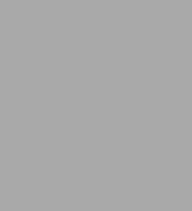
iOS 9 Swift Programming Cookbook: Solutions and Examples for iOS Apps
319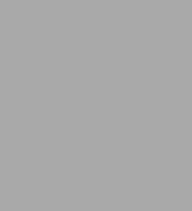
iOS 9 Swift Programming Cookbook: Solutions and Examples for iOS Apps
319Paperback
-
PICK UP IN STORECheck Availability at Nearby Stores
Available within 2 business hours
Related collections and offers
Overview
Ready to build stunning apps for iPhone, iPad, and Apple Watch? This cookbook—completely rewritten with all-new material—provides 90 proven solutions for tackling the latest features in iOS 9 and watchOS 2.0. Written exclusively in Apple’s Swift language, these code-rich recipes show you how to use dynamic user interfaces, interactive maps, multitasking functionality, Apple’s new UI Testing framework, and many other features.
This cookbook is ideal for intermediate and advanced iOS developers looking to work with the newest versions of Apple’s mobile operating systems. Each recipe includes reusable code, available on GitHub, that you can put to work right away.
- Work with new features in Swift 2, Xcode 7, and Interface Builder
- Build standalone apps for Apple Watch
- Create vibrant user interfaces with new UIKit features
- Use Swift to connect with the iOS contacts database
- Block ads or obtrusive content with Safari Content Blockers
- Make your app content searchable with Spotlight APIs
- Add Picture in Picture playback functionality to iPad apps
- Take advantage of MapKit and Core Location updates
- Use Apple’s new UI Testing framework
- Liven up your UI with gravity and turbulence fields
Product Details
ISBN-13: | 9781491936696 |
---|---|
Publisher: | O'Reilly Media, Incorporated |
Publication date: | 01/08/2016 |
Pages: | 319 |
Sales rank: | 1,103,724 |
Product dimensions: | 7.00(w) x 9.20(h) x 0.90(d) |
About the Author
Table of Contents
Preface iii
1 Swift 2.0, Xcode 7, and Interface Builder 1
1.1 Handling Errors in Swift 1
1.2 Specifying Preconditions for Methods 3
1.3 Ensuring the Execution of Code Blocks Before Exiting Methods 4
1.4 Checking for API Availability 6
1.5 Categorizing and Downloading Assets to Get Smaller Binaries 7
1.6 Exporting Device-Specific Binaries 11
1.7 Linking Separate Storyboards Together 12
1.8 Adding Multiple Buttons to the Navigation Bar 13
1.9 Optimizing Your Swift Code 14
1.10 Showing the Header View of Your Swift Classes 18
1.11 Creating Your Own Set Types 19
1.12 Conditionally Extending a Type 20
1.13 Building Equality Functionality into Your Own Types 22
1.14 Looping Conditionally Through a Collection 24
1.15 Designing Interactive Interface Objects in Playgrounds 25
1.16 Grouping Switch Statement Cases Together 28
1.17 Bundling and Reading Data in Your Apps 28
2 Apple Watch 33
2.1 Downloading Files onto the Apple Watch 35
2.2 Noticing Changes in Pairing State Between the iOS and Watch Apps 39
2.3 Transferring Small Pieces of Data to and from the Watch 42
2.4 Transferring Dictionaries in Queues to and from the Watch 52
2.5 Transferring Files to and from the Watch 56
2.6 Communicating Interactively Between iOS and watchOS 60
2.7 Setting Up Apple Watch for Custom Complications 69
2.8 Constructing Small Complications with Text and Images 76
2.9 Displaying Time Offsets in Complications 87
2.10 Displaying Dates in Complications 94
2.11 Displaying Times in Complications 100
2.12 Displaying Time Intervals in Complications 106
2.13 Recording Audio in Your Watch App 112
2.14 Playing Local and Remote Audio and Video in Your Watch App 115
3 The User Interface 119
3.1 Arranging Your Components Horizontally or Vertically 119
3.2 Customizing Stack Views for Different Screen Sizes 121
3.3 Creating Anchored Constraints in Code 125
3.4 Allowing Users to Enter Text in Response to Local and Remote Notifications 130
3.5 Dealing with Stacked Views in Code 134
3.6 Showing Web Content in Safari View Controller 136
3.7 Laying Out Text-Based Content on Your Views 137
3.8 Improving Touch Rates for Smoother UI Interactions 138
3.9 Supporting Right-to-Left Languages 141
3.10 Associating Keyboard Shortcuts with View Controllers 146
3.11 Recording the Screen and Sharing the Video 147
4 Contacts 155
4.1 Creating Contacts 156
4.2 Searching for Contacts 161
4.3 Updating Contacts 166
4.4 Deleting Contacts 168
4.5 Formatting Contact Data 170
4.6 Picking Contacts with the Prebuilt System UI 174
4.7 Creating Contacts with a Prebuilt System UI 180
4.8 Displaying Contacts with a Prebuilt System UI 182
5 Extensions 185
5.1 Creating Safari Content Blockers 185
5.2 Creating Shared Links for Safari 190
5.3 Maintaining Your Apps Indexed Content 193
6 Web and Search 199
6.1 Making Your App's Content Searchable 199
6.2 Making User Activities Searchable 203
6.3 Deleting Your App's Searchable Content 206
7 Multitasking 209
7.1 Adding Picture in Picture Playback Functionality 209
7.2 Handling Low Power Mode and Providing Alternatives 215
8 Maps and Location 219
8.1 Requesting the User's Location a Single Time 219
8.2 Requesting the User's Location in Background 221
8.3 Customizing the Tint Color of Pins on the Map 222
8.4 Providing Detailed Pin Information with Custom Views 225
8.5 Displaying Traffic, Scale, and Compass Indicators on the Map 227
8.6 Providing an ETA for Transit Transport Type 229
8.7 Launching the iOS Maps App in Transit Mode 232
8.8 Showing Maps in Flyover Mode 233
9 UI Testing 235
9.1 Preparing Your Project for UI Testing 235
9.2 Automating UI Test Scripts 238
9.3 Testing Text Fields, Buttons, and Labels 241
9.4 Finding UI Components 243
9.5 Long-Pressing on UI Elements 246
9.6 Typing Inside Text Fields 248
9.7 Swiping on UI Elements 250
9.8 Tapping UI Elements 251
10 Core Motion 253
10.1 Querying Pace and Cadence Information 254
10.2 Recording and Reading Accelerometer Data 255
11 Security 257
11.1 Protecting Your Network Connections with ATS 257
11.2 Binding Keychain Items to Passcode and Touch ID 259
11.3 Opening URLs Safely 261
11.4 Authenticating the User with Touch ID and Timeout 262
12 Multimedia 265
12.1 Reading Out Text with the Default Siri Alex Voice 265
12.2 Downloading and Preparing Remote Media for Playback 267
12.3 Enabling Spoken Audio Sessions 269
13 UI Dynamics 273
13.1 Adding a Radial Gravity Field to Your UI 273
13.2 Creating a Linear Gravity Field on Your UI 278
13.3 Creating Turbulence Effects with Animations 281
13.4 Adding Animated Noise Effects to Your UI 283
13.5 Creating a Magnetic Effect Between UI Components 285
13.6 Designing a Velocity Field on Your UI 288
13.7 Handling Nonrectangular Views 290
Index 297