Java: An Eventful Approach / Edition 1 available in Paperback
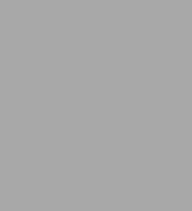
- ISBN-10:
- 0131424157
- ISBN-13:
- 9780131424159
- Pub. Date:
- 07/29/2005
- Publisher:
- Pearson Education
- ISBN-10:
- 0131424157
- ISBN-13:
- 9780131424159
- Pub. Date:
- 07/29/2005
- Publisher:
- Pearson Education
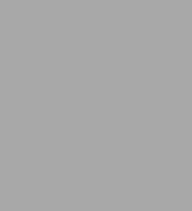
Buy New
$213.32Buy Used
$52.02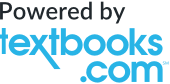
-
SHIP THIS ITEM— This item is available online through Marketplace sellers.
-
PICK UP IN STORECheck Availability at Nearby Stores
Available within 2 business hours
This item is available online through Marketplace sellers.
-
SHIP THIS ITEM
Temporarily Out of Stock Online
Please check back later for updated availability.
This item is available online through Marketplace sellers.
Overview
Authored by three leading experts in computer science instruction—including 2005 SIGCSE award-winner Kim Bruce—this book reflects a fundamental rethinking of how Java and object-oriented languages are taught. To give students the "training wheels" they need to rapidly begin using sophisticated Java features, the authors introduce a complete objectoriented graphics library. Using it, students can immediately begin programming simple graphics and animations, and literally see the results of their code. Students learn core language features as they're ready—and master powerful features such as concurrency far sooner than in conventional treatments.
This book's object-first approach, content, and features have been systematically proven at many of the world's leading universities, four-year colleges, and two-year institutions. Features include:
- Strong focus on event-driven programming with GUI components: a paradigm students recognize from their experience with point-and-click environments
- Early introductions to simple concurrency and threads, focusing on correct programming style
- Thorough, step-by-step coverage of all typical introductory material, including arrays, strings, recursion, files, sorting, searching, inheritance, and more
- An exceptionally effective approach to teaching exceptions
- A practical primer on the fundamentals of object-oriented design
- Embedded chapter exercises for assessing progress
- End-of-chapter review exercises and programming problems that reinforce key concepts and techniques
- Appendix explaining how to navigate and read any Java API
Product Details
ISBN-13: | 9780131424159 |
---|---|
Publisher: | Pearson Education |
Publication date: | 07/29/2005 |
Edition description: | New Edition |
Pages: | 720 |
Product dimensions: | 7.15(w) x 9.25(h) x 1.60(d) |
About the Author
Andrea Pohoreckyj Danyluk, Associate Professor of Computer Science at Williams, holds a Ph.D. from Columbia, and has contributed to the ACM/IEEE Task Force' on Computing Curricula.
Thomas P. Murtagh, Professor of Computer Science at Williams, holds a Ph.D. from Cornell and formerly taught at Purdue. He has published on CS pedagogy and curricular design, and on topics ranging from compiler optimization to operating system design.
Read an Excerpt
A Java-based introductory course provides new challenges to instructors and students. While Java is simpler than C++, the fact that Java is an object-oriented language with a significant number of standard libraries adds both new complexities and opportunities.
This introductory computer science text provides a new approach to teaching programming in Java that combines several interesting features:
- an objects-first approach to programming,
- the intensive use of object-oriented graphics,
- the use of concurrency early,
- the use of event-driven programming from the beginning.
At first glance, this list of topics might seem overwhelming for an introductory text, but the synergy of these features results in a surprisingly effective introduction to programming using Java, especially when presented with the help of a library, objectdraw, that we have developed.
Target Audience
The primary target audiences for this text are first-year computer science majors, other college and university students interested in programming, and students taking high school advanced placement courses in computer science. In particular, this text covers the AP A exam material.
For the Student Mysterious Buzzwords
We began this preface by listing some of the special features of this text that we find particularly exciting. Specifically, we said that the text provides:
- an objects-first approach to programming,
- the intensive use of object-oriented graphics,
- the use of concurrency early,
- the use of event-driven programming from the beginning.
At this point you're probably wondering what all of these buzzwords mean.
Java is an example of an object-oriented programming language. Just as there are many different types of spoken languages, there are many different computer programming languages. The object-oriented languages are simply one class of languages. Because programming languages differ from each other in many ways, it stands to reason that they should not all be taught in the same way. Since Java is object oriented, we have aimed to present it in a manner that is appropriate for that language paradigm.
From the beginning of the text, you will learn how to write programs that involve simple graphicsrectangles, ovals, and lines, for example. You will even learn to write programs that create graphical animations, using mechanisms for concurrency. We find that both students and instructors enjoy writing programs that involve interesting, albeit simple, graphics. In addition to being fun, graphics are very concrete. When a program involves drawing and manipulating graphical objects in a window, you can actually see what the program is doing. We find this helpful for the beginning programmer.
We also introduce event-driven programming early. While you probably haven't heard the term "event-driven programming", you're almost certainly familiar with it. If you've interacted with a computer by pulling down menus, clicking on icons, and dragging items with a mouse, you've interacted with event-driven programs. The programs you will learn to write will allow a user to interact with them through mouse movements, buttons, scroll bars, and so on.
How to Read This Book
Practice is an extremely important component of learning to program. Therefore, we have provided many opportunities for you to practice as you read this text. Each chapter contains embedded exercises that will allow you to check your understanding. Read with a pencil and paper beside you, so that you can do these short exercises as you go along. In addition, at the end of each chapter you will find chapter review exercises as well as programming problems. Working through the review exercises will help you determine whether you have understood the major concepts introduced in the chapter. Once you feel comfortable with these, try the programming problems. The more you do, the better you'll know Java.
For the Instructor Special Features of This Text A Graphics Library for the Objects-First Approach
We have adopted the use of graphics for our first examples and have constructed a truly object-oriented library of graphics classes. There are several reasons we believe that graphics provide a good setting for introducing object-oriented programming.
First, graphics are good exemplars of objects. Graphics classes (e.g., framed and filled rectangles and ovals) provide good examples of objects because they have state (their location and dimensions) and a useful collection of methods that go well beyond methods that just get and set instance variables. Second, the graphics classes in our objectdraw library provide excellent feedback for novice programmers. When a graphics object is created, it appears on the screen immediately. When a graphics object in our library is moved or resized, the picture on the screen changes immediately. As a result, if a program contains a logical error, that error is immediately visible on the screen. Third, graphics provide motivating examples. With graphics, very simple programs can become much more interesting to students. Moreover, once animations are introduced, it is easy to provide fun and interesting examples well before the introduction of arrays. Finally, graphics persist in the course. Rather than introducing a set of example objects and then discarding it, an instructor can use the graphics library throughout the course.
Our objectdraw library not only provides the graphics classes, it also provides a WindowController class that extends JApplet by installing a DrawingCanvas in the center of the window. DrawingCanvas is an extension of JComponent that keeps track of the objects on the canvas and redraws them whenever necessary. This reduces the complexity of using graphics for novice programmers.
Event-Driven Programming
Some authors have argued for an event-driven approach in an introductory course because "real" programs that students use every day operate in an event-driven way. In students' use of computers they rarely see programs that respond to line-by-line text input. Thus event-driven programming is more motivating for students.
We believe there are several other pedagogically important advantages to an event-driven approach in an introductory course. One very important advantage is that students get experience writing methods from the beginning. Moreover, the methods tend to be very short.
In our library, we provide an environment in which novices learn to program by defining simple mouse-event-handling methods. For example, our onMouseDrag method is similar to standard Java's mouseDragged method, except that it has a simpler parameter. Because it is called repeatedly while the mouse is being dragged, very interesting programs can be constructed without using loops. This use of event-driven programming allows us to postpone the discussion of loops until after we discuss the definition of classes, while still presenting interesting examples to students.
Students get experience writing methods and using parameters by writing methods with fixed names and numbers of parameters, simplifying the introduction of these concepts. For example, all of our mouse-handling methods take a single Location parameter representing where the mouse is when the event occurs. Students become accustomed to using these formal parameters inside the associated method bodies. At the same time, students use actual parameters in the graphics commands.
This experience in writing event-handling methods with well-specified names and signatures, as well as the experience of writing code to send messages with actual parameters to graphic objects, makes the transition to designing and writing classes and their methods easier for students. Students still need to work to understand the "how" and "why" of parameter passing, but they will have seen and written many examples. That helps students in writing and understanding their own classes.
Objects-First
The combination of graphics and event-driven programming supports our objects-first approach. Students see example programs using objects from the graphics library starting from the first chapter of the text. Examples contain code to create new graphics objects and send messages to them. Moreover, the programs are extensions of the WindowController class.
Because the WindowController class of our library is an extension of Java's JApplet class, students need not begin with the static main method, and then have to learn about the differences between static and nonstatic methods. Instead they write instance methods that respond to mouse events. Thus students are introduced to using objects and writing their own methods from the first chapter of the text.
In the sixth chapter, students learn how to write their own classes. This chapter occurs before the introduction of loops, and just after the introduction of conditional statements. Our approach using event-driven programming allows us to construct and use interesting classes at this early point in the text.
Concurrency Early
We found that when examples are properly chosen to avoid race conditions, concurrent programming is conceptually easy for students to understand. After all, the world is concurrent, so there is nothing unnatural to students in having several threads executing concurrently. Moreover, many applications are much easier to program using concurrency rather than as a single thread.
We have provided a class ActiveObject in our library that supports using and managing threads. From a student's point of view, the primary difference between the ActiveObject class that we provide and the built-in Thread class is that we provide a variant of the sleep method that does not throw exceptions. As a result we are able to introduce concurrency in Chapter 9 of the text, before our discussion of exceptions. Behind the scenes, we also manage threads so that when a program (or applet in a web page) terminates, all threads will be terminated gracefully.
Why Introduce a Library?
We have chosen to introduce a library to support our approach, because it reduces syntactic and conceptual complexity early in the text. While we depend on the library early, it is not our intention to teach a different style of programming than that normally supported by Java. Our philosophy is to provide support early, but to also teach students the "right" way to program in Java.
A possible obstacle to using event-driven programming early in Java is the number of language and library features that must be introduced in order to handle events. For example, one would have to introduce listeners, interfaces, Java events, and so on. Moreover, if a class is to implement, for example, MouseListener, then it must implement all of the mouse-listening methods, even if only one is needed in the program.
Our library reduces this complexity as the WindowController class from the library implements both of the mouse listener interfaces. It also provides event-handling methods that take the Location of the mouse, rather than a more general MouseEvent. The advantage of getting a Location (a library type representing a point on the screen, but using doubles rather than integers) is that the useful information is immediately available, rather than requiring the programmer to extract it first. Finally, with our library, students only need to write the event-handling methods that they actually use in their program.
In Chapter 11 we teach students about standard Java GUI components. In conjunction with this we also teach students the standard Java event model. Students learn to associate listeners with user interface components and to write methods to handle the generated events. Thus they do leak how to program without using our library, but at a time when they are better equipped to understand the needed concepts.
As we noted above, introducing threads without using our library would require a discussion of exceptions before being able to pause a thread. Moreover, the exception that must be handled with the sleep method is a very bad first example of exceptions, as there is generally not much to do to handle it. Because we think exceptions can be better motivated later in the course (for example, in discussing I/O) and because they involve the complexity of inheritance and subtyping, we designed our library to enable us to postpone the discussion of exceptions. We do not use the library as an excuse to avoid teaching key components of Java. Instead we use it to provide a more pedagogically sound approach to presenting the various concepts introduced.
Supplementary Materials for Instructors
Supplementary materials are available on-line for instructors at http://eventfuljava.cs.williams.edu. These materials include the objectdraw library, a rich collection of sample programs and laboratory assignments that use the library and that are coordinated with the text, and detailed lecture notes.
The sample programs include those already in the text as well as a large collection of additional examples. The supplementary examples are rich and varied and add a great deal to the overall presentation of the material in the text. In some cases the additional programs stress (and therefore reinforce) certain dependencies. (This is in direct contrast to the way in which we wrote the text, where we attempted to minimize dependencies wherever possible.) Many of the additional examples involve Active0bjects and, more specifically, animation. These do not always serve as the best types of examples in a text, as a book is a static medium, but they can be used extremely effectively by the instructor in a classroom or laboratory setting.
Flexibility for the Instructor end Student
In this text we have aimed to provide maximum flexibility for the reader. We expect the reader to cover the core introductory material in Chapters 1, 2, 3, 4, 6, 7, and 9. These chapters introduce the objectdraw library, conditionals, classes, while loops, and concurrency. Chapters 5 and 8 provide additional details about the topics introduced in Chapters 3 and 6. As well, they introduce strings and the topics of declaration and scope. While they are important, these chapters can be covered later, if desired.
Optional sections in all of the chapters, marked with an asterisk (*), can also be skipped.
The remainder of the text presents topics in the order in which we cover them in our course. We have found this order to work very well. However, as remarked earlier one of our goals was to avoid topic dependencies as much as possible, so that instructors could tailor their courses as appropriate for their students and their institutions. Figure 0.1 shows the ways in which Chapters 10-19 depend upon each other. Note that, in particular, recursion (and recursive data structures) and arrays can be presented in any order. It is also possible to cover inheritance before either recursion or arrays.
In addition to the dependencies shown in Figure 0.1, it is important to note that later chapters assume knowledge of GUI to a limited extent. In particular, examples in these later chapters make use of JTextFields and the setText and getText methods.
Chapter 20. "Searching and Sorting", is an advanced topic and assumes knowledge of arrays and recursion. The sections on search do, however, give both iterative and recursive versions of the algorithms presented.
The second half of Chapter 21, "Introduction to Object-Oriented Design", assumes that students are familiar with both recursion and arrays. However, the first half of that chapter assumes nothing beyond familiarity with the core chapters, 1-9. If desired, the reader could work through the design unit in two stages, covering the first half after the core introductory material and the second half after the more advanced topics.
Finally, it is important to note that this text is not meant to be a complete reference on the Java programming language. We have strived to present the elements of the language at a level that is appropriate for a beginner. Some of the chapters in the second half of the text, for example, provide introductions to important concepts, without necessarily providing details on the level that an advanced student might require. Our goal is to give students a firm footing, with the expectation that they will develop a deeper and more complete understanding of the language later, as they gain more experience.
Additional Practical Information
We have included a great deal of additional useful material in several appendices to the book. The first appendix provides style guidelines for programming. While there are fairly standard conventions followed by Java programmers, some issues of style are obviously subjective. Students should note that their instructors might provide their own guidelines.
The next appendix provides a summary of the classes and methods available in the objectdraw library. In the third appendix, we take the reader through the process of navigating the documentation for Java APIs. An API (Application Programming Interface) specifies the details a programmer must know to use the resources a library provides. We go through parts of the API for the objectdraw library, but as the documentation for many APIs follows a standard format, the reader could then be able to read other API documentation as well.
The transition from our library to standard Java is quite straightforward. The final appendix summarizes for the reader the standard Java equivalents for many of the features in the library.
Table of Contents
(NOTE: Most chapters conclude with Summary, Chapter Review Problems, and Programming Problems.)1. What is Programming Anyway? 2. What’s in a name? 3. Working with Numbers.
4. Making Choices.
5. Primitive Types, Operators, and Strings.
6. Classes.
7. Control Structures. 8. Declarations and Scope. 9. Active Objects. 10. Interfaces.
11. Graphical User Interfaces in Java.
12. Recursion.
13. General loops in Java.
14. Arrays.
15. Multidimensional Arrays.
16. Strings and Characters.
17. Inheritance. 18. Exceptions. 19. Streams 481. 20. Searching and Sorting.
21. Introduction to Object-Oriented Design.
Appendix A. Objectdraw API Summary. Appendix B. Programming without objectdraw. Appendix C. Creating runnable applets and applications. Index.
Preface
A Java-based introductory course provides new challenges to instructors and students. While Java is simpler than C++, the fact that Java is an object-oriented language with a significant number of standard libraries adds both new complexities and opportunities.
This introductory computer science text provides a new approach to teaching programming in Java that combines several interesting features:
- an objects-first approach to programming,
- the intensive use of object-oriented graphics,
- the use of concurrency early,
- the use of event-driven programming from the beginning.
At first glance, this list of topics might seem overwhelming for an introductory text, but the synergy of these features results in a surprisingly effective introduction to programming using Java, especially when presented with the help of a library, objectdraw, that we have developed.
Target AudienceThe primary target audiences for this text are first-year computer science majors, other college and university students interested in programming, and students taking high school advanced placement courses in computer science. In particular, this text covers the AP A exam material.
For the Student Mysterious BuzzwordsWe began this preface by listing some of the special features of this text that we find particularly exciting. Specifically, we said that the text provides:
- an objects-first approach to programming,
- the intensive use of object-oriented graphics,
- the use of concurrency early,
- the use of event-driven programming from the beginning.
At this point you're probably wondering what all of these buzzwords mean.
Java is an example of an object-oriented programming language. Just as there are many different types of spoken languages, there are many different computer programming languages. The object-oriented languages are simply one class of languages. Because programming languages differ from each other in many ways, it stands to reason that they should not all be taught in the same way. Since Java is object oriented, we have aimed to present it in a manner that is appropriate for that language paradigm.
From the beginning of the text, you will learn how to write programs that involve simple graphicsrectangles, ovals, and lines, for example. You will even learn to write programs that create graphical animations, using mechanisms for concurrency. We find that both students and instructors enjoy writing programs that involve interesting, albeit simple, graphics. In addition to being fun, graphics are very concrete. When a program involves drawing and manipulating graphical objects in a window, you can actually see what the program is doing. We find this helpful for the beginning programmer.
We also introduce event-driven programming early. While you probably haven't heard the term 'event-driven programming', you're almost certainly familiar with it. If you've interacted with a computer by pulling down menus, clicking on icons, and dragging items with a mouse, you've interacted with event-driven programs. The programs you will learn to write will allow a user to interact with them through mouse movements, buttons, scroll bars, and so on.
How to Read This BookPractice is an extremely important component of learning to program. Therefore, we have provided many opportunities for you to practice as you read this text. Each chapter contains embedded exercises that will allow you to check your understanding. Read with a pencil and paper beside you, so that you can do these short exercises as you go along. In addition, at the end of each chapter you will find chapter review exercises as well as programming problems. Working through the review exercises will help you determine whether you have understood the major concepts introduced in the chapter. Once you feel comfortable with these, try the programming problems. The more you do, the better you'll know Java.
For the Instructor Special Features of This Text A Graphics Library for the Objects-First ApproachWe have adopted the use of graphics for our first examples and have constructed a truly object-oriented library of graphics classes. There are several reasons we believe that graphics provide a good setting for introducing object-oriented programming.
First, graphics are good exemplars of objects. Graphics classes (e.g., framed and filled rectangles and ovals) provide good examples of objects because they have state (their location and dimensions) and a useful collection of methods that go well beyond methods that just get and set instance variables. Second, the graphics classes in our objectdraw library provide excellent feedback for novice programmers. When a graphics object is created, it appears on the screen immediately. When a graphics object in our library is moved or resized, the picture on the screen changes immediately. As a result, if a program contains a logical error, that error is immediately visible on the screen. Third, graphics provide motivating examples. With graphics, very simple programs can become much more interesting to students. Moreover, once animations are introduced, it is easy to provide fun and interesting examples well before the introduction of arrays. Finally, graphics persist in the course. Rather than introducing a set of example objects and then discarding it, an instructor can use the graphics library throughout the course.
Our objectdraw library not only provides the graphics classes, it also provides a WindowController class that extends JApplet by installing a DrawingCanvas in the center of the window. DrawingCanvas is an extension of JComponent that keeps track of the objects on the canvas and redraws them whenever necessary. This reduces the complexity of using graphics for novice programmers.
Event-Driven ProgrammingSome authors have argued for an event-driven approach in an introductory course because 'real' programs that students use every day operate in an event-driven way. In students' use of computers they rarely see programs that respond to line-by-line text input. Thus event-driven programming is more motivating for students.
We believe there are several other pedagogically important advantages to an event-driven approach in an introductory course. One very important advantage is that students get experience writing methods from the beginning. Moreover, the methods tend to be very short.
In our library, we provide an environment in which novices learn to program by defining simple mouse-event-handling methods. For example, our onMouseDrag method is similar to standard Java's mouseDragged method, except that it has a simpler parameter. Because it is called repeatedly while the mouse is being dragged, very interesting programs can be constructed without using loops. This use of event-driven programming allows us to postpone the discussion of loops until after we discuss the definition of classes, while still presenting interesting examples to students.
Students get experience writing methods and using parameters by writing methods with fixed names and numbers of parameters, simplifying the introduction of these concepts. For example, all of our mouse-handling methods take a single Location parameter representing where the mouse is when the event occurs. Students become accustomed to using these formal parameters inside the associated method bodies. At the same time, students use actual parameters in the graphics commands.
This experience in writing event-handling methods with well-specified names and signatures, as well as the experience of writing code to send messages with actual parameters to graphic objects, makes the transition to designing and writing classes and their methods easier for students. Students still need to work to understand the 'how' and 'why' of parameter passing, but they will have seen and written many examples. That helps students in writing and understanding their own classes.
Objects-FirstThe combination of graphics and event-driven programming supports our objects-first approach. Students see example programs using objects from the graphics library starting from the first chapter of the text. Examples contain code to create new graphics objects and send messages to them. Moreover, the programs are extensions of the WindowController class.
Because the WindowController class of our library is an extension of Java's JApplet class, students need not begin with the static main method, and then have to learn about the differences between static and nonstatic methods. Instead they write instance methods that respond to mouse events. Thus students are introduced to using objects and writing their own methods from the first chapter of the text.
In the sixth chapter, students learn how to write their own classes. This chapter occurs before the introduction of loops, and just after the introduction of conditional statements. Our approach using event-driven programming allows us to construct and use interesting classes at this early point in the text.
Concurrency EarlyWe found that when examples are properly chosen to avoid race conditions, concurrent programming is conceptually easy for students to understand. After all, the world is concurrent, so there is nothing unnatural to students in having several threads executing concurrently. Moreover, many applications are much easier to program using concurrency rather than as a single thread.
We have provided a class ActiveObject in our library that supports using and managing threads. From a student's point of view, the primary difference between the ActiveObject class that we provide and the built-in Thread class is that we provide a variant of the sleep method that does not throw exceptions. As a result we are able to introduce concurrency in Chapter 9 of the text, before our discussion of exceptions. Behind the scenes, we also manage threads so that when a program (or applet in a web page) terminates, all threads will be terminated gracefully.
Why Introduce a Library?We have chosen to introduce a library to support our approach, because it reduces syntactic and conceptual complexity early in the text. While we depend on the library early, it is not our intention to teach a different style of programming than that normally supported by Java. Our philosophy is to provide support early, but to also teach students the 'right' way to program in Java.
A possible obstacle to using event-driven programming early in Java is the number of language and library features that must be introduced in order to handle events. For example, one would have to introduce listeners, interfaces, Java events, and so on. Moreover, if a class is to implement, for example, MouseListener, then it must implement all of the mouse-listening methods, even if only one is needed in the program.
Our library reduces this complexity as the WindowController class from the library implements both of the mouse listener interfaces. It also provides event-handling methods that take the Location of the mouse, rather than a more general MouseEvent. The advantage of getting a Location (a library type representing a point on the screen, but using doubles rather than integers) is that the useful information is immediately available, rather than requiring the programmer to extract it first. Finally, with our library, students only need to write the event-handling methods that they actually use in their program.
In Chapter 11 we teach students about standard Java GUI components. In conjunction with this we also teach students the standard Java event model. Students learn to associate listeners with user interface components and to write methods to handle the generated events. Thus they do leak how to program without using our library, but at a time when they are better equipped to understand the needed concepts.
As we noted above, introducing threads without using our library would require a discussion of exceptions before being able to pause a thread. Moreover, the exception that must be handled with the sleep method is a very bad first example of exceptions, as there is generally not much to do to handle it. Because we think exceptions can be better motivated later in the course (for example, in discussing I/O) and because they involve the complexity of inheritance and subtyping, we designed our library to enable us to postpone the discussion of exceptions. We do not use the library as an excuse to avoid teaching key components of Java. Instead we use it to provide a more pedagogically sound approach to presenting the various concepts introduced.
Supplementary Materials for InstructorsSupplementary materials are available on-line for instructors at http://eventfuljava.cs.williams.edu. These materials include the objectdraw library, a rich collection of sample programs and laboratory assignments that use the library and that are coordinated with the text, and detailed lecture notes.
The sample programs include those already in the text as well as a large collection of additional examples. The supplementary examples are rich and varied and add a great deal to the overall presentation of the material in the text. In some cases the additional programs stress (and therefore reinforce) certain dependencies. (This is in direct contrast to the way in which we wrote the text, where we attempted to minimize dependencies wherever possible.) Many of the additional examples involve Active0bjects and, more specifically, animation. These do not always serve as the best types of examples in a text, as a book is a static medium, but they can be used extremely effectively by the instructor in a classroom or laboratory setting.
Flexibility for the Instructor end StudentIn this text we have aimed to provide maximum flexibility for the reader. We expect the reader to cover the core introductory material in Chapters 1, 2, 3, 4, 6, 7, and 9. These chapters introduce the objectdraw library, conditionals, classes, while loops, and concurrency. Chapters 5 and 8 provide additional details about the topics introduced in Chapters 3 and 6. As well, they introduce strings and the topics of declaration and scope. While they are important, these chapters can be covered later, if desired.
Optional sections in all of the chapters, marked with an asterisk (*), can also be skipped.
The remainder of the text presents topics in the order in which we cover them in our course. We have found this order to work very well. However, as remarked earlier one of our goals was to avoid topic dependencies as much as possible, so that instructors could tailor their courses as appropriate for their students and their institutions. Figure 0.1 shows the ways in which Chapters 10-19 depend upon each other. Note that, in particular, recursion (and recursive data structures) and arrays can be presented in any order. It is also possible to cover inheritance before either recursion or arrays.
In addition to the dependencies shown in Figure 0.1, it is important to note that later chapters assume knowledge of GUI to a limited extent. In particular, examples in these later chapters make use of JTextFields and the setText and getText methods.
Chapter 20. 'Searching and Sorting', is an advanced topic and assumes knowledge of arrays and recursion. The sections on search do, however, give both iterative and recursive versions of the algorithms presented.
The second half of Chapter 21, 'Introduction to Object-Oriented Design', assumes that students are familiar with both recursion and arrays. However, the first half of that chapter assumes nothing beyond familiarity with the core chapters, 1-9. If desired, the reader could work through the design unit in two stages, covering the first half after the core introductory material and the second half after the more advanced topics.
Finally, it is important to note that this text is not meant to be a complete reference on the Java programming language. We have strived to present the elements of the language at a level that is appropriate for a beginner. Some of the chapters in the second half of the text, for example, provide introductions to important concepts, without necessarily providing details on the level that an advanced student might require. Our goal is to give students a firm footing, with the expectation that they will develop a deeper and more complete understanding of the language later, as they gain more experience.
Additional Practical InformationWe have included a great deal of additional useful material in several appendices to the book. The first appendix provides style guidelines for programming. While there are fairly standard conventions followed by Java programmers, some issues of style are obviously subjective. Students should note that their instructors might provide their own guidelines.
The next appendix provides a summary of the classes and methods available in the objectdraw library. In the third appendix, we take the reader through the process of navigating the documentation for Java APIs. An API (Application Programming Interface) specifies the details a programmer must know to use the resources a library provides. We go through parts of the API for the objectdraw library, but as the documentation for many APIs follows a standard format, the reader could then be able to read other API documentation as well.
The transition from our library to standard Java is quite straightforward. The final appendix summarizes for the reader the standard Java equivalents for many of the features in the library.