CodeNotes for ASP.NET
CodeNotes provides the most succinct, accurate, and speedy way for
a developer to ramp up on a new technology or language.
Unlike other programming books, CodeNotes drills down to
the core aspects of a technology, focusing on the key elements needed in order to implement it immediately. It is a unique resource for
developers, filling the gap between comprehensive manuals and
pocket references.
CodeNotes for ASP.NET is a revolutionary update to Microsoft’s Active Server Pages. This edition explores how Web applications and Web services can be developed using ASP.NET. The .NET architecture, Base Class Libraries, ASP.NET form designer,
Web controls, and cross-page and cross-language debugging
are discussed. Scaling ASP.NET to multiple servers,
state management, security, and methods of enhancing performance are also covered.
This edition of CodeNotes includes:
• Real-world examples
• “How and Why” and “Bugs and Caveats” sections that provide
workarounds and tips on what should be taken advantage
of or avoided
• “Design Notes” illustrating many of the common use patterns for
Java programs
• Instructions and classroom-style tutorials throughout from experts
Visit www.codenotes.com for updates, source code templates, access to
message boards, and discussion of specific problems with
CodeNotes authors and other developers.
Join our nonfiction newsletter by sending a blank e-mail to:
join-rht-nonfiction@list.randomhouse.com or visit www.atrandom.com
Every CodeNotes title is written and reviewed by a team of commercial software developers and technology experts. See “About the Authors” at the front of the book for more information.
1100300461
a developer to ramp up on a new technology or language.
Unlike other programming books, CodeNotes drills down to
the core aspects of a technology, focusing on the key elements needed in order to implement it immediately. It is a unique resource for
developers, filling the gap between comprehensive manuals and
pocket references.
CodeNotes for ASP.NET is a revolutionary update to Microsoft’s Active Server Pages. This edition explores how Web applications and Web services can be developed using ASP.NET. The .NET architecture, Base Class Libraries, ASP.NET form designer,
Web controls, and cross-page and cross-language debugging
are discussed. Scaling ASP.NET to multiple servers,
state management, security, and methods of enhancing performance are also covered.
This edition of CodeNotes includes:
• Real-world examples
• “How and Why” and “Bugs and Caveats” sections that provide
workarounds and tips on what should be taken advantage
of or avoided
• “Design Notes” illustrating many of the common use patterns for
Java programs
• Instructions and classroom-style tutorials throughout from experts
Visit www.codenotes.com for updates, source code templates, access to
message boards, and discussion of specific problems with
CodeNotes authors and other developers.
Join our nonfiction newsletter by sending a blank e-mail to:
join-rht-nonfiction@list.randomhouse.com or visit www.atrandom.com
Every CodeNotes title is written and reviewed by a team of commercial software developers and technology experts. See “About the Authors” at the front of the book for more information.
CodeNotes for ASP.NET
CodeNotes provides the most succinct, accurate, and speedy way for
a developer to ramp up on a new technology or language.
Unlike other programming books, CodeNotes drills down to
the core aspects of a technology, focusing on the key elements needed in order to implement it immediately. It is a unique resource for
developers, filling the gap between comprehensive manuals and
pocket references.
CodeNotes for ASP.NET is a revolutionary update to Microsoft’s Active Server Pages. This edition explores how Web applications and Web services can be developed using ASP.NET. The .NET architecture, Base Class Libraries, ASP.NET form designer,
Web controls, and cross-page and cross-language debugging
are discussed. Scaling ASP.NET to multiple servers,
state management, security, and methods of enhancing performance are also covered.
This edition of CodeNotes includes:
• Real-world examples
• “How and Why” and “Bugs and Caveats” sections that provide
workarounds and tips on what should be taken advantage
of or avoided
• “Design Notes” illustrating many of the common use patterns for
Java programs
• Instructions and classroom-style tutorials throughout from experts
Visit www.codenotes.com for updates, source code templates, access to
message boards, and discussion of specific problems with
CodeNotes authors and other developers.
Join our nonfiction newsletter by sending a blank e-mail to:
join-rht-nonfiction@list.randomhouse.com or visit www.atrandom.com
Every CodeNotes title is written and reviewed by a team of commercial software developers and technology experts. See “About the Authors” at the front of the book for more information.
a developer to ramp up on a new technology or language.
Unlike other programming books, CodeNotes drills down to
the core aspects of a technology, focusing on the key elements needed in order to implement it immediately. It is a unique resource for
developers, filling the gap between comprehensive manuals and
pocket references.
CodeNotes for ASP.NET is a revolutionary update to Microsoft’s Active Server Pages. This edition explores how Web applications and Web services can be developed using ASP.NET. The .NET architecture, Base Class Libraries, ASP.NET form designer,
Web controls, and cross-page and cross-language debugging
are discussed. Scaling ASP.NET to multiple servers,
state management, security, and methods of enhancing performance are also covered.
This edition of CodeNotes includes:
• Real-world examples
• “How and Why” and “Bugs and Caveats” sections that provide
workarounds and tips on what should be taken advantage
of or avoided
• “Design Notes” illustrating many of the common use patterns for
Java programs
• Instructions and classroom-style tutorials throughout from experts
Visit www.codenotes.com for updates, source code templates, access to
message boards, and discussion of specific problems with
CodeNotes authors and other developers.
Join our nonfiction newsletter by sending a blank e-mail to:
join-rht-nonfiction@list.randomhouse.com or visit www.atrandom.com
Every CodeNotes title is written and reviewed by a team of commercial software developers and technology experts. See “About the Authors” at the front of the book for more information.
14.99
In Stock
5
1
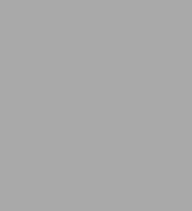
CodeNotes for ASP.NET
272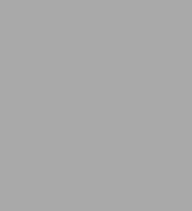
CodeNotes for ASP.NET
272
14.99
In Stock
Product Details
ISBN-13: | 9780679647447 |
---|---|
Publisher: | Random House Publishing Group |
Publication date: | 08/13/2002 |
Series: | Codenotes Series |
Sold by: | Random House |
Format: | eBook |
Pages: | 272 |
File size: | 8 MB |
About the Author
From the B&N Reads Blog