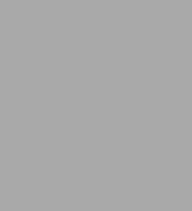
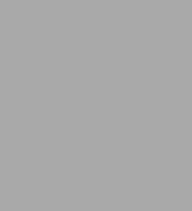
Paperback(13th ed.)
-
SHIP THIS ITEMAvailable for Pre-Order. This item will be released on August 31, 2024PICK UP IN STORE
Store Pickup available after publication date.
Available within 2 business hours
Related collections and offers
Overview
Core Java is the leading no-nonsense tutorial and reference for experienced programmers who want to write robust Java code for real-world applications. Now, Core Java, Volume II: Advanced Features, Thirteenth Edition, has been revised to cover the new features and enhancements in the Java 21 long-term support release. As always, all chapters have been completely updated, outdated material has been removed, and the new APIs are covered in detail.
This volume focuses on the advanced topics that a programmer needs to know for professional software development and includes authoritative coverage of enterprise programming, networking, databases, security, modularization, internationalization, code processing, and native methods, as well as complete chapters on the Streams, XML, and Date and Time APIs. In addition, the chapters on Swing and Graphics cover techniques that are applicable to both client-side user interfaces and server-side generation of graphics and images.
Cay S. Horstmann clearly explains sophisticated new features with depth and completeness and demonstrates how to use them to build professional-quality applications. Horstmann's thoroughly tested sample code reflects modern Java style and best practices. The examples are carefully crafted for easy understanding and maximum practical value, so you can rely on them to jump-start your own programs.
- Master advanced techniques, idioms, and best practices for writing reliable Java code
- Make the most of enhanced Java I/O APIs, object serialization, and regular expressions
- Connect to network services, harvest web data with the HTTP/2 client, and serve data with the built-in web server or implement your own server
- Process code via the Scripting and Compiler APIs
- Deepen your understanding of the Java Platform Module System, including recent refinements
- Leverage the Java security model, user authentication, and the security librarys cryptographic functions
- Preview powerful new APIs for accessing "foreign" functions and memory
"This is the definitive reference and instructional work for Java and the Java ecosystem."
Andrew Binstock, Java Magazine
See Core Java, Volume I: Fundamentals, Thirteenth Edition, for expert coverage of Java programming fundamentals, including objects, generics, collections, lambda expressions, concurrency, and functional programming.
Product Details
ISBN-13: | 9780135371749 |
---|---|
Publisher: | Pearson Education |
Publication date: | 08/31/2024 |
Series: | Oracle Press for Java |
Edition description: | 13th ed. |
Pages: | 988 |
Product dimensions: | 6.00(w) x 1.25(h) x 9.00(d) |
About the Author
Table of Contents
Preface xiAcknowledgments xv
Chapter 1: Streams 1
1.1 From Iterating to Stream Operations 1
1.2 Stream Creation 4
1.3 The filter, map, and flatMap Methods 10
1.4 Extracting Substreams and Combining Streams 13
1.5 Other Stream Transformations 14
1.6 Simple Reductions 15
1.7 The Optional Type 17
1.8 Collecting Results 25
1.9 Collecting into Maps 29
1.10 Grouping and Partitioning 33
1.11 Downstream Collectors 34
1.12 Reduction Operations 40
1.13 Primitive Type Streams 42
1.14 Parallel Streams 47
Chapter 2: Input and Output 53
2.1 Input/Output Streams 53
2.2 Reading and Writing Binary Data 77
2.3 Object Input/Output Streams and Serialization 87
2.4 Working with Files 113
2.5 Memory-Mapped Files 129
2.6 File Locking 139
2.7 Regular Expressions 144
Chapter 3: XML 161
3.1 Introducing XML 161
3.2 The Structure of an XML Document 163
3.3 Parsing an XML Document 167
3.4 Validating XML Documents 176
3.5 Locating Information with XPath 195
3.6 Using Namespaces 200
3.7 Streaming Parsers 203
3.8 Generating XML Documents 212
3.9 XSL Transformations 223
Chapter 4: Networking 235
4.1 Connecting to a Server 235
4.2 Implementing Servers 243
4.3 Getting Web Data 259
4.4 The HTTP Client 278
4.5 The Simple HTTP Server 287
4.6 Sending E-Mail 292
Chapter 5: Database Programming 297
5.1 The Design of JDBC 297
5.2 The Structured Query Language 300
5.3 JDBC Configuration 306
5.4 Working with JDBC Statements 311
5.5 Query Execution 322
5.6 Scrollable and Updatable Result Sets 334
5.7 Row Sets 340
5.8 Metadata 344
5.9 Transactions 353
5.10 Connection Management in Web and Enterprise Applications 358
Chapter 6: The Date and Time API 361
6.1 The Time Line 361
6.2 Local Dates 365
6.3 Date Adjusters 370
6.4 Local Time 372
6.5 Zoned Time 373
6.6 Formatting and Parsing 377
6.7 Interoperating with Legacy Code 383
Chapter 7: Internationalization 385
7.1 Locales 385
7.2 Number Formats 392
7.3 Date and Time 401
7.4 Collation and Normalization 405
7.5 Message Formatting 410
7.6 Text Boundaries 415
7.7 Text Input and Output 416
7.8 Resource Bundles 419
7.9 A Complete Example 424
Chapter 8: Compiling and Scripting 429
8.1 The Compiler API 429
8.2 Scripting for the Java Platform 438
Chapter 9: Security 449
9.1 Class Loaders 449
9.2 User Authentication 464
9.3 Digital Signatures 475
9.4 Encryption 492
Chapter 10: Graphical User Interface Programming 505
10.1 A History of Java User Interface Toolkits 505
10.2 Displaying Frames 506
10.3 Displaying Information in a Component 513
10.4 Event Handling 537
10.5 The Preferences API 562
Chapter 11: User Interface Components with Swing 569
11.1 Swing and the Model-View-Controller Design Pattern 569
11.2 Introduction to Layout Management 574
11.3 Text Input 580
11.4 Choice Components 588
11.5 Menus 607
11.6 The Grid Bag Layout 625
11.7 Custom Layout Managers 635
11.8 Dialog Boxes 640
Chapter 12: Advanced Swing and Graphics 665
12.1 Tables 665
12.2 Working with Rows and Columns 673
12.3 Cell Rendering and Editing 690
12.4 Trees 702
12.5 Advanced AWT 742
12.6 Raster Images 791
12.7 Printing 818
Chapter 13: Native Methods 853
13.1 Calling a C Function from a Java Program 854
13.2 Numeric Parameters and Return Values 860
13.3 String Parameters 862
13.4 Accessing Fields 868
13.5 Encoding Signatures 873
13.6 Calling Java Methods 875
13.7 Accessing Array Elements 882
13.8 Handling Errors 886
13.9 Using the Invocation API 891
13.10 A Complete Example: Accessing the Windows Registry 896
13.11 Foreign Functions: A Glimpse into the Future 911
Index 915