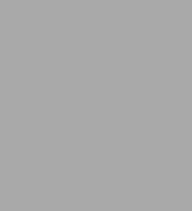
Learning React: Functional Web Development with React and Redux
348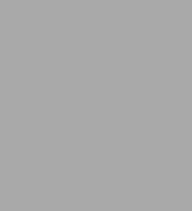
Learning React: Functional Web Development with React and Redux
348Paperback
-
SHIP THIS ITEMTemporarily Out of Stock Online
-
PICK UP IN STORECheck Availability at Nearby Stores
Available within 2 business hours
Related collections and offers
Overview
Developed by Facebook, and used by companies including Netflix, Walmart, and The New York Times for large parts of their web interfaces, React is quickly growing in use. By learning how to build React components with this hands-on guide, you’ll fully understand how useful React can be in your organization.
- Learn key functional programming concepts with JavaScript
- Peek under the hood to understand how React runs in the browser
- Create application presentation layers by mounting and composing React components
- Use component trees to manage data and reduce the time you spend debugging applications
- Explore React’s component lifecycle and use it to load data and improve UI performance
- Use a routing solution for browser history, bookmarks, and other features of single-page applications
- Learn how to structure React applications with servers in mind
Product Details
ISBN-13: | 9781491954621 |
---|---|
Publisher: | O'Reilly Media, Incorporated |
Publication date: | 05/22/2017 |
Pages: | 348 |
Product dimensions: | 7.00(w) x 9.10(h) x 0.90(d) |
About the Author
Eve Porcello is a software architect and cofounder of Moon Highway, a curriculum development company in Northern California. Prior to Moon Highway, Eve worked on software projects for 1-800-Dentist and Microsoft. She is an active corporate trainer, speaker, and author on Lynda.com.
Table of Contents
Preface ix
1 Welcome to React 1
Obstacles and Roadblocks 1
React Is a Library 2
New ECMAScript Syntax 2
Popularity of Functional JavaScript 2
JavaScript Tooling Fatigue 2
Why React Doesn't Have to Be Hard to Learn 3
React's Future 3
Keeping Up with the Changes 4
Working with the Files 4
File Repository 4
React Developer Tools 5
Installing Node.js 6
2 Emerging JavaScript 9
Declaring Variables in ES6 10
Const 10
Let 10
Template Strings 12
Default Parameters 14
Arrow Functions 14
Transpiling ES6 17
ES6 Objects and Arrays 19
Destructuring Assignment 19
Object Literal Enhancement 20
The Spread Operator 22
Promises 24
Classes 25
ES6 Modules 27
CommonJS 28
3 Functional Programming with JavaScript 31
What It Means to Be Functional 32
Imperative Versus Declarative 34
Functional Concepts 36
Immutability 36
Pure Functions 38
Data Transformations 41
Higher-Order Functions 48
Recursion 49
Composition 52
Putting It All Together 54
4 Pure React 59
Page Setup 59
The Virtual DOM 60
React Elements 62
ReactDOM 64
Children 65
Constructing Elements with Data 67
React Components 68
React.createClass 69
React.Component 72
Stateless Functional Components 73
DOM Rendering 74
Factories 77
5 React with JSX 81
React Elements as JSX 81
JSX Tips 82
Babel 84
Recipes as JSX 85
Intro to Webpack 93
Webpack Loaders 94
Recipes App with a Webpack Build 94
6 Props, State, and the Component Tree 109
Property Validation 109
Validating Props with createClass 110
Default Props 114
Custom Property Validation 115
ES6 Classes and Stateless Functional Components 116
Refs 119
Inverse Data Flow 121
Refs in Stateless Functional Components 123
React State Management 123
Introducing Component State 124
Initializing State from Properties 128
State Within the Component Tree 130
Color Organizer App Overview 130
Passing Properties Down the Component Tree 131
Passing Data Back Up the Component Tree 134
7 Enhancing Components 141
Component Lifecycles 141
Mounting Lifecycle 142
Updating Lifecycle 146
React.Children 157
JavaScript Library Integration 158
Making Requests with Fetch 159
Incorporating a D3 Timeline 160
Higher-Order Components 166
Managing State Outside of React 172
Rendering a Clock 173
Flux 174
Views 176
Actions and Action Creators 177
Dispatcher 177
Stores 178
Putting It All Together 179
Flux Implementations 180
8 Redux 183
State 184
Actions 187
Action Payload Data 189
Reducers 190
The Color Reducer 193
The Colors Reducer 195
The Sort Reducer 197
The Store 198
Subscribing to Stores 201
Saving to localStorage 202
Action Creators 203
Middleware 206
Applying Middleware to the Store 207
9 React Redux 211
Explicitly Passing the Store 213
Passing the Store via Context 216
Presentational Versus Container Components 220
The React Redux Provider 223
React Redux connect 224
10 Testing 229
ESLint 229
Testing Redux 233
Test-Driven Development 233
Testing Reducers 234
Testing the Store 242
Testing React Components 245
Setting Up the Jest Environment 245
Enzyme 247
Mocking Components 249
Snapshot Testing 258
Using Code Coverage 262
11 React Router 273
Incorporating the Router 274
Router Properties 277
Nesting Routes 279
Using a Page Template 279
Subsections and Submenus 281
Router Parameters 286
Adding Color Details Page 286
Moving Color Sort State to Router 292
12 React and the Server 297
Isomorphism versus Universalism 297
Server Rendering React 301
Universal Color Organizer 306
Universal Redux 308
Universal Routing 310
Communicating with the Server 318
Completing Actions on the Server 318
Actions with Redux Thunks 321
Index 329