Express in Action: Writing, building, and testing Node.js applications
Summary
Express in Action is a carefully designed tutorial that teaches you how to build web applications using Node and Express.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the Technology
Express.js is a web application framework for Node.js. Express organizes your server-side JavaScript into testable, maintainable modules. It provides a powerful set of features to efficiently manage routes, requests, and views along with beautiful boilerplate for your web applications. Express helps you concentrate on what your application does instead of managing time-consuming technical details.
About the Book
Express in Action teaches you how to build web applications using Node and Express. It starts by introducing Node's powerful traits and shows you how they map to the features of Express. You'll explore key development techniques, meet the rich ecosystem of companion tools and libraries, and get a glimpse into its inner workings. By the end of the book, you'll be able to use Express to build a Node app and know how to test it, hook it up to a database, and automate the dev process.
What's Inside
About the Reader
To get the most out of this book, you'll need to know the basics of web application design and be proficient with JavaScript.
About the Author
Evan Hahn is an active member of the Node and Express community and contributes to many open source JavaScript projects.
Table of Contents
1123661411
Express in Action is a carefully designed tutorial that teaches you how to build web applications using Node and Express.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the Technology
Express.js is a web application framework for Node.js. Express organizes your server-side JavaScript into testable, maintainable modules. It provides a powerful set of features to efficiently manage routes, requests, and views along with beautiful boilerplate for your web applications. Express helps you concentrate on what your application does instead of managing time-consuming technical details.
About the Book
Express in Action teaches you how to build web applications using Node and Express. It starts by introducing Node's powerful traits and shows you how they map to the features of Express. You'll explore key development techniques, meet the rich ecosystem of companion tools and libraries, and get a glimpse into its inner workings. By the end of the book, you'll be able to use Express to build a Node app and know how to test it, hook it up to a database, and automate the dev process.
What's Inside
- Simplify Node app setup with Express
- Testing Express applications
- Use Express for easy access to Node features
- Data storage with MongoDB
- Covers Express 4 and Express 5 alpha
About the Reader
To get the most out of this book, you'll need to know the basics of web application design and be proficient with JavaScript.
About the Author
Evan Hahn is an active member of the Node and Express community and contributes to many open source JavaScript projects.
Table of Contents
- PART 1 INTRO
- What is Express?
- The basics of Node.js
- Foundations of Express PART 2 CORE
- Middleware
- Routing
- Building APIs
- Views and templates: Pug and EJS PART 3 EXPRESS IN CONTEXT
- Persisting your data with MongoDB
- Testing Express applications
- Security
- Deployment: assets and Heroku
- Best practices
Express in Action: Writing, building, and testing Node.js applications
Summary
Express in Action is a carefully designed tutorial that teaches you how to build web applications using Node and Express.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the Technology
Express.js is a web application framework for Node.js. Express organizes your server-side JavaScript into testable, maintainable modules. It provides a powerful set of features to efficiently manage routes, requests, and views along with beautiful boilerplate for your web applications. Express helps you concentrate on what your application does instead of managing time-consuming technical details.
About the Book
Express in Action teaches you how to build web applications using Node and Express. It starts by introducing Node's powerful traits and shows you how they map to the features of Express. You'll explore key development techniques, meet the rich ecosystem of companion tools and libraries, and get a glimpse into its inner workings. By the end of the book, you'll be able to use Express to build a Node app and know how to test it, hook it up to a database, and automate the dev process.
What's Inside
About the Reader
To get the most out of this book, you'll need to know the basics of web application design and be proficient with JavaScript.
About the Author
Evan Hahn is an active member of the Node and Express community and contributes to many open source JavaScript projects.
Table of Contents
Express in Action is a carefully designed tutorial that teaches you how to build web applications using Node and Express.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the Technology
Express.js is a web application framework for Node.js. Express organizes your server-side JavaScript into testable, maintainable modules. It provides a powerful set of features to efficiently manage routes, requests, and views along with beautiful boilerplate for your web applications. Express helps you concentrate on what your application does instead of managing time-consuming technical details.
About the Book
Express in Action teaches you how to build web applications using Node and Express. It starts by introducing Node's powerful traits and shows you how they map to the features of Express. You'll explore key development techniques, meet the rich ecosystem of companion tools and libraries, and get a glimpse into its inner workings. By the end of the book, you'll be able to use Express to build a Node app and know how to test it, hook it up to a database, and automate the dev process.
What's Inside
- Simplify Node app setup with Express
- Testing Express applications
- Use Express for easy access to Node features
- Data storage with MongoDB
- Covers Express 4 and Express 5 alpha
About the Reader
To get the most out of this book, you'll need to know the basics of web application design and be proficient with JavaScript.
About the Author
Evan Hahn is an active member of the Node and Express community and contributes to many open source JavaScript projects.
Table of Contents
- PART 1 INTRO
- What is Express?
- The basics of Node.js
- Foundations of Express PART 2 CORE
- Middleware
- Routing
- Building APIs
- Views and templates: Pug and EJS PART 3 EXPRESS IN CONTEXT
- Persisting your data with MongoDB
- Testing Express applications
- Security
- Deployment: assets and Heroku
- Best practices
30.99
In Stock
5
1
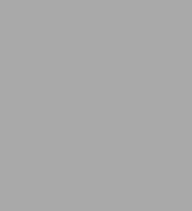
Express in Action: Writing, building, and testing Node.js applications
256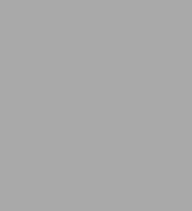
Express in Action: Writing, building, and testing Node.js applications
256
30.99
In Stock
From the B&N Reads Blog