The ability to asynchronously fetch data and load scripts in the browser broadens the capabilities of JavaScript applications. But if you don't understand how the async part works, you'll wind up with unpredictable code that's difficult to maintain. This book is ideal whether you're new to Promises or want to expand your knowledge of this technology.
- Understand how async JavaScript works by delving into callbacks, the event loop, and threading
- Learn how Promises organize callbacks into discrete steps that are easier to read and maintain
- Examine scenarios you'll encounter and techniques you can use when writing real-world applications
- Use features in the Bluebird library and jQuery to work with Promises
- Learn how the Promise API handles asynchronous errors
- Explore ECMAScript 6 language features that simplify Promise-related code
The ability to asynchronously fetch data and load scripts in the browser broadens the capabilities of JavaScript applications. But if you don't understand how the async part works, you'll wind up with unpredictable code that's difficult to maintain. This book is ideal whether you're new to Promises or want to expand your knowledge of this technology.
- Understand how async JavaScript works by delving into callbacks, the event loop, and threading
- Learn how Promises organize callbacks into discrete steps that are easier to read and maintain
- Examine scenarios you'll encounter and techniques you can use when writing real-world applications
- Use features in the Bluebird library and jQuery to work with Promises
- Learn how the Promise API handles asynchronous errors
- Explore ECMAScript 6 language features that simplify Promise-related code
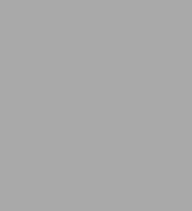
JavaScript with Promises: Managing Asynchronous Code
92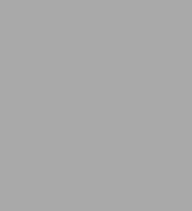
JavaScript with Promises: Managing Asynchronous Code
92Product Details
ISBN-13: | 9781449373214 |
---|---|
Publisher: | O'Reilly Media, Incorporated |
Publication date: | 06/19/2015 |
Pages: | 92 |
Product dimensions: | 6.80(w) x 8.80(h) x 0.30(d) |