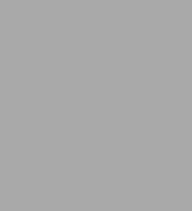
Dependency Injection Principles, Practices, and Patterns
552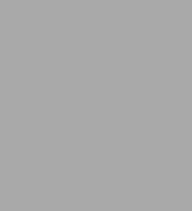
Dependency Injection Principles, Practices, and Patterns
552eBook
Available on Compatible NOOK devices, the free NOOK App and in My Digital Library.
Related collections and offers
Overview
Dependency Injection Principles, Practices, and Patterns teaches you to use DI to reduce hard-coded dependencies between application components. You'll start by learning what DI is and what types of applications will benefit from it. Then, you'll work through concrete scenarios using C# and the .NET framework to implement DI in your own projects. As you dive into the thoroughly-explained examples, you'll develop a foundation you can apply to any of the many DI libraries for .NET and .NET Core.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the Technology
Dependency Injection (DI) is a great way to reduce tight coupling between software components. Instead of hard-coding dependencies, such as specifying a database driver, you make those connections through a third party. Central to application frameworks like ASP.NET Core, DI enables you to better manage changes and other complexity in your software.
About the Book
Dependency Injection Principles, Practices, and Patterns is a revised and expanded edition of the bestselling classic Dependency Injection in .NET. It teaches you DI from the ground up, featuring relevant examples, patterns, and anti-patterns for creating loosely coupled, well-structured applications. The well-annotated code and diagrams use C# examples to illustrate principles that work flawlessly with modern object-oriented languages and DI libraries.
What's Inside
- Refactoring existing code into loosely coupled code
- DI techniques that work with statically typed OO languages
- Integration with common .NET frameworks
- Updated examples illustrating DI in .NET Core
About the Reader
For intermediate OO developers.
About the Authors
Mark Seemann is a programmer, software architect, and speaker who has been working with software since 1995, including six years with Microsoft. Steven van Deursen is a seasoned .NET developer and architect, and the author and maintainer of the Simple Injector DI library.
Table of Contents
- PART 1 Putting Dependency Injection on the map
- The basics of Dependency Injection: What, why, and how
- Writing tightly coupled code
- Writing loosely coupled code PART 2 Catalog
- DI patterns
- DI anti-patterns
- Code smells PART 3 Pure DI
- Application composition
- Object lifetime
- Interception
- Aspect-Oriented Programming by design
- Tool-based Aspect-Oriented Programming PART 4 DI Containers
- DI Container introduction
- The Autofac DI Container
- The Simple Injector DI Container
- The Microsoft.Extensions.DependencyInjection DI Container
Product Details
ISBN-13: | 9781638357100 |
---|---|
Publisher: | Manning |
Publication date: | 03/06/2019 |
Sold by: | SIMON & SCHUSTER |
Format: | eBook |
Pages: | 552 |
Sales rank: | 317,705 |
File size: | 15 MB |
Note: | This product may take a few minutes to download. |
About the Author
Steven van Deursen is a seasoned .NET developer and architect, and the author and maintainer of the Simple Injector DI library.
Table of Contents
Preface xv
Acknowledgments xvii
About this book xix
About the authors xxiii
About the cover illustration xxiv
Part 1 Putting- Dependency Injection on the map 1
1 The basics of Dependency Injection: What, why, and how 3
1.1 Writing maintainable code 5
Common myths about DI 5
Understanding the purpose of DI 8
1.2 A simple example: Hello DI! 14
Hello DI code 15
Benefits of DI 17
1.3 What to inject and what not to inject 24
Stable Dependencies 26
Volatile Dependencies 26
1.4 DI scope 27
Object Composition 29
Object Lifetime 30
Interception 30
DI in three dimensions 31
1.5 Conclusion 32
2 Writing tightly coupled code 34
2.1 Building a tightly coupled application 35
Meet Mary Rowan 35
Creating the data layer 36
Creating the domain layer 39
Creating the UI layer 42
2.2 Evaluating the tightly coupled application 44
Evaluating the dependency graph 44
Evaluating composability 45
2.3 Analysis of missing composability 47
Dependency graph analysis 47
Data access interface analysis 48
Miscellaneous other issues 50
2.4 Conclusion 50
3 Writing loosely coupled code 52
3.1 Rebuilding the e-commerce application 53
Building a more maintainable UI 56
Building an independent domain model 61
Building a new data access layer 70
Implementing an ASP.NET Core-specific IUserContext Adapter 71
Composing the application in the Composition Root 73
3.2 Analyzing the loosely coupled implementation 74
Understanding the interaction between components 74
Analyzing the new dependency graph 75
Part 2 Catalog 81
4 DI pattems 83
4.1 Composition Root 85
How Composition Root works 87
Using a DI Container in a Composition Root 88
Example: Implementing a Composition Root using Pure DI 89
The apparent dependency explosion 92
4.2 Constructor Injection 95
How Constructor Injection works 95
When to use Constructor Injection 97
Known use of Constructor Injection 99
Example: Adding currency conversions to the featured products 100
Wrap-up 102
4.3 Method Injection 104
How Method Injection works 104
When to use Method Injection 105
Known use of Method Injection 111
Example: Adding currency conversions to the Product Entity 112
4.4 Property Injection 114
How Property Injection works 114
When louse Property Injection 115
Known uses of Property Injection 118
Example: Property Injection as an extensibility model of a reusable library 118
4.5 Choosing which pattern to use 120
5 D1 anti-patterns 124
5.1 Control Freak 127
Example: Control Freak through newing up Dependencies 128
Example: Control Freak through factories 129
Example: Control Freak through overloaded constructors 134
Analysis of Control Freak 135
5.2 Service Locator 138
Example: ProductService using a Service Locator 140
Analysis of Service Locator 142
5.3 Ambient Context 146
Example: Accessing time through Ambient Context 147
Example: Logging through Ambient Context 149
Analysis of Ambient Context 150
5.4 Constrained Construction 154
Example: Late binding a ProductReposilory 154
Analysis of Constrained Construction 156
6 Code smells 163
6.1 Dealing with the Constructor Over-injection code smell 164
Recognizing Constructor Over-injection 165
Refactoring from Constructor Over-injection to Facade Services 168
Refactoring from Constructor Over-injection to domain events 173
6.2 Abuse of Abstract Factories 180
Abusing Abstract Factories to overcome lifetime problems 180
Abusing Abstract Factories to select Dependencies based on runtime data 187
6.3 Fixing cyclic Dependencies 194
Example: Dependency cycle caused by an SRP violation 195
Analysis of Mary's Dependency cycle 199
Refactoring from SRP violations to resolve the Dependency cycle 200
Common strategies for breaking Dependency cycles 204
Last resort: Breaking the cycle with Property Injection 204
Part 3 Pure DI 209
7 Application composition 211
7.1 Composing console applications 213
Example: Updating currencies using the UpdateCurrency program 214
Building the Composition Root of the UpdateCurrency program 215
Composing object graphs in Create Currency Parser 216
A closer look at UpdateCurrency's layering 217
7.2 Composing UWP applications 218
UWP composition 218
Example: Wiring up a product-management rich client 219
Implementing the Composition Root in the UWP application 226
7.3 Composing ASP.NET Core MVC applications 228
Creating a custom controller activator 230
Constructing custom middleware components using Pure DI 233
8 Object lifetime 236
8.1 Managing Dependency Lifetime 238
Introducing Lifetime Management 238
Managing lifetime with Pure DI 242
8.2 Working with disposable Dependencies 245
Consuming disposable Dependencies 246
Managing disposable Dependencies 250
8.3 Lifestyle catalog 255
The Singleton Lifestyle 256
The Transient Lifestyle 259
The Scoped Lifestyle 260
8.4 Bad Lifestyle choices 266
Captive Dependencies 266
Using Leaky Abstractions to leak Lifestyle choices to consumers 269
Causing concurrency bugs by tying instances to the lifetime of a thread 275
9 Interception 281
9.1 Introducing Interception 283
Decorator design pattern 284
Example: Implementing auditing using a Decorator 287
9.2 Implementing Cross-Cutting Concerns 290
Intercepting with a Circuit Breaker 292
Reporting exceptions using the Decorator pattern 297
Preventing unauthorized access to sensitive functionality using a Decorator 298
10 Aspect-Oriented Programming by design 301
10.1 Introducing AOP 302
10.2 The Solid principles 305
Single Responsibility Principle (SRP) 306
Open/Closed Principle (OCP) 306
Liskov Substitution Principle (LSP) 307
Interface Segregation Principle (ISP) 307
Dependency Inversion Principle (DIP) 308
Solid principles and Interception 308
10.3 Solid as a driver for AOP 308
Example: Implementing product-related features using IProductService 309
Analysis of IProductService from the perspective of Solid 311
Improving design, by applying Solid principles 314
Adding more Cross-Cutting Concerns 327
Conclusion 336
11 Tool-based Aspect-Oriented Programming 341
11.1 Dynamic Interception 342
Example: Interception with Castle Dynamic Proxy 344
Analysis of dynamic Interception 346
11.2 Compile-time weaving 348
Example: Applying a transaction aspect using compile-time weaving 349
Analysis of compile-time weaving 351
Part 4 DI Containers 357
12 D1 Container introduction 359
12.1 Introducing DI Containers 361
Exploring containers' Resolve API 361
Auto-Wiring 363
Example: Implementing a simplistic DI Container that supports Auto-Wiring 364
12.2 Configuring DI Containers 372
Configuring containers with configuration files 373
Configuring container's using Configuration As Code 377
Configuring containers by convention using Auto-Registration 379
Mixing and matching configuration approaches 385
12.3 When to use a DI Container 385
Using third,-party libraries involves costs and risks 386
Pure DI gives a shorter feedback cycle 388
The verdict: When to use a DI Container 389
13 The Autofac DI Container 393
13.1 Introducing Autofac 394
Resolving objects 395
Configuring the ContainerBuilder 398
13.2 Managing lifetime 404
Configuring instance scopes 405
Releasing components 406
13.3 Registering difficult APIs 409
Configuring primitive Dependencies 409
Registering objects with code blocks 411
13.4 Working with multiple components 412
Selecting among multiple candidates 413
Wiring sequences 417
Wiring Decorators 420
Wiring Composites 422
14 The Simple Injector DI Container 427
14.1 Introducing Simple Injector 428
Resolving objects 429
Configuring the container 432
14.2 Managing lifetime 438
Configuring Lifestyles 439
Releasing components 440
Ambient scopes 443
Diagnosing the container for common lifetime problems 444
14.3 Registering difficult APIs 447
Configuring primitive Dependencies 448
Extracting primitive Dependencies to Parameter Objects 449
Registering objects with code blocks 450
14.4 Working with multiple components 451
Selecting among multiple candidates 452
Wiring sequences 454
Wiring Decorators 457
Wiring Composites 459
Sequences are streams 462
15 The Microsoft.Extensions.DependencyInjection DI Container 466
15.1 Introducing MicrosoftExtensions.Dependencylnjection 467
Resolving objects 468
Configuring the ServiceCollection 471
15.2 Managing lifetime 476
Configuring Lifestyles 477
Releasing components 477
15.3 Registering difficult APIs 480
Configuring primitive Dependencies 480
Extracting primitive Dependencies to Parameter Objects 481
Registering objects with code blocks 482
15.4 Working with multiple components 483
Selecting among multiple candidates 483
Wiring sequences 486
Wiring Decorators 489
Wiring Composites 492
Glossary 499
Resources 504
Index 507