Practices of the Python Pro
Professional developers know the many benefits of writing application code that’s clean, well-organized, and easy to maintain. By learning and following established patterns and best practices, you can take your code and your career to a new level.
With Practices of the Python Pro, you’ll learn to design professional-level, clean, easily maintainable software at scale using the incredibly popular programming language, Python. You’ll find easy-to-grok examples that use pseudocode and Python to introduce software development best practices, along with dozens of instantly useful techniques that will help you code like a pro.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
Professional-quality code does more than just run without bugs. It’s clean, readable, and easy to maintain. To step up from a capable Python coder to a professional developer, you need to learn industry standards for coding style, application design, and development process. That’s where this book is indispensable.
Practices of the Python Pro teaches you to design and write professional-quality software that’s understandable, maintainable, and extensible. Dane Hillard is a Python pro who has helped many dozens of developers make this step, and he knows what it takes. With helpful examples and exercises, he teaches you when, why, and how to modularize your code, how to improve quality by reducing complexity, and much more. Embrace these core principles, and your code will become easier for you and others to read, maintain, and reuse.
For readers familiar with the basics of Python, or another OO language.
Dane Hillard has spent the majority of his development career using Python to build web applications.
Table of Contents:
PART 1 WHY IT ALL MATTERS
1 ¦ The bigger picture
PART 2 FOUNDATIONS OF DESIGN
2 ¦ Separation of concerns
3 ¦ Abstraction and encapsulation
4 ¦ Designing for high performance
5 ¦ Testing your software
PART 3 NAILING DOWN LARGE SYSTEMS
6 ¦ Separation of concerns in practice
7 ¦ Extensibility and flexibility
8 ¦ The rules (and exceptions) of inheritance
9 ¦ Keeping things lightweight
10 ¦ Achieving loose coupling
PART 4 WHAT’S NEXT?
11 ¦ Onward and upward
1132684286
With Practices of the Python Pro, you’ll learn to design professional-level, clean, easily maintainable software at scale using the incredibly popular programming language, Python. You’ll find easy-to-grok examples that use pseudocode and Python to introduce software development best practices, along with dozens of instantly useful techniques that will help you code like a pro.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
Professional-quality code does more than just run without bugs. It’s clean, readable, and easy to maintain. To step up from a capable Python coder to a professional developer, you need to learn industry standards for coding style, application design, and development process. That’s where this book is indispensable.
Practices of the Python Pro teaches you to design and write professional-quality software that’s understandable, maintainable, and extensible. Dane Hillard is a Python pro who has helped many dozens of developers make this step, and he knows what it takes. With helpful examples and exercises, he teaches you when, why, and how to modularize your code, how to improve quality by reducing complexity, and much more. Embrace these core principles, and your code will become easier for you and others to read, maintain, and reuse.
- Organizing large Python projects
- Achieving the right levels of abstraction
- Writing clean, reusable code Inheritance and composition
- Considerations for testing and performance
For readers familiar with the basics of Python, or another OO language.
Dane Hillard has spent the majority of his development career using Python to build web applications.
Table of Contents:
PART 1 WHY IT ALL MATTERS
1 ¦ The bigger picture
PART 2 FOUNDATIONS OF DESIGN
2 ¦ Separation of concerns
3 ¦ Abstraction and encapsulation
4 ¦ Designing for high performance
5 ¦ Testing your software
PART 3 NAILING DOWN LARGE SYSTEMS
6 ¦ Separation of concerns in practice
7 ¦ Extensibility and flexibility
8 ¦ The rules (and exceptions) of inheritance
9 ¦ Keeping things lightweight
10 ¦ Achieving loose coupling
PART 4 WHAT’S NEXT?
11 ¦ Onward and upward
Practices of the Python Pro
Professional developers know the many benefits of writing application code that’s clean, well-organized, and easy to maintain. By learning and following established patterns and best practices, you can take your code and your career to a new level.
With Practices of the Python Pro, you’ll learn to design professional-level, clean, easily maintainable software at scale using the incredibly popular programming language, Python. You’ll find easy-to-grok examples that use pseudocode and Python to introduce software development best practices, along with dozens of instantly useful techniques that will help you code like a pro.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
Professional-quality code does more than just run without bugs. It’s clean, readable, and easy to maintain. To step up from a capable Python coder to a professional developer, you need to learn industry standards for coding style, application design, and development process. That’s where this book is indispensable.
Practices of the Python Pro teaches you to design and write professional-quality software that’s understandable, maintainable, and extensible. Dane Hillard is a Python pro who has helped many dozens of developers make this step, and he knows what it takes. With helpful examples and exercises, he teaches you when, why, and how to modularize your code, how to improve quality by reducing complexity, and much more. Embrace these core principles, and your code will become easier for you and others to read, maintain, and reuse.
For readers familiar with the basics of Python, or another OO language.
Dane Hillard has spent the majority of his development career using Python to build web applications.
Table of Contents:
PART 1 WHY IT ALL MATTERS
1 ¦ The bigger picture
PART 2 FOUNDATIONS OF DESIGN
2 ¦ Separation of concerns
3 ¦ Abstraction and encapsulation
4 ¦ Designing for high performance
5 ¦ Testing your software
PART 3 NAILING DOWN LARGE SYSTEMS
6 ¦ Separation of concerns in practice
7 ¦ Extensibility and flexibility
8 ¦ The rules (and exceptions) of inheritance
9 ¦ Keeping things lightweight
10 ¦ Achieving loose coupling
PART 4 WHAT’S NEXT?
11 ¦ Onward and upward
With Practices of the Python Pro, you’ll learn to design professional-level, clean, easily maintainable software at scale using the incredibly popular programming language, Python. You’ll find easy-to-grok examples that use pseudocode and Python to introduce software development best practices, along with dozens of instantly useful techniques that will help you code like a pro.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
Professional-quality code does more than just run without bugs. It’s clean, readable, and easy to maintain. To step up from a capable Python coder to a professional developer, you need to learn industry standards for coding style, application design, and development process. That’s where this book is indispensable.
Practices of the Python Pro teaches you to design and write professional-quality software that’s understandable, maintainable, and extensible. Dane Hillard is a Python pro who has helped many dozens of developers make this step, and he knows what it takes. With helpful examples and exercises, he teaches you when, why, and how to modularize your code, how to improve quality by reducing complexity, and much more. Embrace these core principles, and your code will become easier for you and others to read, maintain, and reuse.
- Organizing large Python projects
- Achieving the right levels of abstraction
- Writing clean, reusable code Inheritance and composition
- Considerations for testing and performance
For readers familiar with the basics of Python, or another OO language.
Dane Hillard has spent the majority of his development career using Python to build web applications.
Table of Contents:
PART 1 WHY IT ALL MATTERS
1 ¦ The bigger picture
PART 2 FOUNDATIONS OF DESIGN
2 ¦ Separation of concerns
3 ¦ Abstraction and encapsulation
4 ¦ Designing for high performance
5 ¦ Testing your software
PART 3 NAILING DOWN LARGE SYSTEMS
6 ¦ Separation of concerns in practice
7 ¦ Extensibility and flexibility
8 ¦ The rules (and exceptions) of inheritance
9 ¦ Keeping things lightweight
10 ¦ Achieving loose coupling
PART 4 WHAT’S NEXT?
11 ¦ Onward and upward
39.99
In Stock
5
1
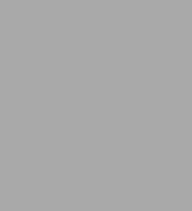
Practices of the Python Pro
248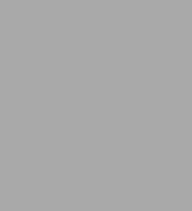
Practices of the Python Pro
248Paperback(1st Edition)
$39.99
39.99
In Stock
From the B&N Reads Blog