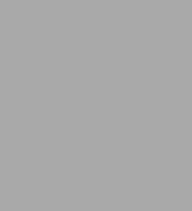
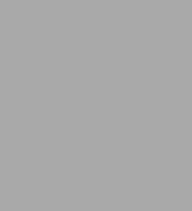
eBook
Available on Compatible NOOK devices, the free NOOK App and in My Digital Library.
Related collections and offers
Overview
Software Expert Kent Beck Presents a Catalog of Patterns Infinitely Useful for Everyday Programming
Great code doesn’t just function: it clearly and consistently communicates your intentions, allowing other programmers to understand your code, rely on it, and modify it with confidence. But great code doesn’t just happen. It is the outcome of hundreds of small but critical decisions programmers make every single day. Now, legendary software innovator Kent Beck—known worldwide for creating Extreme Programming and pioneering software patterns and test-driven development—focuses on these critical decisions, unearthing powerful “implementation patterns” for writing programs that are simpler, clearer, better organized, and more cost effective.
Beck collects 77 patterns for handling everyday programming tasks and writing more readable code. This new collection of patterns addresses many aspects of development, including class, state, behavior, method, collections, frameworks, and more. He uses diagrams, stories, examples, and essays to engage the reader as he illuminates the patterns. You’ll find proven solutions for handling everything from naming variables to checking exceptions.
Product Details
ISBN-13: | 9780132702553 |
---|---|
Publisher: | Pearson Education |
Publication date: | 10/23/2007 |
Series: | Addison-Wesley Signature Series (Beck) |
Sold by: | Barnes & Noble |
Format: | eBook |
Pages: | 176 |
File size: | 3 MB |
Age Range: | 18 Years |
About the Author
Read an Excerpt
This is a book about programming—specifically, about programming so other people can understand your code. There is no magic to writing code other people can read. It’s like all writing—know your audience, have a clear overall structure in mind, express the details so they contribute to the whole story. Java offers some good ways to communicate. The implementation patterns here are Java programming habits that result in readable code.
Another way to look at implementation patterns is as a way of thinking “What do I want to tell a reader about this code?” Programmers spend so much of their time in their own heads that trying to look at the world from someone else’s viewpoint is a big shift. Not just “What will the computer do with this code?” but “How can I communicate what I am thinking to people?” This shift in perspective is healthy and potentially profitable, since so much software development money is spent on understanding existing code.
There is an American game show called Jeopardy in which the host supplies answers and the contestants try to guess the questions. “A word describing being thrown through a window.” “What is ‘defenestration’?” “Correct.”
Coding is like Jeopardy. Java provides answers in the form of its basic constructs. Programmers usually have to figure out for themselves what the questions are, what problems are solved by each language construct. If the answer is “Declare a field as a
Set.” the question might be “How can I tell other programmers that a collection contains no duplicates?” The implementation patterns provide a catalog of the common problems in programming and the features of Java that address those problems.
Scope management is as important in book writing as it is in software development. Here are some things this book is not. It is not a style guide because it contains too much explanation and leaves the final decisions up to the reader. It is not a design book because it is mostly concerned with smaller-scale decisions, the kind programmers make many times a day. It’s not a patterns book because the format of the patterns is idiosyncratic and ad hoc (literally “built for a particular purpose”). It’s not a language book because, while it covers many Java language features, it assumes readers already know Java.
Actually this book is built on a rather fragile premise: that good code matters. I have seen too much ugly code make too much money to believe that quality of code is either necessary or sufficient for commercial success or widespread use. However, I still believe that quality of code matters even if it doesn’t provide control over the future. Businesses that are able to develop and release with confidence, shift direction in response to opportunities and competition, and maintain positive morale through challenges and setbacks will tend to be more successful than businesses with shoddy, buggy code.
Even if there was no long-term economic impact from careful coding I would still choose to write the best code I could. A seventy-year lifespan contains just over two billion seconds. That’s not enough seconds to waste on work I’m not proud of. Coding well is satisfying, both the act itself and the knowledge that others will be able to understand, appreciate, use, and extend my work.
In the end, then, this is a book about responsibility. As a programmer you have been given time, talent, money, and opportunity. What will you do to make responsible use of these gifts? The pages that follow contain my answer to this question for me: code for others as well as myself and my buddy the CPU.
Table of Contents
Preface xv
Chapter 1: Introduction 1
Tour Guide 3
And Now... 4
Chapter 2: Patterns 5
Chapter 3: A Theory of Programming 9
Values 10
Principles 13
Conclusion 18
Chapter 4: Motivation 19
Chapter 5: Class 21
Class 22
Simple Superclass Name 23
Qualified Subclass Name 24
Abstract Interface 24
Interface 26
Abstract Class 26
Versioned Interface 27
Value Object 28
Specialization 31
Subclass 32
Implementor 34
Inner Class 34
Instance-Specific Behavior 36
Conditional 36
Delegation 38
Pluggable Selector 40
Anonymous Inner Class 41
Library Class 41
Conclusion 42
Chapter 6: State 43
State 44
Access 45
Direct Access 46
Indirect Access 47
Common State 47
Variable State 48
Extrinsic State 50
Variable 50
Local Variable 51
Field 52
Parameter 53
Collecting Parameter 55
Optional Parameter 56
Var Args 56
Parameter Object 57
Constant 58
Role-Suggesting Name 58
Declared Type 60
Initialization 61
Eager Initialization 61
Lazy Initialization 62
Conclusion 62
Chapter 7: Behavior 63
Control Flow 64
Main Flow 64
Message 65
Choosing Message 65
Double Dispatch 66
Decomposing (Sequencing) Message 67
Reversing Message 67
Inviting Message 68
Explaining Message 69
Exceptional Flow 70
Guard Clause 70
Exception 72
Checked Exceptions 72
Exception Propagation 73
Conclusion 73
Chapter 8: Methods 75
Composed Method 77
Intention-Revealing Name 79
Method Visibility 80
Method Object 82
Overridden Method 83
Overloaded Method 83
Method Return Type 84
Method Comment 85
Helper Method 85
Debug Print Method 86
Conversion 87
Conversion Method 87
Conversion Constructor 88
Creation 88
Complete Constructor 89
Factory Method 90
Internal Factory 91
Collection Accessor Method 91
Boolean Setting Method 93
Query Method 93
Equality Method 94
Getting Method 95
Setting Method 96
Safe Copy 97
Conclusion 98
Chapter 9: Collections 99
Metaphors 100
Issues 101
Interfaces 103
Implementations 107
Collections 110
Extending Collections 114
Conclusion 115
Chapter 10: Evolving Frameworks 117
Changing Frameworks without Changing Applications 117
Incompatible Upgrades 118
Encouraging Compatible Change 120
Conclusion 129
Appendix A: Performance Measurement 131
Example 131
API 132
Implementation 133
MethodTimer 134
Canceling Overhead 136
Tests 136
Conclusion 142
Bibliography 145
General Programming 145
Philosophy 147
Java 148
Index 149
What People are Saying About This
“Kent is a master at creating code that communicates well, is easy to understand, and is a pleasure to read. Every chapter of this book contains excellent explanations and insights into the smaller but important decisions we continuously have to make when creating quality code and classes.”
–Erich Gamma, IBM Distinguished Engineer
“Many teams have a master developer who makes a rapid stream of good decisions all day long. Their code is easy to understand, quick to modify, and feels safe and comfortable to work with. If you ask how they thought to write something the way they did, they always have a good reason. This book will help you become the master developer on your team. The breadth and depth of topics will engage veteran programmers, who will pick up new tricks and improve on old habits, while the clarity makes it accessible to even novice developers.”
–Russ Rufer, Silicon Valley Patterns Group
“Many people don’t realize how readable code can be and how valuable that readability is. Kent has taught me so much, I’m glad this book gives everyone the chance to learn from him.”
–Martin Fowler, chief scientist, ThoughtWorks
“Code should be worth reading, not just by the compiler, but by humans. Kent Beck distilled his experience into a cohesive collection of implementation patterns. These nuggets of advice will make your code truly worth reading.”
–Gregor Hohpe, author of Enterprise Integration Patterns
“In this book Kent Beck shows how writing clear and readable code follows from the application of simple principles. Implementation Patterns will help developers write intention revealing code that is both easy to understand and flexible towards future extensions. A must read for developers who are serious about their code.”
–Sven Gorts
“Implementation Patterns bridges the gap between design and coding. Beck introduces a new way of thinking about programming by basing his discussion on values and principles.”
–Diomidis Spinellis, author of Code Reading and Code Quality
Preface
This is a book about programming—specifically, about programming so other people can understand your code. There is no magic to writing code other people can read. It’s like all writing—know your audience, have a clear overall structure in mind, express the details so they contribute to the whole story. Java offers some good ways to communicate. The implementation patterns here are Java programming habits that result in readable code.
Another way to look at implementation patterns is as a way of thinking “What do I want to tell a reader about this code?” Programmers spend so much of their time in their own heads that trying to look at the world from someone else’s viewpoint is a big shift. Not just “What will the computer do with this code?” but “How can I communicate what I am thinking to people?” This shift in perspective is healthy and potentially profitable, since so much software development money is spent on understanding existing code.
There is an American game show called Jeopardy in which the host supplies answers and the contestants try to guess the questions. “A word describing being thrown through a window.” “What is ‘defenestration’?” “Correct.”
Coding is like Jeopardy. Java provides answers in the form of its basic constructs. Programmers usually have to figure out for themselves what the questions are, what problems are solved by each language construct. If the answer is “Declare a field as a
Set.” the question might be “How can I tell other programmers that a collection contains no duplicates?” The implementation patterns provide a catalog of the common problems in programming and the features of Java that address those problems.
Scope management is as important in book writing as it is in software development. Here are some things this book is not. It is not a style guide because it contains too much explanation and leaves the final decisions up to the reader. It is not a design book because it is mostly concerned with smaller-scale decisions, the kind programmers make many times a day. It’s not a patterns book because the format of the patterns is idiosyncratic and ad hoc (literally “built for a particular purpose”). It’s not a language book because, while it covers many Java language features, it assumes readers already know Java.
Actually this book is built on a rather fragile premise: that good code matters. I have seen too much ugly code make too much money to believe that quality of code is either necessary or sufficient for commercial success or widespread use. However, I still believe that quality of code matters even if it doesn’t provide control over the future. Businesses that are able to develop and release with confidence, shift direction in response to opportunities and competition, and maintain positive morale through challenges and setbacks will tend to be more successful than businesses with shoddy, buggy code.
Even if there was no long-term economic impact from careful coding I would still choose to write the best code I could. A seventy-year lifespan contains just over two billion seconds. That’s not enough seconds to waste on work I’m not proud of. Coding well is satisfying, both the act itself and the knowledge that others will be able to understand, appreciate, use, and extend my work.
In the end, then, this is a book about responsibility. As a programmer you have been given time, talent, money, and opportunity. What will you do to make responsible use of these gifts? The pages that follow contain my answer to this question for me: code for others as well as myself and my buddy the CPU.