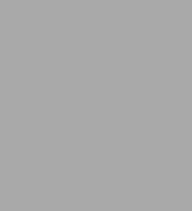
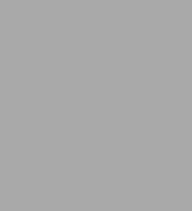
eBook
Available on Compatible NOOK devices, the free NOOK App and in My Digital Library.
Related collections and offers
Overview
Revised and updated for Elixir 1.7, Elixir in Action, Second Edition teaches you how to apply Elixir to practical problems associated with scalability, fault tolerance, and high availability. Along the way, you'll develop an appreciation for, and considerable skill in, a functional and concurrent style of programming.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the Technology
When you're building mission-critical software, fault tolerance matters. The Elixir programming language delivers fast, reliable applications, whether you're building a large-scale distributed system, a set of backend services, or a simple web app. And Elixir's elegant syntax and functional programming mindset make your software easy to write, read, and maintain.
About the Book
Elixir in Action, Second Edition teaches you how to build production-quality distributed applications using the Elixir programming language. Author Saša Jurić introduces this powerful language using examples that highlight the benefits of Elixir's functional and concurrent programming. You'll discover how the OTP framework can radically reduce tedious low-level coding tasks. You'll also explore practical approaches to concurrency as you learn to distribute a production system over multiple machines.
What's inside
- Updated for Elixir 1.7
- Functional and concurrent programming
- Introduction to distributed system design
- Creating deployable releases
About the Reader
You'll need intermediate skills with client/server applications and a language like Java, C#, or Ruby. No previous experience with Elixir required.
About the Author
Saša Jurić is a developer with extensive experience using Elixir and Erlang in complex server-side systems.
Table of Contents
- First steps
- Building blocks
- Control flow
- Data abstractions
- Concurrency primitives
- Generic server processes
- Building a concurrent system
- Fault-tolerance basics
- Isolating error effects
- Beyond GenServer
- Working with components
- Building a distributed system
- Running the system
Product Details
ISBN-13: | 9781638351658 |
---|---|
Publisher: | Manning |
Publication date: | 01/03/2019 |
Sold by: | SIMON & SCHUSTER |
Format: | eBook |
Pages: | 384 |
File size: | 2 MB |
About the Author
Table of Contents
Preface xiii
Acknowledgments xv
About this book xvii
About the author xxi
About the cover illustration xxii
1 First steps 1
1.1 About Erlang 1
High availability 3
Erlang concurrency 3
Server-side systems 5
The development platform 7
1.2 About Elixir 8
Code simplification 9
Composing functions 12
The big picture 13
1.3 Disadvantages 13
Speed 14
Ecosystem 14
2 Building Mocks 16
2.1 The interactive shell 17
2.2 Working with variables 18
2.3 Organizing your code 20
Modules 20
Functions 21
Function arity 25
Function visibility 26
Imports and aliases 27
Module attributes 28
Comments 30
2.4 Understanding the type system 30
Numbers 30
Atoms 31
Tuples 34
Lists 35
Immutability 38
Maps 41
Binaries and bitstrings 43
Strings 44
First-class functions 46
Other built-in types 49
Higher-level types 50
IO fats 54
2.5 Operators 55
2.6 Macros 56
2.7 Understanding the runtime 57
Modules and functions in the runtime 57
Starting the runtime 59
3 Control flow 63
3.1 Pattern matching 64
The match operator 64
Matching tuples 64
Matching constants 65
Variables in patterns 66
Matching lists 67
Matching maps 68
Matching bitstrings and binaries 69
Compound matches 71
General behavior 72
3.2 Matching with functions 72
Multiclause functions 73
Guards 76
Multiclause lambdas 78
3.3 Conditionals 79
Branching with multiclause functions 79
Classical branching constructs 81
The with special form 83
3.4 Loops and iterations 86
Iterating with recursion 87
Tail function calls 88
Higher-order functions 91
Comprehensions 95
Streams 97
4 Data abstractions 102
4.1 Abstracting with modules 104
Basic abstraction 104
Composing abstractions 106
Structuring data with maps 107
Abstracting with structs 108
Data transparency 112
4.2 Working with hierarchical data 114
Generating IDs 115
Updating entries 117
Immutable hierarchical updates 119
Iterative updates 121
Exercise: importing from a file 122
4.3 Polymorphism with protocols 124
Protocol basics 124
Implementing a protocol 125
Built-in protocols 126
5 Concurrency primitives 129
5.1 Concurrency in BEAM 130
5.2 Working with processes 132
Creating processes 133
Message passing 134
5.3 Stateful server processes 139
Server processes 139
Keeping a process state 144
Mutable state 145
Complex states 148
Registered processes 152
5.4 Runtime considerations 153
A process is sequential 153
Unlimited process mailboxes 155
Shared-nothing concurrency 156
Scheduler inner workings 157
6 Generic server processes 159
6.1 Building a generic server process 160
Plugging in with modules 160
Implementing the generic code 161
Using the generic abstraction 162
Supporting asynchronous requests 164
Exercise: refactoring the to-do server 166
6.2 Using GenServer 166
OTP behaviours 167
Plugging into GenServer 168
Handling requests 169
Handling plain messages 170
Other GenServer features 172
Process lifecycle 175
OTP-compliant processes 176
Exercise: GenServer-powered to-do server 177
7 Building a concurrent system 179
7.1 Working with the mix project 180
7.2 Managing multiple to-do lists 182
Implementing a cache 182
Writing tests 185
Analyzing process dependencies 188
7.3 Persisting data 189
Encoding and persisting 189
Using the database 191
Analyzing the system 194
Addressing the process bottleneck 195
Exercise: pooling and synchronizing 198
7.4 Reasoning with processes 199
8 Fault-tolerance basics 201
8.1 Runtime errors 202
Error types 203
Handling errors 204
8.2 Errors in concurrent systems 204
Linking processes 208
Monitors 210
8.3 Supervisors 211
Preparing the existing code 213
Starting the supervisor process 214
Child specification 216
Wrapping the supervisor 218
Using a callback module 218
Linking all processes 219
Restart frequency 222
9 Isolating error effects 224
9.1 Supervision trees 225
Separating loosely dependent parts 225
Rich process discovery 228
Via tuples 230
Registering database workers 232
Supervising database workers 234
Organizing the supervision tree 237
9.2 Starting processes dynamically 241
Registering to-do servers 241
Dynamic supervision 242
Finding to-do servers 243
Using temporary restart strategy 244
Testing the system 245
9.3 "Let it crash" 246
Processes that shouldn't crash 247
Handling expected errors 248
Preserving the state 249
10 Beyond GenServer 251
A if 10.1 Tasks 252
Awaited tasks 252
Non-awaited tasks 254
10.2 Agents 256
Basic use 256
Agents and concurrency 257
Agent-powered to-do server 259
Limitations of agents 260
10.3 ETS tables 263
Basic operations 265
ETS powered key/value store 268
Other ETS operations 271
Exercise: process registry 274
11 Working with components 277
11.1 OTP applications 278
Creating applications with the mix tool 278
The application behavior 280
Starling the application 280
Library applications 281
Creating a to-do application 282
The application folder structure 284
11.2 Working with dependencies 286
Adding a dependency 286
Adapting the pool 287
Visualizing the system 289
11.3 Building a web server 291
Choosing dependencies 291
Starting the server 292
Handling requests 293
Reasoning about the system 296
11.4 Configuring applications 300
Application environment 300
Varying configuration 301
Config script considerations 303
12 Building a distributed system 305
12.1 Distribution primitives 307
Starting a cluster 307
Communicating between nodes 309
Process discovery 311
Links and monitors 314
Other distribution services 315
12.2 Building a fault-tolerant cluster 317
Cluster design 318
The distributed to-do cache 318
Implementing a replicated database 323
Testing the system 326
Detecting partitions 327
Highly available systems 329
12.3 Network considerations 330
Node names 330
Cookies 331
Hidden nodes 331
Firewalls 332
13 Running the system 334
13.1 Running a system with Elixir tools 335
Using the mix and elixir commands 335
Running scripts 337
Compiling for production 338
13.2 OTP releases 339
Building a release with distillery 340
Using a release 341
Release contents 343
13.3 Analyzing system behavior 346
Debugging 347
Logging 348
Interacting with the system 348
Tracing 350
Index 353