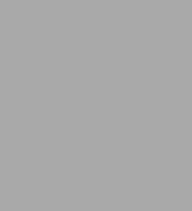
Hello World!: Computer Programming for Kids and Other Beginners
435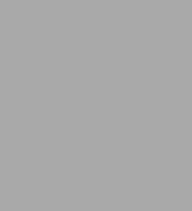
Hello World!: Computer Programming for Kids and Other Beginners
435Paperback(2nd Edition)
-
PICK UP IN STORECheck Availability at Nearby Stores
Available within 2 business hours
Related collections and offers
Overview
A gentle but thorough introduction to the world of computer programming, it is written in language a 12-year-old can follow, but anyone who wants to learn how to program a computer can use it. Even adults. Written by Warren Sande and his son, Carter, and reviewed by professional educators, this book is kid-tested and parent-approved.
ABOUT THIS BOOK
Learn to talk to your computer in its own language! Whether you want to create a game, start a business, or solve an important problem, the first step is learning to write your own programs. Programming is a fun challenge, and it's easy to get started!
This updated and revised edition of Hello World! introduces the world of computer programming in a clear and engaging style. Written by Warren Sande and his son, Carter, it is full of examples that will get you thinking and learning. Reviewed by professional educators, this book is kid-tested and parent-approved. You don't need to know anything about programming to use the book, just the basics of using a computer. If you can start a program and save a file, you'll be off and running!
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
WHAT'S INSIDE
- Explains concepts in clear language
- Lots of pictures, cartoons, and fun examples
- Complete set of practice questions and exercises
- Illustrated in full color
Hello World! uses Python, a programming language designed to be easy to learn. Using fun examples, it brings to life concepts of computing— looping, decisions, input and output, data structures, graphics—and many more.
ABOUT THE AUTHORS
Warren Sande is an electronic systems engineer who uses Python both as a "do anything" scripting language and as a way to teach computers and programming. Carter Sande is a high school student who is passionate about technology. When he's not fixing his school's network and helping his classmates recover lost homework, he likes to ride his bike and write retro video games.
TABLE OF CONTENTS
- Getting Started
- Remember This: Memory and Variables
- Basic Math
- Types of Data
- Input
- GUIs—Graphical User Interfaces
- Decisions, Decisions
- Loop the Loop
- Just for You—Comments
- Game Time
- Nested and Variable Loops
- Collecting Things Together—Lists and Dictionaries
- Functions
- Objects
- Modules
- Graphics
- Sprites and Collision Detection
- A New Kind of Input—Events
- Sound
- More GUIs
- Print Formatting and Strings
- File Input and Output
- Take a Chance—Randomness
- Computer Simulations
- Skier Explained
- Python Battle
- What's Next?
Product Details
ISBN-13: | 9781617290923 |
---|---|
Publisher: | Manning |
Publication date: | 12/21/2013 |
Edition description: | 2nd Edition |
Pages: | 435 |
Product dimensions: | 7.30(w) x 9.20(h) x 1.30(d) |
Age Range: | 10 - 16 Years |
About the Author
Carter Sande is a bright, curious, energetic, and thoughtful boy who loves computers, playing the piano, bouncing on the trampoline, and Mario. He has been playing and experimenting with computers from a young age.
Table of Contents
Preface xiii
Acknowledgments xix
About this book xxii
1 Getting Started 1
Installing Python 1
Starting Python with IDLE 2
Instructions, please 3
Interacting with Python 6
Time to program 8
Running your first program 10
If something goes wrong 11
Our second program 13
2 Remember This: Memory and Variables 16
Input, processing, output 16
Names 18
What's in a name? 23
Numbers and strings 24
How "variable" are they? 25
The new me 26
3 Basic Math 30
The four basic operations 31
Operators 31
Order of operations 32
Integer division: Quotient and remainder 34
Exponentiation: Raising to a power 35
Increment and decrement 37
Really big and really small 37
4 Types of Data 42
Changing types 42
Getting more information: type() 46
Type-conversion errors 46
5 Input 48
Input() 49
Putting the input on the same line 49
Inputting numbers 51
Input from the web 52
6 GUIs: Graphical User Interfaces 55
What's a GUI? 55
Our first GUI 56
GUI input 57
Pick your flavor 57
The number guessing game … again 61
Other GUI pieces 62
7 Decisions, Decisions 64
Testing, testing 64
Indenting 66
Am I seeing double? 67
Other kinds of tests 68
What happens if the test is false? 69
Testing for more than one condition 71
Using and 71
Using or 72
Using not 73
8 Loop the Loop 77
Counting loops 78
Using a counting loop 80
A shortcut: range() 81
A matter of style: Loop variable names 82
Counting by steps 85
Counting without numbers 86
While we're on the subject 87
Bailing out of a loop: break and continue 88
9 Just for You: Comments 92
Adding comments 92
Single-line comments 93
End-of-line comments 93
Multiline comments 94
Triple-quoted strings 94
Commenting style 95
Comments in this book 95
Commenting out 96
10 Game Time 97
Skier 97
11 Nested and Variable Loops 102
Nested loops 102
Variable loops 104
Variable nested loops 105
Even more variable nested loops 106
Using nested loops 108
Counting calories 111
12 Collecting Things Together: Lists and Dictionaries 115
What's a list? 115
Creating a list 116
Adding things to a list 116
Getting items from a list 118
"Slicing" a list 119
Modifying items 121
Other ways of adding to a list 122
Deleting from a list 123
Searching a list 125
Looping through a list 126
Sorting lists 127
Mutable and immutable 130
Lists of lists: Tables of data 131
Dictionaries 134
13 Functions 141
Functions: The building blocks 141
Passing arguments to a function 145
Functions that return a value 149
Variable scope 151
A bit of advice on naming variables 155
14 Objects 157
Objects in the real world 158
Objects in Python 158
Object = attributes + methods 160
Creating objects 160
An example class: HotDog 166
Hiding the data 170
Polymorphism and inheritance 171
Thinking ahead 173
15 Modules 176
What's a module? 176
Why use modules? 176
How do we create modules? 177
How do we use modules? 178
Namespaces 179
Standard modules 183
16 Graphics 187
Getting some help: Pygame 187
A Pygame window 188
Drawing in the window 189
Individual pixels 198
Images 203
Let's get moving! 205
Animation 206
Smoother animation 208
Bouncing the ball 209
Wrapping the ball 211
17 Sprites and Collision Detection 215
Sprites 215
Bump! Collision detection 221
Counting time 225
18 A New Kind of Input: Events 230
Events 230
Keyboard events 232
Mouse events 237
Timer events 238
Time for another game-PyPong 241
19 Sound 253
More help from Pygame: mixer 253
Making sounds vs. playing sounds 254
Playing sounds 254
Controlling volume 257
Playing background music 258
Repeating music 259
Adding sounds to PyPong 260
Adding music to PyPong 264
20 More GUIs 269
Working with PyQt 269
Qt Designer 270
Saving the GUI 272
Making our GUI do something 274
The return of event handlers 276
More useful GUIs 277
TempGUI 278
Creating the new GUI 279
Squashing a bug 283
What's on the menu? 284
21 Print Formatting and Strings 290
New lines 291
Horizontal spacing: Tabs 293
Inserting variables in strings 295
Number formatting 296
Formatting, the new way 301
Strings 'n' things 303
22 File Input and Output 311
What's a file? 312
Filenames 312
File locations 313
Opening a file 317
Reading a file 318
Text files and binary files 320
Writing to a file 321
Saving your stuff in files: pickle 325
Game time again-Hangman 327
23 Take a Chance: Randomness 334
What's randomness? 335
Rolling the dice 335
Creating a deck of cards 340
Crazy Eights 345
24 Computer Simulations 358
Modeling the real world 358
Lunar Lander 359
Keeping time 364
Time objects 365
Virtual Pet 371
25 Skier Explained 382
The skier 382
The obstacles 386
26 Making Network Connections with Sockets 395
What's the difference between text and bytes? 396
Servers 398
Getting data from the client 402
Making a chat server 402
27 What's Next? 415
For younger programmers 415
Python 416
Game programming and Pygame 416
Other game programming (non-Python) 416
Keep it BASIC 417
Websites 417
Mobile apps 417
Look around 417
Appendix A Variable Naming Rules 419
Appendix B Differences Between Python 3 and 2 421
Appendix C Answers to Self-Test Questions 424
List of Code Listings 455
Index 459