adversarial search
9. Miscellaneous problems
Classic Computer Science Problems in Python
”Highly recommended to everyone interested in deepening their understanding of Python and practical computer science.” | Key Features > Master formal techniques taught in college computer science classes > Connect computer science theory to real-world applications, data, and performance > Prepare for programmer interviews > Recognize the core ideas behind most “new” challenges > Covers Python 3.7 Note: Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About The Book
Programming problems that seem new or unique are usually rooted in well-known engineering principles. Classic Computer Science Problems in Python guides you through time-tested scenarios, exercises, and algorithms that will prepare you for the “new” problems you’ll face when you start your next project.
In this amazing book, you'll tackle dozens of coding challenges, ranging from simple tasks like binary search algorithms to clustering data using k-means. As you work through examples for web development, machine learning, and more, you'll remember important things you've forgotten and discover classic solutions that will save you hours of time.
What You Will Learn
• Search algorithms
• Common techniques for graphs
• Neural networks
• Genetic algorithms
• Adversarial search
• Uses type hints throughout
This Book Is Written For
For intermediate Python programmers.
About The Author
David Kopec is an assistant professor of Computer Science and Innovation at Champlain College in Burlington, Vermont. He is the author of Dart for Absolute Beginners (Apress, 2014), Classic Computer Science Problems in Swift (Manning, 2018), and Classic Computer Science Problems in Java (Manning, 2020)
Table of Contents
1. Small problems
2. Search problems
3. Constraint-satisfaction problems
4. Graph problems
5. Genetic algorithms
6. K-means clustering
7. Fairly simple neural networks
8. Adversarial search
9. Miscellaneous problems
About The Book
Programming problems that seem new or unique are usually rooted in well-known engineering principles. Classic Computer Science Problems in Python guides you through time-tested scenarios, exercises, and algorithms that will prepare you for the “new” problems you’ll face when you start your next project.
In this amazing book, you'll tackle dozens of coding challenges, ranging from simple tasks like binary search algorithms to clustering data using k-means. As you work through examples for web development, machine learning, and more, you'll remember important things you've forgotten and discover classic solutions that will save you hours of time.
What You Will Learn
• Search algorithms
• Common techniques for graphs
• Neural networks
• Genetic algorithms
• Adversarial search
• Uses type hints throughout
This Book Is Written For
For intermediate Python programmers.
About The Author
David Kopec is an assistant professor of Computer Science and Innovation at Champlain College in Burlington, Vermont. He is the author of Dart for Absolute Beginners (Apress, 2014), Classic Computer Science Problems in Swift (Manning, 2018), and Classic Computer Science Problems in Java (Manning, 2020)
Table of Contents
1. Small problems
2. Search problems
3. Constraint-satisfaction problems
4. Graph problems
5. Genetic algorithms
6. K-means clustering
7. Fairly simple neural networks
8. Adversarial search
9. Miscellaneous problems
39.99
In Stock
5
1
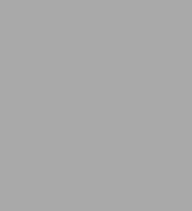
Classic Computer Science Problems in Python
224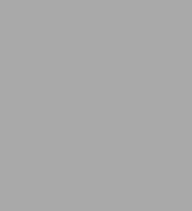
Classic Computer Science Problems in Python
224Paperback(1st Edition)
$39.99
39.99
In Stock
From the B&N Reads Blog