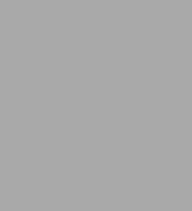
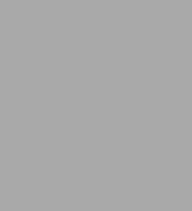
Paperback(13th ed.)
-
SHIP THIS ITEMAvailable for Pre-Order. This item will be released on August 31, 2024PICK UP IN STORE
Store Pickup available after publication date.
Available within 2 business hours
Related collections and offers
Overview
Whatever version of Java you are using, Core Java, Volume I: Fundamentals, Thirteenth Edition, will help you achieve a deep and practical understanding of the language and APIs. With hundreds of realistic examples, Java Champion Cay S. Horstmann demonstrates the most powerful and effective ways to get the job done.
Written for experienced programmers looking for in-depth coverage of the Java language and platform, this revised and updated edition continues to be the first choice for serious programmers. The carefully crafted sample programs demonstrate almost every language and library feature, as well as the newest capabilities introduced in Java 21. The examples are purposefully simple to focus on the major points, but, for the most part, they aren't fake and they don't cut corners. They should make good starting points for your own code.
This first of two volumes offers a detailed treatment of fundamental Java programming topics, including object-oriented programming, reflection and proxies, interfaces and inner classes, exception handling, generics, collections, lambda expressions, concurrency, annotations, and the Java platform module system.
- Master foundational techniques, idioms, and best practices for writing superior Java code
- Leverage the power of interfaces, lambda expressions, and inner classes
- Take advantage of sealed class hierarchies and pattern matching for processing structured data
- Harden programs through effective exception handling and debugging
- Write safer, more reusable code with generic programming
- Improve performance and efficiency with Java's standard collections
- Explore simple programs with JShell and assemble complex programs with archives and modules
- Fully utilize multicore processors with Java's powerful concurrency model
See Core Java, Volume II: Advanced Features, Thirteenth Edition, for coverage of Java 21 enterprise features, including detailed discussions of networking, security, internationalization, and advanced UI programming.
Product Details
ISBN-13: | 9780135328378 |
---|---|
Publisher: | Oracle Press |
Publication date: | 08/31/2024 |
Series: | Oracle Press for Java |
Edition description: | 13th ed. |
Pages: | 840 |
Product dimensions: | 6.00(w) x 1.25(h) x 9.00(d) |
About the Author
Table of Contents
Preface xiiiAcknowledgments xix
Chapter 1: An Introduction to Java 1
1.1 Java as a Programming Platform 1
1.2 The Java "White Paper" Buzzwords 2
1.3 Java Applets and the Internet 7
1.4 A Short History of Java 8
1.5 Common Misconceptions about Java 12
Chapter 2: The Java Programming Environment 15
2.1 Installing the Java Development Kit 15
2.2 Using the Command-Line Tools 19
2.3 Using an Integrated Development Environment 24
2.4 JShell 25
Chapter 3: Fundamental Programming Structures in Java 31
3.1 A Simple Java Program 31
3.2 Comments 35
3.3 Data Types 36
3.4 Variables and Constants 43
3.5 Operators 48
3.6 Strings 59
3.7 Input and Output 76
3.8 Control Flow 85
3.9 Big Numbers 107
3.10 Arrays 110
Chapter 4: Objects and Classes 127
4.1 Introduction to Object-Oriented Programming 127
4.2 Using Predefined Classes 132
4.3 Defining Your Own Classes 142
4.4 Static Fields and Methods 157
4.5 Method Parameters 164
4.6 Object Construction 171
4.7 Records 182
4.8 Packages 188
4.9 JAR Files 200
4.10 Documentation Comments 206
4.11 Class Design Hints 214
Chapter 5: Inheritance 217
5.1 Classes, Superclasses, and Subclasses 217
5.2 Object: The Cosmic Superclass 240
5.3 Generic Array Lists 257
5.4 Object Wrappers and Autoboxing 265
5.5 Methods with a Variable Number of Arguments 270
5.6 Abstract Classes 271
5.7 Enumeration Classes 277
5.8 Sealed Classes 282
5.9 Pattern Matching 288
5.10 Reflection 296
5.11 Design Hints for Inheritance 324
Chapter 6: Interfaces, Lambda Expressions, and Inner Classes 327
6.1 Interfaces 327
6.2 Lambda Expressions 354
6.3 Inner Classes 375
6.4 Service Loaders 393
6.5 Proxies 395
Chapter 7: Exceptions, Assertions, and Logging 403
7.1 Dealing with Errors 403
7.2 Catching Exceptions 412
7.3 Tips for Using Exceptions 427
7.4 Using Assertions 431
7.5 Logging 436
7.6 Debugging Tips 452
Chapter 8: Generic Programming 459
8.1 Why Generic Programming? 459
8.2 Defining a Simple Generic Class 462
8.3 Generic Methods 464
8.4 Bounds for Type Variables 465
8.5 Generic Code and the Virtual Machine 468
8.6 Inheritance Rules for Generic Types 474
8.7 Wildcard Types 477
8.8 Restrictions and Limitations 485
8.9 Reflection and Generics 498
Chapter 9: Collections 511
9.1 The Java Collections Framework 511
9.2 Interfaces in the Collections Framework 521
9.3 Concrete Collections 525
9.4 Maps 548
9.5 Copies and Views 562
9.6 Algorithms 574
9.7 Legacy Collections 586
Chapter 10: Concurrency 599
10.1 Running Threads 599
10.2 Thread States 605
10.3 Thread Properties 608
10.4 Coordinating Tasks 618
10.5 Synchronization 635
10.6 Thread-Safe Collections 667
10.7 Asynchronous Computations 685
10.8 Processes 702
Chapter 11: Annotations 711
11.1 Using Annotations 711
11.2 Defining Annotations 717
11.3 Annotations in the Java API 720
11.4 Processing Annotations at Runtime 725
11.5 Source-Level Annotation Processing 729
11.6 Bytecode Engineering 736
Chapter 12: The Java Platform Module System 747
12.1 The Module Concept 747
12.2 Naming Modules 748
12.3 The Modular "Hello, World!" Program 749
12.4 Requiring Modules 751
12.5 Exporting Packages 753
12.6 Modular JARs 757
12.7 Modules and Reflective Access 759
12.8 Automatic Modules 762
12.9 The Unnamed Module 764
12.10 Command-Line Flags for Migration 765
12.11 Transitive and Static Requirements 766
12.12 Qualified Exporting and Opening 768
12.13 Service Loading 769
12.14 Tools for Working with Modules 772
Appendix 775
Index 781