Introduction to Programming Using Sml / Edition 1 available in Paperback

Introduction to Programming Using Sml / Edition 1
- ISBN-10:
- 0201398206
- ISBN-13:
- 9780201398205
- Pub. Date:
- 07/21/1999
- Publisher:
- Addison-Wesley
- ISBN-10:
- 0201398206
- ISBN-13:
- 9780201398205
- Pub. Date:
- 07/21/1999
- Publisher:
- Addison-Wesley

Introduction to Programming Using Sml / Edition 1
Buy New
$39.00Buy Used
$99.99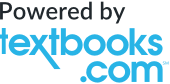
-
SHIP THIS ITEM— This item is available online through Marketplace sellers.
-
PICK UP IN STORE
Your local store may have stock of this item.
Available within 2 business hours
This item is available online through Marketplace sellers.
-
SHIP THIS ITEM
Temporarily Out of Stock Online
Please check back later for updated availability.
Overview
- Attractive and reader-friendly presentation
- Clear and careful explanations
- A rich collection of programming problems and a wide variety of examples
- Coverage of modelling and abstraction using data structures and the SML module system
- Overview and statement of objectives at the start of each chapter
- An introduction to producing technical documentation based on the SML module system
- Extensive material in the appendices covering the SML language and module system and selected parts of the SML basis library
Product Details
ISBN-13: | 9780201398205 |
---|---|
Publisher: | Addison-Wesley |
Publication date: | 07/21/1999 |
Series: | International Computer Science Series |
Edition description: | New Edition |
Pages: | 355 |
Product dimensions: | 6.77(w) x 9.20(h) x 0.71(d) |
About the Author
Michael Hansen is an Associate Professor in the Department of Information Technology at the Technical University of Denmark. He has written numerous papers in the areas of formal methods, real-time and hybrid systems.
Hans Rischel is an Associate Professor in the Department of Information Technology at the Technical University of Denmark. He has written numerous papers in the areas of formal methods, real-time and hybrid systems.
Read an Excerpt
PREFACE:
The topics of this book are programming and program design. The contents are used in the introductory programming course in the Informatics Programme at the Technical University of Denmark. This is the first course on programming that many of the students attend. The contents are furthermore used in a programming course for electrical engineers in a later semester. The emphasis is on programming and program design with a systematic use of lists, trees, sets and tables to build models and programs. The book does not cover efficient implementations using specialized representations of these data structures, as this topic is covered in other textbooks on 'algorithms and data structures'.
It is a goal of the book to bring theory to practical use. The examples and exercises in the book teach the student how to use basic, theoretically well-understood, concepts from computer science in problem solving in order to achieve succinct and elegant programs and program designs which can be communicated to other people. The book does, however, avoid formalistic explanations of the theory. Fundamental concepts such as bindings of identifiers and environments are explained in an informal but precise way such that the students get the right intuitive understanding. The presented concepts are programming language independent, and the book is therefore a general book on programming.
Throughout the book we use Standard ML (abbreviated SML) as the programming language. The reasons for this choice are:
The language is very powerful in expressing structured data as well as computations on such data. This enables students to solve interesting problems from thevery beginning of the course.
The different SML systems have a simple interactive interface to the user. This allows us to downplay the role of I/O while solving difficult problems in a brief and elegant way.
The language is close to common mathematical notation. This means that it is not very hard for students to learn the syntax of programs.
There is an extensive standard library, and there are several SML systems running on a number of platforms.
The language has a complete, formal semantics. Based on this formal semantics, we give a clear (informal) semantics of the programming language constructs, so that students can appreciate a language with a well-defined semantics.
We use the Moscow ML system in our course, which has turned out to be a fast and very reliable system with moderate resource demands. The SML-mode of the Emacs editor gives a pleasant environment where the user can easily switch between program editing, compilation and program runs. The students can get the system on their own PC so that they can use the system in their home work. The distribution of the system is easy as a complete ML system for a PC can be housed on a single floppy disk.
The chapters of the book are:
- Getting started
- Basic values and operators
- Tuples and records
- Problem solving I
- Lists
- Problem solving II
- Tagged values and partial functions
- Finite trees
- Higher-order functions
- Finite sets
- Modules
- Tables
- Problem solving III
- Input/output in SML
- Interactive programs
- Problem solving IV
- Iteration
- Imperative programming
The main organization of the first 12 chapters follows the data types: simple types, tuples, lists, trees, sets and tables -with the associated operators. Applications of these types, with associated operators, are illustrated through a large collection of interesting programming problems.
The chapters on 'problem solving' define a standard way of writing down the solution to a programming problem. This standard emphasizes specification of interfaces by means of signatures as described in Chapter 11 on the module system of SML.
The chapter on interactive programming introduces the concepts of I/O actions and dialogue automata for designing such programs, and this is supplemented by the notion of abstract syntax for commands in the following chapter: 'Problem solving IV'.
The chapter on iteration gives a few basic techniques, which iii some cases can give more efficient implementations. The last chapter introduces various aspects of imperative programming and imperative-data structures, together with the imperative operators.
The book has the following appendices:
- SML systems
- Overview of Standard ML
- Overview of the SML module system
- Selected parts of the SML basis library
- Modules of sets and tables
- The ASCII alphabet
- Further reading
Appendix A gives pointers to where to find information about existing SML systems. Appendices B and C give an overview of the SML language. The description is based on the mathematical semantics of SML, but it is presented in an informal way. Appendix D is a reference to selected parts of the Standard ML Basis Library. Appendix E contains modules for sets and tables which are used in main text. Appendix F gives a table of the ASCII alphabet. Appendix G contains some references for further reading.
The following WWW pages describe how we use the book in our course:
...
Table of Contents
(Each chapter concludes with a Summary and Exercises.)
1. Getting Started.
Simple Function Declarations.
Comments.
Recursion.
The Power Function.
About Types and Type Checking.
Bindings and Environments.
2. Basic Values and Operators.
Expressions, Precedence, Association.
Euclid'S Algorithm.
Evaluations with Environments.
Characters and Strings.
Truth Values.
The
If-Then-Else
Expression. Overloaded Operators.
Type Inference.
3. Tuples and Records.
Tuple Patterns.
Infix Functions on Pairs.
Records.
Record Patterns.
Type Declarations.
Locally Declared Identifiers.
4. Problem Solving I.
Solution 1.
Solution 2.
Comparing the Solutions.
A Solution using Records.
5. Lists.
The 'Cons' Operator.
List Patterns.
Append and Reverse; Polymorphic Types.
Polymorphic Values.
A Library of List Functions.
Membership; Equality Types.
Type Inference.
6. Problem Solving Ii.
Programming.
Test.
7. Tagged Values and Partial Functions.
The Case-Expression.
Enumeration Types.
The Order Type.
Partial Functions: The Option Datatype.
Exception Handling.
The Eight Queens Problem.
8. Finite Trees.
Symbolic Differentiation.
Trees of Ancestors; Traversal of a Tree.
Mutual Recursion.
Parameterized Datatypes.
Electrical Circuits.
Abstract Types.
9. Higher-Order Functions.
Value Declarations of Recursive Functions.
The
Map
Function. Declaring Higher order Functions.
Higher-Order List Functions.
Functional Composition.
Tree Recursion.
Type Inference For Higher-Order Functions.
Closures.
Static Binding.
Lazy Evaluation.
10. Finite Sets.
Operations on Sets.
An Abstype for Sets.
Other Representations of Sets.
11. Modules.
Specifications and Signatures.
Signatures and Structures.
Further Facilities.
Fixity of Identifiers.
Functors.
12. Tables.
A Representation of Tables.
Operations on Tables.
13. Problem Solving Iii.
A Sample Run.
Programming.
Test.
14. Input/Output In Sml.
Output Streams.
Simple Input and Output.
Use of Conversions.
Sequential Composition.
Input/Output of Composite Data.
Input with Prompt and Validation.
15. Interactive Programs.
Actions.
Action Schemas.
Functions for Action Schemas.
Dialogue Automaton.
Dialogue Program.
Action Refinement.
16. Problem Solving Iv.
Programming.
Test.
17. Iteration.
Two Problems.
Solutions: Accumulating Parameters.
Iteration.
18. Imperative Programming.
Operations on the Sml Store.
References and Polymorphism.
Arrays.
The
While
Loop. Imperative Data Structures.
Appendices.
A. Sml Systems.
B. Overview of Standard Ml.
Syntax.
Value, Environment And Store.
Types.
Semantics.
C. Overview of the Sml Module System.
Syntax.
Interfaces and Environments.
Semantics.
D. Selected Parts of the Sml Basis Library.
Numbers.
Characters And Strings.
Lists.
Vectors.
Arrays.
Timers and Time.
Date.
Conversions.
Text Input.
Text Output.
E. Modules of Sets and Tables.
Tables.
Signatures.
Structures.
F. The Ascii Alphabet.
G. Further Reading.
Index.
Preface
The topics of this book are programming and program design. The contents are used in the introductory programming course in the Informatics Programme at the Technical University of Denmark. This is the first course on programming that many of the students attend. The contents are furthermore used in a programming course for electrical engineers in a later semester. The emphasis is on programming and program design with a systematic use of lists, trees, sets and tables to build models and programs. The book does not cover efficient implementations using specialized representations of these data structures, as this topic is covered in other textbooks on 'algorithms and data structures'.
It is a goal of the book to bring theory to practical use. The examples and exercises in the book teach the student how to use basic, theoretically well-understood, concepts from computer science in problem solving in order to achieve succinct and elegant programs and program designs which can be communicated to other people. The book does, however, avoid formalistic explanations of the theory. Fundamental concepts such as bindings of identifiers and environments are explained in an informal but precise way such that the students get the right intuitive understanding. The presented concepts are programming language independent, and the book is therefore a general book on programming.
Throughout the book we use Standard ML (abbreviated SML) as the programming language. The reasons for this choice are:
The language is very powerful in expressing structured data as well as computations on such data. This enables students to solve interesting problems from the verybeginning of the course.
The different SML systems have a simple interactive interface to the user. This allows us to downplay the role of I/O while solving difficult problems in a brief and elegant way.
The language is close to common mathematical notation. This means that it is not very hard for students to learn the syntax of programs.
There is an extensive standard library, and there are several SML systems running on a number of platforms.
The language has a complete, formal semantics. Based on this formal semantics, we give a clear (informal) semantics of the programming language constructs, so that students can appreciate a language with a well-defined semantics.
We use the Moscow ML system in our course, which has turned out to be a fast and very reliable system with moderate resource demands. The SML-mode of the Emacs editor gives a pleasant environment where the user can easily switch between program editing, compilation and program runs. The students can get the system on their own PC so that they can use the system in their home work. The distribution of the system is easy as a complete ML system for a PC can be housed on a single floppy disk.
The chapters of the book are:
- Getting started
- Basic values and operators
- Tuples and records
- Problem solving I
- Lists
- Problem solving II
- Tagged values and partial functions
- Finite trees
- Higher-order functions
- Finite sets
- Modules
- Tables
- Problem solving III
- Input/output in SML
- Interactive programs
- Problem solving IV
- Iteration
- Imperative programming
The main organization of the first 12 chapters follows the data types: simple types, tuples, lists, trees, sets and tables -with the associated operators. Applications of these types, with associated operators, are illustrated through a large collection of interesting programming problems.
The chapters on 'problem solving' define a standard way of writing down the solution to a programming problem. This standard emphasizes specification of interfaces by means of signatures as described in Chapter 11 on the module system of SML.
The chapter on interactive programming introduces the concepts of I/O actions and dialogue automata for designing such programs, and this is supplemented by the notion of abstract syntax for commands in the following chapter: 'Problem solving IV'.
The chapter on iteration gives a few basic techniques, which iii some cases can give more efficient implementations. The last chapter introduces various aspects of imperative programming and imperative-data structures, together with the imperative operators.
The book has the following appendices:
- SML systems
- Overview of Standard ML
- Overview of the SML module system
- Selected parts of the SML basis library
- Modules of sets and tables
- The ASCII alphabet
- Further reading
Appendix A gives pointers to where to find information about existing SML systems. Appendices B and C give an overview of the SML language. The description is based on the mathematical semantics of SML, but it is presented in an informal way. Appendix D is a reference to selected parts of the Standard ML Basis Library. Appendix E contains modules for sets and tables which are used in main text. Appendix F gives a table of the ASCII alphabet. Appendix G contains some references for further reading.
The following WWW pages describe how we use the book in our course:
http://www.it.dtu.dk/introSML
Acknowledgments
We have received numerous comments and suggestions from many colleagues and students. Special thanks go to Jens Thyge Kristensen for his enthusiastic support in reading through all versions of the manuscript and providing many recommendations about the overall structure of the book as well as details of each chapter. Also, Ken Larsen, Jakob Lichtenberg, Anders P. Ravn, Peter Sestoft, Simon Mørk and Torben Hoffmann have given many important suggestions. Furthermore, the final version of the book has been strongly influenced by the corrections and suggestions from the anonymous referees.
The book The Definition of Standard ML (Revised), by Milner, Tofte, Harper and MacQueen has been an invaluable reference for us during the writing of this book. Furthermore, we are indebted to Peter Sestoft for his great help and endless patience in explaining to us details of the SML language.
Our sincere thanks go to the late Disa la Cour for proof reading a draft version of this book and for trying to improve our English writing.
Finally, we are grateful to Dines Bjørner who in the first place taught us the use of mathematical concepts in software development.