Engineering Problem Solving With C++, Fourth Edition provides a clear, concise introduction to engineering problem solving with C++ as well as the object-oriented features of the C++ programming language. The authors’ proven five-step problem solving methodology is presented and then incorporated in every chapter of the text. The chapters in this text are designed to give the instructor flexibility in the ordering of topics with chapter topics covering the essentials of mathematical computations, character data, control structures, functions, arrays, classes, and pointers. Outstanding engineering and scientific applications are used throughout; all applications are centered around the theme of engineering challenges in the 21st century with an emphasis on incorporating real-world engineering and scientific examples and problems.
Engineering Problem Solving With C++, Fourth Edition provides a clear, concise introduction to engineering problem solving with C++ as well as the object-oriented features of the C++ programming language. The authors’ proven five-step problem solving methodology is presented and then incorporated in every chapter of the text. The chapters in this text are designed to give the instructor flexibility in the ordering of topics with chapter topics covering the essentials of mathematical computations, character data, control structures, functions, arrays, classes, and pointers. Outstanding engineering and scientific applications are used throughout; all applications are centered around the theme of engineering challenges in the 21st century with an emphasis on incorporating real-world engineering and scientific examples and problems.
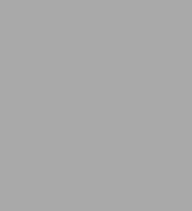
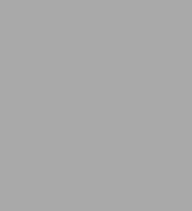
Paperback(New Edition)
-
SHIP THIS ITEMIn stock. Ships in 6-10 days.PICK UP IN STORE
Your local store may have stock of this item.
Available within 2 business hours
Related collections and offers
Overview
Engineering Problem Solving With C++, Fourth Edition provides a clear, concise introduction to engineering problem solving with C++ as well as the object-oriented features of the C++ programming language. The authors’ proven five-step problem solving methodology is presented and then incorporated in every chapter of the text. The chapters in this text are designed to give the instructor flexibility in the ordering of topics with chapter topics covering the essentials of mathematical computations, character data, control structures, functions, arrays, classes, and pointers. Outstanding engineering and scientific applications are used throughout; all applications are centered around the theme of engineering challenges in the 21st century with an emphasis on incorporating real-world engineering and scientific examples and problems.
Product Details
ISBN-13: | 9780134444291 |
---|---|
Publisher: | Pearson Education |
Publication date: | 03/08/2016 |
Edition description: | New Edition |
Pages: | 696 |
Product dimensions: | 7.30(w) x 9.00(h) x 1.00(d) |
Table of Contents
Introduction to Computing and Engineering1 Problem Solving
1.1 Historical Perspective
1.2 Recent Engineering Achievements
Changing Engineering Environment
1.3 Computing Systems
Computer Hardware
Computer Software
1.4 Data Representation and Storage
Number Systems
Data Types and Storage
1.5 An Engineering Problem-Solving Methodology
Summary
2 Simple C++ Programs
ENGINEERING CHALLENGE: Vehicle Performance
2.1 Program Structure
2.2 Constants and Variables
Scientific Notation
Numeric Data Types
Boolean Data Type
Character Data Type
String Data
Symbolic Constants
Auto Type Specifier
2.3 C++ Classes
Class Declaration
Class Implementation
2.4 Building C++ Solutions with IDEs: Xcode
Xcode
2.5 C++ Operators
Assignment Operator
Arithmetic Operators
Precedence of Operators
Overflow and Underflow
Increment and Decrement Operators
Abbreviated Assignment Operators
2.6 Standard Input and Output
The cout Object
Stream Objects
Manipulators
The cin Object
2.7 Building C++ Solutions with IDEs: NetBeans
NetBeans
2.8 Basic Functions Included in the C++ Standard Library
Elementary Math Functions
Trigonometric Functions
Hyperbolic Functions*
Character Functions
2.9 Problem Solving Applied: Velocity Computation
2.10 System Limitations
Summary
3 Control Structures: Selection
ENGINEERING CHALLENGE: Global Change
3.1 Algorithm Development
Top-Down Design
3.2 Structured Programming
Pseudocode
Evaluation of Alternative Solutions
3.3 Conditional Expressions
Relational Operators
Logical Operators
Precedence and Associativity
3.4 Selection Statements: if Statement
Simple if Statements
if/else Statement
3.5 Numerical Technique: Linear Interpolation
3.6 Problem Solving Applied: Freezing Temperature of Seawater
3.7 Selection Statements: switch Statement
3.8 Building C++ Solutions with IDEs: NetBeans
NetBeans
3.9 Defining Operators for Programmer-Defined Data Types
Summary
4 Control Structures: Repetition
ENGINEERING CHALLENGE: Data Collection
4.1 Algorithm Development
Pseudocode and Flowchart Description
4.2 Repetition Structures 156
while Loop
do/while Loop
for Loop
4.3 Problem Solving Applied: GPS
4.4 break and continue Statements
4.5 Structuring Input Loops
Counter-Controlled Loops
Sentinel-Controlled Loop
End-Of-Data Loop
4.6 Problem Solving Applied: Weather Balloons
4.7 Building C++ Solutions with IDEs: Microsoft Visual C++
Microsoft Visual C++
Summary
5 Working with Data Files
ENGINEERING CHALLENGE: Weather Prediction
5.1 Defining File Streams
Stream Class Hierarchy
ifstream Class
ofstream Class
5.2 Reading Data Files
Specified Number of Records
Trailer or Sentinel Signals
End-of-File
5.3 Generating a Data File
5.4 Problem Solving Applied: Data Filters–Modifying an HTML File
5.5 Error Checking
The Stream State
5.6 Numerical Technique: Linear Modeling
5.7 Problem Solving Applied: Ozone Measurements
5.8 Building C++ Solutions with IDEs: Xcode-Weather Patterns
Summary
6 Modular Programming with Functions
ENGINEERING CHALLENGE: Simulation
6.1 Modularity
6.2 Programmer-Defined Functions
Function Definition
Solution 1
Solution 2
Function Prototype
6.3 Parameter Passing
Pass by Value
Pass by Reference
Storage Class and Scope
6.4 Problem Solving Applied: Calculating a Center of Gravity
6.5 Random Numbers
Integer Sequences
Floating-Point Sequences
6.6 Problem Solving Applied: Instrumentation Reliability
6.7 Defining Class Methods
Public Interface
Accessor Methods
Mutator Methods
6.8 Problem Solving Applied: Design of Composite Materials
Solution 1:
Solution 2:
6.9 Numerical Technique: Roots of Polynomials
Polynomial Roots
Incremental-Search Technique
6.10 Problem Solving Applied: System Stability
Newton—Raphson Method
6.11 Numerical Technique: Integration
Integration Using the Trapezoidal Rule
Summary
7 One-Dimensional Arrays
ENGINEERING CHALLENGE: Tsunami Warning Systems
7.1 Arrays
Definition and Initialization
Pseudocode
Range-based for Statement
Computation and Output
Function Arguments
7.2 Problem Solving Applied: Hurricane Categories 357
7.3 Statistical Measurements
Simple Analysis
Variance and Standard Deviation
Custom Header Files
7.4 Problem Solving Applied: Speech Signal Analysis
7.5 Sorting and Searching Algorithms
Selection Sort
Search Algorithms
Unordered Lists
Ordered Lists
7.6 Problem Solving Applied: Tsunami Warning Systems
7.7 Character Strings
C Style String Definition and I/O
String Functions
7.8 The string Class
7.9 Building C++ Solutions with IDEs: Xcode Vegetation Maps
7.10 The vector class
Parameter Passing
7.11 Problem Solving Applied: Calculating Probabilities
Summary
8 Two-Dimensional Arrays
ENGINEERING CHALLENGE: Terrain Navigation
8.1 Two-Dimensional Arrays
Declaration and Initialization
Computations and Output
Function Arguments
8.2 Problem Solving Applied: Terrain Navigation
8.3 Two-Dimensional Arrays and the vector class
Function Arguments
8.4 Matrices
Determinant
Transpose
Matrix Addition and Subtraction
Matrix Multiplication
8.5 Numerical Technique: Solution to Simultaneous Equations
Graphical Interpretation
Gauss Elimination
8.6 Problem Solving Applied: Electrical Circuit Analysis
8.7 Higher-Dimensional Arrays
Summary
9 An Introduction to Pointers
ENGINEERING CHALLENGE: Weather Patterns
9.1 Addresses and Pointers
Address Operator
Pointer Assignment
Pointer Arithmetic
9.2 Pointers to Array Elements
One-Dimensional Arrays
Character Strings
Pointers as Function Arguments
9.3 Problem Solving Applied: El Niño-Southern Oscillation Data
9.4 Dynamic Memory Allocation
The new Operator
Dynamically Allocated Arrays
The delete Operator
9.5 Problem Solving Applied: Seismic Event Detection
9.6 Common Errors Using new and delete
9.7 Linked Data Structures
Linked Lists
Stacks
Queue 518
9.8 The C++ Standard Template Library
The list class
The stack class
The queue class
9.9 Problem Solving Applied: Concordance of a Text File 525
Summary
10 Advanced Topics
ENGINEERING CHALLENGE: Artificial Intelligence
10.1 Data Abstraction
Overloading Operators
The Pixel class
\Arithmetic Operators
friend Functions
Validating Objects
Bitwise Operators
10.2 Building C++ Solutions with IDEs: Xcode Image Files
10.3 Binary File Input and Output
Opening Binary Files
Reading and Writing Binary Files
Contents
10.4 Problem Solving Applied: Color Image Processing
10.5 Recursion
Factorial Function
Fibonacci Sequence
The BinaryTree class
10.6 Generic Programming
Function Templates
Class Templates
10.7 Inheritance
The Rectangle class
The Square Class
The Cube class
10.8 virtual Methods
10.9 Problem Solving Applied: Iterated Prisoner’s Dilemma
Summary
C++ Standard Library
B ASCII Character Codes
C Using MATLAB to Plot Data from ASCII Files
C++ Program to Generate a Data File
ASCII Data File Generated by the C++ Program 649
Generating a Plot with MATLAB
D References
E PRACTICE! Solutions
Index
Introduction
- to develop a consistent methodology for solving engineering problems,
- to present the object-based features of C++ while focusing on the fundamentals of programming,
- to illustrate the problem-solving process with C++ through a variety of engineering examples and applications,
- to provide an easy-to-understand, integrated introduction to function templates and classes defined in the Standard C++ Library.
To accomplish these objectives, Chapter 1 presents a five-step process that is used consistently in the rest of the text for solving engineering problems. Chapter 2 introduces the use of predefined objects and member functions in the discussion of data types and standard input and output. Chapters 3-5 present the fundamental capabilities of C++ forsolving engineering problems, including control structures, data files, and functions. Chapters 6 and 7 present arrays and introduce the reader to function templates and the vector class. Chapter 8 is an introduction to programmer-defined classes. Chapter 9 introduces the use of pointers, dynamic memory allocation, and classes defined in the Standard C++ library to implement dynamic data structures. Chapter 10 provides a more in-depth look at classes, including overloading operators, inheritance, and virtual functions. Throughout all these chapters, we present a large number of examples from many different engineering, science, and computer science disciplines. The solutions to these examples are developed using the five-step process and Standard C++.
Prerequisites
No prior experience with the computer is assumed. The mathematical prerequisites are college algebra and trigonometry. Of course, the initial material can be covered much faster if the student has used other computer languages or software tools.
Course Structure
The material in these chapters was selected to provide the basis for a one-term course in engineering and scientific computing. These chapters contain the essential topics of mathematical computations, character data, control structures, functions, arrays, classes, and pointers. Students with background in another computer language should be able to complete this material in one semester. A minimal course that provides only an introduction to C++ can be designed using the nonoptional sections of the text. (Optional sections are indicated in the Contents with an *.) Three ways to use the text, along with the recommended chapter sections, are
- Introduction to C++. Many freshman introductory courses introduce the student to several computer tools in addition to an introduction to a language. For these courses, we recommend covering the nonoptional sections of Chapters 1-7. This material introduces students to the fundamental capabilities of C++, and they will be able to write substantial programs using mathematical computations, character data, control structures, functions, and arrays.
- Problem Solving with C++. In a semester course devoted specifically to teaching students to master the C++ language, we recommend covering all nonoptional sections of Chapters 1-10. This material covers all the fundamental concepts of the C++ language, including mathematical computations, character data, control structures, functions, arrays, classes, and pointers.
- Problem Solving with C++ and Numerical Techniques. Upper-level students or students who are already familiar with other high-level languages will be able to cover the material in this text very quickly. In addition, they will be able to apply the numerical technique material to their other courses. Therefore, we recommend that these students cover all sections of Chapters 1-10, including the optional material.
The chapters in this text were designed to give the instructor flexibility in the ordering of topics, especially regarding the decision of when to cover classes: before or after arrays. The introductory chapter on classes does not depend on the chapters on arrays, and the chapters on arrays do not depend on the introductory chapter on classes. The dependency chart on the next page illustrates the dependency of chapters.
Problem-Solving Methodology
The emphasis on engineering and scientific problem solving is an integral part of the text. Chapter 1 introduces a five-step process for solving engineering problems using the computer:
- State the problem clearly.
- Describe the input and output information, and determine required data types.
- Work a simple example by hand.
- Develop an algorithm and convert it to a computer program.
- Test the solution with a variety of data.
To reinforce the development of problem-solving skills, each of these five steps is clearly identified each time that a complete engineering problem is solved. In addition, top-down design and stepwise refinement are presented with the use of decomposition outlines, pseudocode, and flowcharts.
Engineering and Scientific Applications
Throughout the text, emphasis is placed on incorporating real-world engineering and scientific examples and problems. This emphasis is centered around a theme of grand challenges, which include
- prediction of weather, climate, and global change
- computerized speech understanding
- mapping of the human genome
- improvements in vehicle performance
- enhanced oil and gas recovery
- simulation
Each chapter begins with a photograph and a discussion of some aspect of one of these grand challenges that provides a glimpse of some of the exciting and interesting areas in which engineers might work. Later in the chapter, we solve a problem that not only relates to the introductory problem, but also has applications in other problem solutions. The grand challenges are also referenced in many of the other examples and problems.
Standard C++
The statements presented and all programs developed use C++ standards developed by the International Standards Organization and American National Standards Institute (ISO/ANSI) C++ Standards committee. ISO and ANSI together have published the first international standard for the C++ programming language. By using Standard C++, students learn to write portable code that can be transferred from one computer platform to another. Many of the standard capabilities of the C++ programming language are discussed in the text. Additional components of the C++ standard library are discussed in Appendix A.
Software Engineering Concepts
Engineers and scientists are expected to develop and implement user-friendly and reusable computer solutions. Learning software engineering techniques is therefore crucial to successfully developing these computer solutions. Readability and documentation are stressed in the development of programs. Additional topics that relate to software engineering issues are discussed throughout the text and include issues such as software life cycle, portability, maintenance, modularity, recursion, abstraction, reusability, structured programming, validation, and verification.
Four Types of Problems
Learning any new skill requires practice at a number of different levels of difficulty. We have developed four types of exercises that are used throughout the text to develop problem-solving skills. The first set of exercises is Practice! problems. These are short-answer questions that relate to the section of material just presented. Most sections are immediately followed by a set of Practice! problems so that students can determine if they are ready to continue to the next section. Complete solutions to all the Practice! problems are included at the end of the text.
The Modify! problems are designed to provide hands-on experiences with the programs developed in the Problem-Solving Applied sections. In these sections, we develop a complete C++ program using the five-step process. The Modify! problems ask students to run the program (which is available on our Instructor's Resource CD) with different sets of data to test their understanding of how the program works and of the relationships among the engineering variables. These exercises also ask the students to make simple modifications to the program and then run the program to test their changes.
Most chapters end with a set of Exam Practice! problems, and every chapter includes a set of Programming Problems. The Exam Practice! problems are short-answer questions that relate to the material covered in the chapter. These problems help students determine how well they understand the features of C++ presented in the chapter. The Programming Problems are new problems that relate to a variety of engineering applications, and the level of difficulty ranges from very straightforward to longer project assignments. Each programming problem requires that the student develop a complete C++ program or function. Engineering data sets for many of the problems are included on our Instructor's Resource CD to use in testing. Solutions to all of the Exam Practice! and some of the Programming Problems are included at the end of the text.
Study and Programming Aids
Margin notes are used to help the reader not only identify the important concepts, but also easily locate specific topics. In addition, margin notes are used to identify programming style guidelines and debugging information. Style guidelines show students how to write C++ programs that incorporate good software discipline; debugging sections help students recognize common errors so that they can avoid them. The programming style notes are indicated with the margin note Style, and the debugging notes are indicated with a bug icon. Object oriented features of C++ are indicated with an OOP icon to help student recognize these features early in the text. Each Chapter Summary contains a summary of the style notes and debugging notes, plus a list of the Key Terms from the chapter and a C++ Statement Reference of the new statements, to make the book easy to use as a reference.
Optional Numerical Techniques
Numerical techniques that are commonly used in solving engineering problems are also discussed in optional sections in the chapters, and include interpolation, linear modeling (regression), root finding, numerical integration, and the solution to simultaneous equations. The concept of a matrix is also introduced and then illustrated using a number of examples. All of these topics are presented assuming only a trigonometry and college algebra background.
Appendices
To further enhance reference use, the appendices include a number of important topics. Appendix A contains a discussion components in the C++ standard library. Appendix B presents the ASCII character codes. Appendix D contains a list of references used throughout the text. MATLAB Appendix also included as Appendix C.
Instructor's Resource CD
An Instructor's Resource CD is available for instructors who adopt this text. The CD contains all of the example programs used in the text, complete solutions to most of the Programming Problems found at the end of each chapter, as well as data files to use with application problems and a complete set of PowerPoint slides to assist the instructor in preparing lecture notes.