Programmer's Guide to Apache Thrift
Summary
Programmer's Guide to Apache Thrift provides comprehensive coverage of the Apache Thrift framework along with a developer's-eye view of modern distributed application architecture.
Foreword by Jens Geyer.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the Technology
Thrift-based distributed software systems are built out of communicating components that use different languages, protocols, and message types. Sitting between them is Thrift, which handles data serialization, transport, and service implementation. Thrift supports many client and server environments and a host of languages ranging from PHP to JavaScript, and from C++ to Go.
About the Book
Programmer's Guide to Apache Thrift provides comprehensive coverage of distributed application communication using the Thrift framework. Packed with code examples and useful insight, this book presents best practices for multi-language distributed development. You'll take a guided tour through transports, protocols, IDL, and servers as you explore programs in C++, Java, and Python. You'll also learn how to work with platforms ranging from browser-based clients to enterprise servers.
What's inside
About the Reader
Readers should be comfortable with a language like Python, Java, or C++ and the basics of service-oriented or microservice architectures.
About the Author
Randy Abernethy is an Apache Thrift Project Management Committee member and a partner at RX-M.
Table of Contents
1120132574
Programmer's Guide to Apache Thrift provides comprehensive coverage of the Apache Thrift framework along with a developer's-eye view of modern distributed application architecture.
Foreword by Jens Geyer.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the Technology
Thrift-based distributed software systems are built out of communicating components that use different languages, protocols, and message types. Sitting between them is Thrift, which handles data serialization, transport, and service implementation. Thrift supports many client and server environments and a host of languages ranging from PHP to JavaScript, and from C++ to Go.
About the Book
Programmer's Guide to Apache Thrift provides comprehensive coverage of distributed application communication using the Thrift framework. Packed with code examples and useful insight, this book presents best practices for multi-language distributed development. You'll take a guided tour through transports, protocols, IDL, and servers as you explore programs in C++, Java, and Python. You'll also learn how to work with platforms ranging from browser-based clients to enterprise servers.
What's inside
- Complete coverage of Thrift's IDL
- Building and serializing complex user-defined types
- Plug-in protocols, transports, and data compression
- Creating cross-language services with RPC and messaging systems
About the Reader
Readers should be comfortable with a language like Python, Java, or C++ and the basics of service-oriented or microservice architectures.
About the Author
Randy Abernethy is an Apache Thrift Project Management Committee member and a partner at RX-M.
Table of Contents
- PART 1 - APACHE THRIFT OVERVIEW
- Introduction to Apache Thrift
- Apache Thrift architecture
- Building, testing, and debugging PART 2 - PROGRAMMING APACHE THRIFT
- Moving bytes with transports
- Serializing data with protocols
- Apache Thrift IDL
- User-defined types
- Implementing services
- Handling exceptions
- Servers PART 3 - APACHE THRIFT LANGUAGES
- Building clients and servers with C++
- Building clients and servers with Java
- Building C# clients and servers with .NET Core and Windows
- Building Node.js clients and servers
- Apache Thrift and JavaScript
- Scripting Apache Thrift
- Thrift in the enterprise
Programmer's Guide to Apache Thrift
Summary
Programmer's Guide to Apache Thrift provides comprehensive coverage of the Apache Thrift framework along with a developer's-eye view of modern distributed application architecture.
Foreword by Jens Geyer.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the Technology
Thrift-based distributed software systems are built out of communicating components that use different languages, protocols, and message types. Sitting between them is Thrift, which handles data serialization, transport, and service implementation. Thrift supports many client and server environments and a host of languages ranging from PHP to JavaScript, and from C++ to Go.
About the Book
Programmer's Guide to Apache Thrift provides comprehensive coverage of distributed application communication using the Thrift framework. Packed with code examples and useful insight, this book presents best practices for multi-language distributed development. You'll take a guided tour through transports, protocols, IDL, and servers as you explore programs in C++, Java, and Python. You'll also learn how to work with platforms ranging from browser-based clients to enterprise servers.
What's inside
About the Reader
Readers should be comfortable with a language like Python, Java, or C++ and the basics of service-oriented or microservice architectures.
About the Author
Randy Abernethy is an Apache Thrift Project Management Committee member and a partner at RX-M.
Table of Contents
Programmer's Guide to Apache Thrift provides comprehensive coverage of the Apache Thrift framework along with a developer's-eye view of modern distributed application architecture.
Foreword by Jens Geyer.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the Technology
Thrift-based distributed software systems are built out of communicating components that use different languages, protocols, and message types. Sitting between them is Thrift, which handles data serialization, transport, and service implementation. Thrift supports many client and server environments and a host of languages ranging from PHP to JavaScript, and from C++ to Go.
About the Book
Programmer's Guide to Apache Thrift provides comprehensive coverage of distributed application communication using the Thrift framework. Packed with code examples and useful insight, this book presents best practices for multi-language distributed development. You'll take a guided tour through transports, protocols, IDL, and servers as you explore programs in C++, Java, and Python. You'll also learn how to work with platforms ranging from browser-based clients to enterprise servers.
What's inside
- Complete coverage of Thrift's IDL
- Building and serializing complex user-defined types
- Plug-in protocols, transports, and data compression
- Creating cross-language services with RPC and messaging systems
About the Reader
Readers should be comfortable with a language like Python, Java, or C++ and the basics of service-oriented or microservice architectures.
About the Author
Randy Abernethy is an Apache Thrift Project Management Committee member and a partner at RX-M.
Table of Contents
- PART 1 - APACHE THRIFT OVERVIEW
- Introduction to Apache Thrift
- Apache Thrift architecture
- Building, testing, and debugging PART 2 - PROGRAMMING APACHE THRIFT
- Moving bytes with transports
- Serializing data with protocols
- Apache Thrift IDL
- User-defined types
- Implementing services
- Handling exceptions
- Servers PART 3 - APACHE THRIFT LANGUAGES
- Building clients and servers with C++
- Building clients and servers with Java
- Building C# clients and servers with .NET Core and Windows
- Building Node.js clients and servers
- Apache Thrift and JavaScript
- Scripting Apache Thrift
- Thrift in the enterprise
59.99
In Stock
5
1
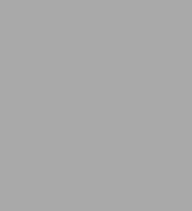
Programmer's Guide to Apache Thrift
592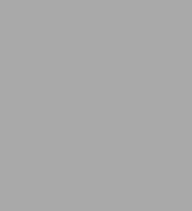
Programmer's Guide to Apache Thrift
592Paperback(1st Edition)
$59.99
59.99
In Stock
From the B&N Reads Blog