The Java' Programming Language / Edition 4 available in Paperback

The Java' Programming Language / Edition 4
- ISBN-10:
- 0321349806
- ISBN-13:
- 9780321349804
- Pub. Date:
- 08/17/2005
- Publisher:
- Pearson Education
- ISBN-10:
- 0321349806
- ISBN-13:
- 9780321349804
- Pub. Date:
- 08/17/2005
- Publisher:
- Pearson Education

The Java' Programming Language / Edition 4
Buy New
$69.99Buy Used
$29.86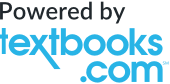
-
SHIP THIS ITEM— This item is available online through Marketplace sellers.
-
PICK UP IN STORE
Your local store may have stock of this item.
Available within 2 business hours
This item is available online through Marketplace sellers.
-
SHIP THIS ITEM
Temporarily Out of Stock Online
Please check back later for updated availability.
Overview
Direct from the creators of the Java™ programming language, the completely revised fourth edition of The Java™ Programming Language is an indispensable resource for novice and advanced programmers alike.
Developers around the world have used previous editions to quickly gain a deep understanding of the Java programming language, its design goals, and how to use it most effectively in real-world development. Now, Ken Arnold, James Gosling, and David Holmes have updated this classic to reflect the major enhancements in Java™ 2 Standard Edition 5.0 (J2SE™ 5.0).
The authors systematically cover most classes in Java’s main packages, java.lang.*, java.util, and java.io, presenting in-depth explanations of why these classes work as they do, with informative examples. Several new chapters and major sections have been added, and every chapter has been updated to reflect today’s best practices for building robust, efficient, and maintainable Java software.
Key changes in this edition include
- New chapters on generics, enums, and annotations, the most powerful new language features introduced in J2SE 5.0
- Changes to classes and methods throughout to reflect the addition of generics
- Major new sections on assertions and regular expressions
- Coverage of all the new language features, from autoboxing and variable argument methods to the enhanced for-loop and covariant return types
- Coverage of key new classes, such as Formatter and Scanner
The Java™ Programming Language, Fourth Edition, is the definitive tutorial introduction to the Java language and essential libraries and an indispensable reference for all programmers, including those with extensive experience. It brings together insights you can only get from the creators of Java: insights that will help you write software of exceptional quality.
Product Details
ISBN-13: | 9780321349804 |
---|---|
Publisher: | Pearson Education |
Publication date: | 08/17/2005 |
Series: | Java Series |
Edition description: | REV |
Pages: | 928 |
Product dimensions: | 7.40(w) x 9.25(h) x 1.55(d) |
About the Author
Ken Arnold, formerly senior engineer at Sun Microsystems Laboratories, is a leading expert in object-oriented design and implementation. He was one of the original architects of the Jini™ technology, and the lead engineer of Sun's JavaSpaces™ technology.
James Gosling is a Fellow and Chief Technology Officer of Sun's Developer Products group, the creator of the Java programming language, and one of the computer industry's most noted programmers. He is the 1996 recipient of Software Development's "Programming Excellence Award." He previously developed NeWS, Sun's network-extensible window system, and was a principal in the Andrew project at Carnegie Mellon University, where he earned a Ph.D. in computer science.
David Holmes is director of DLTeCH Pty Ltd, located in Brisbane, Australia. He specializes in synchronization and concurrency and was a member of the JSR-166 expert group that developed the new concurrency utilities. He is also a contributor to the update of the Real-Time Specification for Java, and has spent the past few years working on an implementation of that specification.
Read an Excerpt
Beautiful buildings are more than scientific. They are true organisms,spiritually conceived; works of art, using the best technology by inspiration rather than the idiosyncrasies of mere taste or any averaging by the committee mind.
Frank Lloyd Wright
The Java programming language has been warmly received by the world community of software developers and Internet content providers. Users of the Internet and World Wide Web benefit from access to secure, platform-independent applications that can come from anywhere on the Internet. Software developers who create applications in the Java programming language benefit by developing code only once, with no need to "port" their applications to every software and hardware platform.
For many, the language was known first as a tool to create applets for the World Wide Web. An applet is a mini-application that runs inside a Web page. An applet can perform tasks and interact with users on their browser pages without using resources from the Web server after being downloaded. Some applets may, of course, talk with the server to do their job, but that's their business.
The Java programming language is indeed valuable for distributed network environments like the Web. However, it goes well beyond this domain to provide a powerful general-purpose programming language suitable for building a variety of applications that either do not depend on network features or want them for different reasons. The ability to execute downloaded code on remote hosts in a secure manner is a critical requirement for many organizations.
Other groups use it as a general-purpose programminglanguage for projects in which machine independence is less important. Ease of programming and safety features help you quickly produce working code. Some common programming errors never occur because of features like garbage collection and type-safe references. Support for multithreading caters to modern network-based and graphical user interface-based applications that must attend to multiple tasks simultaneously, and the mechanisms of exception handling ease the task of dealing with error conditions. While the built-in tools are powerful, it is a simple language in which programmers can quickly become proficient.
The Java programming language is designed for maximum portability with as few implementation dependencies as possible. An int, for example, is a 32-bit signed two's-complement integer in all implementations, irrespective of the CPU architecture on which the program executes. Defining everything possible about the language and its runtime environment enables users to run compiled code anywhere and share code with anyone who has a Java runtime environment.About This Book
This book teaches the Java programming language to people who are familiar with basic programming concepts. It explains the language without being arduously formal or complete. This book is not an introduction to object-oriented programming, although some issues are covered to establish a common terminology. Other books in this series and much online documentation focus on applets, graphical interfaces, Web sites, databases, components, and other specific kinds of programming tasks. For other references, see "Further Reading" on page 755.
This fourth edition provides integrated coverage of the Java programming language as provided by the Java 2 Platform Standard Edition 5.0 and specified by the Java Language Specification, Third Edition. It also covers most of the classes in the main packages (java.lang, java.util, java.io) as implemented in the J2SE Development Kit 5.0 (more commonly known as JDK 5.0, or in the older nomenclature JDK 1.5.0).
If you have already read the third edition of this book, you will find some major changes, both in the language and the book, since the 1.3 release that the third edition covered. There are new chapters on generics, enums, and annotationsthe major new language features introduced in the 5.0 releaseand major new sections on assertions and regular expressions. Some existing material has been restructured to accommodate other changes and to improve the general flow of the textsuch as introducing the new boxing and unboxing conversions. But every single chapter has been updated in some way, whether it is a new language feature like variable argument methods; the new enhanced for loop construct; a new class such as Formatter for formatting text output; or changes to classes and methods caused by the addition of generics (such as the collections utilities and the reflection classes)change permeates this entire fourth edition.
The Java programming language shares many features common to most programming languages in use today. The language should look familiar to C and C++ programmers because it was designed with C and C++ constructs where the languages are similar. That said, this book is neither a comparative analysis nor a "bridge" tutorialno knowledge of C or C++ is assumed. C++ programmers, especially, may be as hindered by what they must unlearn as they are helped by their knowledge.
Chapter 1A Quick Tourgives a quick overview of the language. Programmers who are unfamiliar with object-oriented programming notions should read the quick tour, while programmers who are already familiar with object-oriented programming paradigms will find the quick tour a useful introduction to the object-oriented features of the language. The quick tour introduces some of the basic language features on which examples through the rest of the book are built.
Chapters 2 through 6 cover the object-oriented core features of the language, namely, class declarations that define components of a program, and objects manufactured according to class definitions. Chapter 2Classes and Objectsdescribes the basis of the language: classes. Chapter 3Extending Classesdescribes how an existing class can be extended, or subclassed, to create a new class with additional data and behavior. Chapter 4Interfacesdescribes how to declare interface types that are abstract descriptions of behavior that provide maximum flexibility for class designers and implementors. Chapter 5Nested Classes and Interfacesdescribes how classes and interfaces can be declared inside other classes and interfaces, and the benefits that provides. Finally, Chapter 6Enumeration Typescovers the definition and use of type-safe enumeration constants.
Chapters 7 through 10 cover standard constructs common to most languages. Chapter 7Tokens, Values, and Variablesdescribes the tokens of the language from which statements are constructed, the types defined by the language and their allowed values, and the variables that store data in objects, arrays, or locally within methods. Chapter 8Primitives as Typesexplores the relationship between the primitive types and objects of their corresponding wrapper classes, and how boxing and unboxing can transparently convert between them. Chapter 9Operators and Expressionsdescribes the basic operators of the language, how these operators are used to build expressions, and how expressions are evaluated. Chapter 10Control Flowdescribes how control statements direct the order of statement execution.
Chapter 11Generic Typesdescribes generic types: how they are written and used, their power, and their limitations.
Chapter 12Exceptions and Assertionsdescribes the language's powerful error-handling capabilities, and the use of assertions to validate the expected behavior of code.
Chapter 13Strings and Regular Expressionsdescribes the built-in language and runtime support for String objects, the underlying character set support, and the powerful utilities for regular expression matching.
Chapter 14Threadsexplains the language's view of multithreading. Many applications, such as graphical interface-based software, must attend to multiple tasks simultaneously. These tasks must cooperate to behave correctly, and threads meet the needs of cooperative multitasking.
Chapter 15Annotationsdescribes the annotation types used to document some of the extra-linguistic properties of classes and method.
Chapter 16Reflectiondescribes the runtime type introspection mechanism and how you can construct and manipulate objects of unknown type dynamically at runtime.
Chapter 17Garbage Collection and Memorytalks about garbage collection, finalization, and lower-strength reference objects.
Chapter 18Packagesdescribes how you can group collections of classes and interfaces into separate packages.
Chapter 19Documentation Commentsshows how to write reference documentation in comments.
Chapters 20 through 24 cover the main packages. Chapter 20The I/O Packagedescribes the input/output system, which is based on streams. Chapter 21Collectionscovers the collection or container classes such as sets and lists. Chapter 22Miscellaneous Utilitiescovers the rest of the utility classes such as bit sets, formatted output, text scanning, and random number generation. Chapter 23System Programmingleads you through the system classes that provide access to features of the underlying platform. Chapter 24Internationalization and Localizationcovers some of the tools used to create programs that can run in many linguistic and cultural environments.
Chapter 25Standard Packagesbriefly explores the packages that are part of the standard platform, giving overviews of those packages not covered in more detail in this book.
Appendix AApplication Evolutionlooks at some of the issues involved in dealing with the evolution of applications and the Java platform, and the impact this has on some of the new language features.
Appendix BUseful Tableshas tables of information that you may find useful for quick reference.
Finally, Further Reading lists works that may be interesting for further reading on complete details, object orientation, programming with threads, software design, and other topics.Examples and Documentation
All the code examples in the text have been compiled and run on the latest version of the language available at the time the book was written, which was the JDK 1.5.0_02 product version. Only supported features are covereddeprecated types, methods, and fields are ignored except when unavoidable or when knowledge of the past is necessary to understand the present. We have also covered issues beyond writing programs that simply compile. Part of learning a language is to learn to use it well. For this reason, we have tried to show principles of good programming style and design.
In a few places we refer to online documentation. Development environments provide a way to automatically generate documentation (usually HTML documents) for a compiled class from its documentation comments. This documentation is normally viewed with a Web browser.
Results! Why, man, I have gotten a lot of results. I know several thousand things that won't work.
Thomas Edison
Table of Contents
Preface xxi
Chapter 1: A Quick Tour 1
1.1 Getting Started 1
1.2 Variables 3
1.3 Comments in Code 6
1.4 Named Constants 7
1.5 Unicode Characters 8
1.6 Flow of Control 9
1.7 Classes and Objects 12
1.8 Methods and Parameters 15
1.9 Arrays 18
1.10 String Objects 21
1.11 Extending a Class 24
1.12 Interfaces 27
1.13 Generic Types 29
1.14 Exceptions 32
1.15 Annotations 35
1.16 Packages 36
1.17 The Java Platform 38
1.18 Other Topics Briefly Noted 39
Chapter 2: Classes and Objects 41
2.1 A Simple Class 42
2.2 Fields 44
2.3 Access Control 47
2.4 Creating Objects 49
2.5 Construction and Initialization 50
2.6 Methods 56
2.7 this
68
2.8 Overloading Methods 69
2.9 Importing Static Member Names 71
2.10 The main
Method 73
2.11 Native Methods 74
Chapter 3: Extending Classes 75
3.1 An Extended Class 76
3.2 Constructors in Extended Classes 80
3.3 Inheriting and Redefining Members 84
3.4 Type Compatibility and Conversion 90
3.5 What protected
Really Means 93
3.6 Marking Methods and Classes final
96
3.7 Abstract Classes and Methods 97
3.8 The Object
Class 99
3.9 Cloning Objects 101
3.10 Extending Classes: How and When 107
3.11 Designing a Class to Be Extended 108
3.12 Single Inheritance versus Multiple Inheritance 114
Chapter 4: Interfaces 117
4.1 A Simple Interface Example 118
4.2 Interface Declarations 120
4.3 Extending Interfaces 122
4.4 Working with Interfaces 126
4.5 Marker Interfaces 130
4.6 When to Use Interfaces 131
Chapter 5: Nested Classes and Interfaces 133
5.1 Static Nested Types 133
5.2 Inner Classes 136
5.3 Local Inner Classes 142
5.4 Anonymous Inner Classes 144
5.5 Inheriting Nested Types 146
5.6 Nesting in Interfaces 148
5.7 Implementation of Nested Types 149
Chapter 6: Enumeration Types 151
6.1 A Simple Enum Example 151
6.2 Enum Declarations 152
6.3 Enum Constant Declarations 154
6.4 java.lang.Enum
159
6.5 To Enum or Not 160
Chapter 7: Tokens, Values, and Variables 161
7.1 Lexical Elements 161
7.2 Types and Literals 166
7.3 Variables 169
7.4 Array Variables 173
7.5 The Meanings of Names 178
Chapter 8: Primitives as Types 183
8.1 Common Fields and Methods 184
8.2 Void
187
8.3 Boolean
187
8.4 Number
188
8.5 Character
192
8.6 Boxing Conversions 198
Chapter 9: Operators and Expressions 201
9.1 Arithmetic Operations 201
9.2 General Operators 204
9.3 Expressions 214
9.4 Type Conversions 216
9.5 Operator Precedence and Associativity 221
9.6 Member Access 223
Chapter 10: Control Flow 229
10.1 Statements and Blocks 229
10.2 if-else
230
10.3 switch
232
10.4 while
and do-while
235
10.5 for
236
10.6 Labels 241
10.7 break
241
10.8 continue
244
10.9 return
245
10.10 What, No goto
? 246
Chapter 11: Generic Types 247
11.1 Generic Type Declarations 250
11.2 Working with Generic Types 256
11.3 Generic Methods and Constructors 260
11.4 Wildcard Capture 264
11.5 Under the Hood: Erasure and Raw Types 267
11.6 Finding the Right Method--Revisited 272
11.7 Class Extension and Generic Types 276
Chapter 12: Exceptions and Assertions 279
12.1 Creating Exception Types 280
12.2 throw
282
12.3 The throws
Clause 283
12.4 try
, catch
, and finally
286
12.5 Exception Chaining 291
12.6 Stack Traces 294
12.7 When to Use Exceptions 294
12.8 Assertions 296
12.9 When to Use Assertions 297
12.10 Turning Assertions On and Off 300
Chapter 13: Strings and Regular Expressions 305
13.1 Character Sequences 305
13.2 The String
Class 306
13.3 Regular Expression Matching 321
13.4 The StringBuilder
Class 330
13.5 Working with UTF-16 336
Chapter 14: Threads 337
14.1 Creating Threads 339
14.2 Using Runnable
341
14.3 Synchronization 345
14.4 wait
, notifyAll
, and notify
354
14.5 Details of Waiting and Notification 357
14.6 Thread Scheduling 358
14.7 Deadlocks 362
14.8 Ending Thread Execution 365
14.9 Ending Application Execution 369
14.10 The Memory Model: Synchronization and volatile
370
14.11 Thread Management, Security, and ThreadGroup
375
14.12 Threads and Exceptions 379
14.13 ThreadLocal
Variables 382
14.14 Debugging Threads 384
Chapter 15: Annotations 387
15.1 A Simple Annotation Example 388
15.2 Annotation Types 389
15.3 Annotating Elements 392
15.4 Restricting Annotation Applicability 393
15.5 Retention Policies 395
15.6 Working with Annotations 395
Chapter 16: Reflection 397
16.1 The Class
Class 399
16.2 Annotation Queries 414
16.3 The Modifier
Class 416
16.4 The Member classes 416
16.5 Access Checking and AccessibleObject
417
16.6 The Field
Class 418
16.7 The Method
Class 420
16.8 Creating New Objects and the Constructor
Class 423
16.9 Generic Type Inspection 426
16.10 Arrays 429
16.11 Packages 432
16.12 The Proxy
Class 432
16.13 Loading Classes 435
16.14 Controlling Assertions at Runtime 444
Chapter 17: Garbage Collection and Memory 447
17.1 Garbage Collection 447
17.2 A Simple Model 448
17.3 Finalization 449
17.4 Interacting with the Garbage Collector 452
17.5 Reachability States and Reference Objects 454
Chapter 18: Packages 467
18.1 Package Naming 468
18.2 Type Imports 469
18.3 Package Access 471
18.4 Package Contents 475
18.5 Package Annotations 476
18.6 Package Objects and Specifications 477
Chapter 19: Documentation Comments 481
19.1 The Anatomy of a Doc Comment 482
19.2 Tags 483
19.3 Inheriting Method Documentation Comments 489
19.4 A Simple Example 491
19.5 External Conventions 496
19.6 Notes on Usage 497
Chapter 20: The I/O Package 499
20.1 Streams Overview 500
20.2 Byte Streams 501
20.3 Character Streams 507
20.4 InputStreamReader
and OutputStreamWriter
512
20.5 A Quick Tour of the Stream Classes 514
20.6 The Data Byte Streams 537
20.7 Working with Files 540
20.8 Object Serialization 549
20.9 The IOException
Classes 563
20.10 A Taste of New I/O 565
Chapter 21: Collections 567
21.1 Collections 567
21.2 Iteration 571
21.3 Ordering with Comparable
and Comparator
574
21.4 The Collection
Interface 575
21.5 Set
and SortedSet
577
21.6 List
580
21.7 Queue
585
21.8 Map
and SortedMap
587
21.9 enum
Collections 594
21.10 Wrapped Collections and the Collections
Class 597
21.11 Synchronized Wrappers and Concurrent Collections 602
21.12 The Arrays
Utility Class 607
21.13 Writing Iterator Implementations 609
21.14 Writing Collection Implementations 611
21.15 The Legacy Collection Types 616
21.16 Properties
620
Chapter 22: Miscellaneous Utilities 623
22.1 Formatter
624
22.2 BitSet
632
22.3 Observer/Observable
635
22.4 Random
639
22.5 Scanner
641
22.6 StringTokenizer
651
22.7 Timer
and TimerTask
653
22.8 UUID
656
22.9 Math
and StrictMath
657
Chapter 23: System Programming 661
23.1 The System
Class 662
23.2 Creating Processes 666
23.3 Shutdown 672
23.4 The Rest of Runtime
675
23.5 Security 677
Chapter 24: Internationalization and Localization 685
24.1 Locale 686
24.2 Resource Bundles 688
24.3 Currency 694
24.4 Time, Dates, and Calendars 695
24.5 Formatting and Parsing Dates and Times 703
24.6 Internationalization and Localization for Text 708
Chapter 25: Standard Packages 715
25.1 java.awt
--The Abstract Window Toolkit 717
25.2 java.applet
--Applets 720
25.3 java.beans
--Components 721
25.4 java.math
--Mathematics 722
25.5 java.net
--The Network 724
25.6 java.rmi
--Remote Method Invocation 727
25.7 java.security
and Related Packages--Security Tools 732
25.8 java.sql
--Relational Database Access 732
25.9 Utility Subpackages 733
25.10 javax.*
--Standard Extensions 737
25.11 javax.accessibility
--Accessibility for GUIs 737
25.12 javax.naming
--Directory and Naming Services 738
25.13 javax.sound
--Sound Manipulation 739
25.14 javax.swing
--Swing GUI Components 740
25.15 org.omg.CORBA
--CORBA APIs 740
Appendix A: Application Evolution 741
A.1 Language, Library, and Virtual Machine Versions 741
A.2 Dealing with Multiple Dialects 743
A.3 Generics: Reification, Erasure, and Raw Types 744
Appendix B: Useful Tables 749
Further Reading 755
Index 761
Preface
Beautiful buildings are more than scientific. They are true organisms,spiritually conceived; works of art, using the best technology by inspiration rather than the idiosyncrasies of mere taste or any averaging by the committee mind.
Frank Lloyd Wright
The Java programming language has been warmly received by the world community of software developers and Internet content providers. Users of the Internet and World Wide Web benefit from access to secure, platform-independent applications that can come from anywhere on the Internet. Software developers who create applications in the Java programming language benefit by developing code only once, with no need to “port” their applications to every software and hardware platform.
For many, the language was known first as a tool to create applets for the World Wide Web. An applet is a mini-application that runs inside a Web page. An applet can perform tasks and interact with users on their browser pages without using resources from the Web server after being downloaded. Some applets may, of course, talk with the server to do their job, but that’s their business.
The Java programming language is indeed valuable for distributed network environments like the Web. However, it goes well beyond this domain to provide a powerful general-purpose programming language suitable for building a variety of applications that either do not depend on network features or want them for different reasons. The ability to execute downloaded code on remote hosts in a secure manner is a critical requirement for many organizations.
Other groups use it as a general-purpose programming language for projects in which machine independence is less important. Ease of programming and safety features help you quickly produce working code. Some common programming errors never occur because of features like garbage collection and type-safe references. Support for multithreading caters to modern network-based and graphical user interface-based applications that must attend to multiple tasks simultaneously, and the mechanisms of exception handling ease the task of dealing with error conditions. While the built-in tools are powerful, it is a simple language in which programmers can quickly become proficient.
The Java programming language is designed for maximum portability with as few implementation dependencies as possible. An int, for example, is a 32-bit signed two’s-complement integer in all implementations, irrespective of the CPU architecture on which the program executes. Defining everything possible about the language and its runtime environment enables users to run compiled code anywhere and share code with anyone who has a Java runtime environment.
About This BookThis book teaches the Java programming language to people who are familiar with basic programming concepts. It explains the language without being arduously formal or complete. This book is not an introduction to object-oriented programming, although some issues are covered to establish a common terminology. Other books in this series and much online documentation focus on applets, graphical interfaces, Web sites, databases, components, and other specific kinds of programming tasks. For other references, see “Further Reading” on page 755.
This fourth edition provides integrated coverage of the Java programming language as provided by the Java 2 Platform Standard Edition 5.0 and specified by the Java Language Specification, Third Edition. It also covers most of the classes in the main packages (java.lang, java.util, java.io) as implemented in the J2SE Development Kit 5.0 (more commonly known as JDK 5.0, or in the older nomenclature JDK 1.5.0).
If you have already read the third edition of this book, you will find some major changes, both in the language and the book, since the 1.3 release that the third edition covered. There are new chapters on generics, enums, and annotationsthe major new language features introduced in the 5.0 releaseand major new sections on assertions and regular expressions. Some existing material has been restructured to accommodate other changes and to improve the general flow of the textsuch as introducing the new boxing and unboxing conversions. But every single chapter has been updated in some way, whether it is a new language feature like variable argument methods; the new enhanced for loop construct; a new class such as Formatter for formatting text output; or changes to classes and methods caused by the addition of generics (such as the collections utilities and the reflection classes)change permeates this entire fourth edition.
The Java programming language shares many features common to most programming languages in use today. The language should look familiar to C and C++ programmers because it was designed with C and C++ constructs where the languages are similar. That said, this book is neither a comparative analysis nor a “bridge” tutorialno knowledge of C or C++ is assumed. C++ programmers, especially, may be as hindered by what they must unlearn as they are helped by their knowledge.
Chapter 1A Quick Tourgives a quick overview of the language. Programmers who are unfamiliar with object-oriented programming notions should read the quick tour, while programmers who are already familiar with object-oriented programming paradigms will find the quick tour a useful introduction to the object-oriented features of the language. The quick tour introduces some of the basic language features on which examples through the rest of the book are built.
Chapters 2 through 6 cover the object-oriented core features of the language, namely, class declarations that define components of a program, and objects manufactured according to class definitions. Chapter 2Classes and Objectsdescribes the basis of the language: classes. Chapter 3Extending Classesdescribes how an existing class can be extended, or subclassed, to create a new class with additional data and behavior. Chapter 4Interfacesdescribes how to declare interface types that are abstract descriptions of behavior that provide maximum flexibility for class designers and implementors. Chapter 5Nested Classes and Interfacesdescribes how classes and interfaces can be declared inside other classes and interfaces, and the benefits that provides. Finally, Chapter 6Enumeration Typescovers the definition and use of type-safe enumeration constants.
Chapters 7 through 10 cover standard constructs common to most languages. Chapter 7Tokens, Values, and Variablesdescribes the tokens of the language from which statements are constructed, the types defined by the language and their allowed values, and the variables that store data in objects, arrays, or locally within methods. Chapter 8Primitives as Typesexplores the relationship between the primitive types and objects of their corresponding wrapper classes, and how boxing and unboxing can transparently convert between them. Chapter 9Operators and Expressionsdescribes the basic operators of the language, how these operators are used to build expressions, and how expressions are evaluated. Chapter 10Control Flowdescribes how control statements direct the order of statement execution.
Chapter 11Generic Typesdescribes generic types: how they are written and used, their power, and their limitations.
Chapter 12Exceptions and Assertionsdescribes the language’s powerful error-handling capabilities, and the use of assertions to validate the expected behavior of code.
Chapter 13Strings and Regular Expressionsdescribes the built-in language and runtime support for String objects, the underlying character set support, and the powerful utilities for regular expression matching.
Chapter 14Threadsexplains the language’s view of multithreading. Many applications, such as graphical interface-based software, must attend to multiple tasks simultaneously. These tasks must cooperate to behave correctly, and threads meet the needs of cooperative multitasking.
Chapter 15Annotationsdescribes the annotation types used to document some of the extra-linguistic properties of classes and method.
Chapter 16Reflectiondescribes the runtime type introspection mechanism and how you can construct and manipulate objects of unknown type dynamically at runtime.
Chapter 17Garbage Collection and Memorytalks about garbage collection, finalization, and lower-strength reference objects.
Chapter 18Packagesdescribes how you can group collections of classes and interfaces into separate packages.
Chapter 19Documentation Commentsshows how to write reference documentation in comments.
Chapters 20 through 24 cover the main packages. Chapter 20The I/O Packagedescribes the input/output system, which is based on streams. Chapter 21Collectionscovers the collection or container classes such as sets and lists. Chapter 22Miscellaneous Utilitiescovers the rest of the utility classes such as bit sets, formatted output, text scanning, and random number generation. Chapter 23System Programmingleads you through the system classes that provide access to features of the underlying platform. Chapter 24Internationalization and Localizationcovers some of the tools used to create programs that can run in many linguistic and cultural environments.
Chapter 25Standard Packagesbriefly explores the packages that are part of the standard platform, giving overviews of those packages not covered in more detail in this book.
Appendix AApplication Evolutionlooks at some of the issues involved in dealing with the evolution of applications and the Java platform, and the impact this has on some of the new language features.
Appendix BUseful Tableshas tables of information that you may find useful for quick reference.
Finally, Further Reading lists works that may be interesting for further reading on complete details, object orientation, programming with threads, software design, and other topics.
Examples and DocumentationAll the code examples in the text have been compiled and run on the latest version of the language available at the time the book was written, which was the JDK 1.5.0_02 product version. Only supported features are covereddeprecated types, methods, and fields are ignored except when unavoidable or when knowledge of the past is necessary to understand the present. We have also covered issues beyond writing programs that simply compile. Part of learning a language is to learn to use it well. For this reason, we have tried to show principles of good programming style and design.
In a few places we refer to online documentation. Development environments provide a way to automatically generate documentation (usually HTML documents) for a compiled class from its documentation comments. This documentation is normally viewed with a Web browser.
Results! Why, man, I have gotten a lot of results. I know several thousand things that won’t work.Thomas Edison