Effective C: An Introduction to Professional C Programming
A detailed introduction to the C programming language for experienced programmers.
The world runs on code written in the C programming language, yet most schools begin the curriculum with Python or Java. Effective C bridges this gap and brings C into the modern eracovering the modern C17 Standard as well as potential C2x features. With the aid of this instant classic, you'll soon be writing professional, portable, and secure C programs to power robust systems and solve real-world problems.
Robert C. Seacord introduces C and the C Standard Library while addressing best practices, common errors, and open debates in the C community. Developed together with other C Standards committee experts, Effective C will teach you how to debug, test, and analyze C programs. You'll benefit from Seacord's concise explanations of C language constructs and behaviors, and from his 40 years of coding experience.
You'll learn:How to identify and handle undefined behavior in a C program The range and representations of integers and floating-point values How dynamic memory allocation works and how to use nonstandard functions How to use character encodings and types How to perform I/O with terminals and filesystems using C Standard streams and POSIX file descriptors How to understand the C compiler's translation phases and the role of the preprocessor How to test, debug, and analyze C programs
Effective C will teach you how to write professional, secure, and portable C code that will stand the test of time and help strengthen the foundation of the computing world.
1136765031
The world runs on code written in the C programming language, yet most schools begin the curriculum with Python or Java. Effective C bridges this gap and brings C into the modern eracovering the modern C17 Standard as well as potential C2x features. With the aid of this instant classic, you'll soon be writing professional, portable, and secure C programs to power robust systems and solve real-world problems.
Robert C. Seacord introduces C and the C Standard Library while addressing best practices, common errors, and open debates in the C community. Developed together with other C Standards committee experts, Effective C will teach you how to debug, test, and analyze C programs. You'll benefit from Seacord's concise explanations of C language constructs and behaviors, and from his 40 years of coding experience.
You'll learn:
Effective C will teach you how to write professional, secure, and portable C code that will stand the test of time and help strengthen the foundation of the computing world.
Effective C: An Introduction to Professional C Programming
A detailed introduction to the C programming language for experienced programmers.
The world runs on code written in the C programming language, yet most schools begin the curriculum with Python or Java. Effective C bridges this gap and brings C into the modern eracovering the modern C17 Standard as well as potential C2x features. With the aid of this instant classic, you'll soon be writing professional, portable, and secure C programs to power robust systems and solve real-world problems.
Robert C. Seacord introduces C and the C Standard Library while addressing best practices, common errors, and open debates in the C community. Developed together with other C Standards committee experts, Effective C will teach you how to debug, test, and analyze C programs. You'll benefit from Seacord's concise explanations of C language constructs and behaviors, and from his 40 years of coding experience.
You'll learn:How to identify and handle undefined behavior in a C program The range and representations of integers and floating-point values How dynamic memory allocation works and how to use nonstandard functions How to use character encodings and types How to perform I/O with terminals and filesystems using C Standard streams and POSIX file descriptors How to understand the C compiler's translation phases and the role of the preprocessor How to test, debug, and analyze C programs
Effective C will teach you how to write professional, secure, and portable C code that will stand the test of time and help strengthen the foundation of the computing world.
The world runs on code written in the C programming language, yet most schools begin the curriculum with Python or Java. Effective C bridges this gap and brings C into the modern eracovering the modern C17 Standard as well as potential C2x features. With the aid of this instant classic, you'll soon be writing professional, portable, and secure C programs to power robust systems and solve real-world problems.
Robert C. Seacord introduces C and the C Standard Library while addressing best practices, common errors, and open debates in the C community. Developed together with other C Standards committee experts, Effective C will teach you how to debug, test, and analyze C programs. You'll benefit from Seacord's concise explanations of C language constructs and behaviors, and from his 40 years of coding experience.
You'll learn:
Effective C will teach you how to write professional, secure, and portable C code that will stand the test of time and help strengthen the foundation of the computing world.
49.99
In Stock
5
1
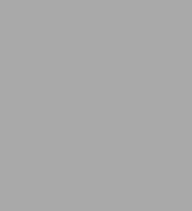
Effective C: An Introduction to Professional C Programming
272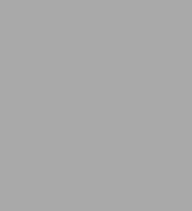
Effective C: An Introduction to Professional C Programming
272Paperback
$49.99
49.99
In Stock
Product Details
ISBN-13: | 9781718501041 |
---|---|
Publisher: | No Starch Press |
Publication date: | 08/04/2020 |
Pages: | 272 |
Product dimensions: | 6.90(w) x 9.10(h) x 0.80(d) |
About the Author
From the B&N Reads Blog