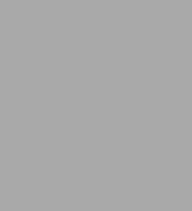
Python One-Liners: Write Concise, Eloquent Python Like a Professional
216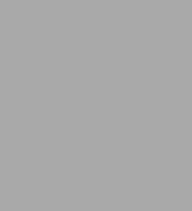
Python One-Liners: Write Concise, Eloquent Python Like a Professional
216eBook
Available on Compatible NOOK devices, the free NOOK App and in My Digital Library.
Related collections and offers
Overview
Python One-Liners will teach you how to read and write "one-liners": concise statements of useful functionality packed into a single line of code. You'll learn how to systematically unpack and understand any line of Python code, and write eloquent, powerfully compressed Python like an expert.
The book's five chapters cover tips and tricks, regular expressions, machine learning, core data science topics, and useful algorithms. Detailed explanations of one-liners introduce key computer science concepts and boost your coding and analytical skills. You'll learn about advanced Python features such as list comprehension, slicing, lambda functions, regular expressions, map and reduce functions, and slice assignments. You'll also learn how to:
• Leverage data structures to solve real-world problems, like using Boolean indexing to find cities with above-average pollution
• Use NumPy basics such as array, shape, axis, type, broadcasting, advanced indexing, slicing, sorting, searching, aggregating, and statistics
• Calculate basic statistics of multidimensional data arrays and the K-Means algorithms for unsupervised learning
• Create more advanced regular expressions using grouping and named groups, negative lookaheads, escaped characters, whitespaces, character sets (and negative characters sets), and greedy/nongreedy operators
• Understand a wide range of computer science topics, including anagrams, palindromes, supersets, permutations, factorials, prime numbers, Fibonacci numbers, obfuscation, searching, and algorithmic sorting
By the end of the book, you'll know how to write Python at its most refined, and create concise, beautiful pieces of "Python art" in merely a single line.
Product Details
ISBN-13: | 9781718500518 |
---|---|
Publisher: | No Starch Press |
Publication date: | 05/12/2020 |
Sold by: | Penguin Random House Publisher Services |
Format: | eBook |
Pages: | 216 |
File size: | 19 MB |
Note: | This product may take a few minutes to download. |
About the Author
Table of Contents
Acknowledgments xvii
Introduction xix
Python One-Liner Example xx
A Note on Readability xxi
Who Is This Book For? xxii
What Will You Learn? xxii
Online Resources xxiii
1 Python Refresher 1
Basic Data Structures 2
Numerical Data Types and Structures 2
Booleans 2
Strings 4
The Keyword None 5
Container Data Structures 6
Lists 6
Stacks 8
Sets 9
Dictionaries 10
Membership 11
List and Set Comprehension 12
Control Flow 12
If, else, and elif 13
Loops 13
Functions 14
Lambdas 15
Summary 16
2 Python Tricks 17
Using List Comprehension to Find Top Earners 18
The Basics 18
The Code 20
How It Works 20
Using List Comprehension to Find Words with High Information Value 21
The Basics 21
The Code 21
How It Works 22
Reading a File 22
The Basics 22
The Code 23
How It Works 23
Using Lambda and Map Functions 24
The Basics 24
The Code 25
How It Works 26
Using Slicing to Extract Matching Substring Environments 26
The Basics 26
The Code 28
How It Works 29
Combining List Comprehension and Slicing 29
The Basics 29
The Code 30
How It Works 30
Using Slice Assignment to Correct Corrupted Lists 31
The Basics 31
The Code 32
How It Works 32
Analyzing Cardiac Health Data with List Concatenation 33
The Basics 33
The Code 35
How It Works 35
Using Generator Expressions to Find Companies That Pay Below Minimum Wage 35
The Basics 35
The Code 36
How It Works 36
Formatting Databases with the zip() Function 37
The Basics 37
The Code 38
How It Works 39
Summary 39
3 Data Science 41
Basic Two-Dimensional Array Arithmetic 42
The Basics 42
The Code 45
How It Works 45
Working with NumPy Arrays: Slicing, Broadcasting, and Array Types 46
The Basics 46
The Code 51
How It Works 52
Conditional Array Search, Filtering, and Broadcasting to Detect Outliers 53
The Basics 53
The Code 54
How It Works 55
Boolean Indexing to Filter Two-Dimensional Arrays 57
The Basics 57
The Code 58
How It Works 58
Broadcasting, Slice Assignment, and Reshaping to Clean Every i-th Array Element 60
The Basics 60
The Code 62
How It Works 63
When to Use the sort() Function and When to Use the argsort() Function in NumPy 64
The Basics 64
The Code 66
How It Works 66
How to Use Lambda Functions and Boolean Indexing to Filter Arrays 68
The Basics 68
The Code 68
How It Works 69
How to Create Advanced Array Filters with Statistics, Math, and Logic 70
The Basics 70
The Code 73
How It Works 74
Simple Association Analysis: People Who Bought X Also Bought Y 74
The Basics 74
The Code 75
How It Works 76
Intermediate Association Analysis to Find Bestseller Bundles 77
The Basics 77
The Code 77
How It Works 78
Summary 79
4 Machine Learning 81
The Basics of Supervised Machine Learning 82
Training Phase 82
Inference Phase 83
Linear Regression 83
The Basics 83
The Code 86
How It Works 87
Logistic Regression in One Line 89
The Basics 89
The Code 92
How It Works 93
K-Means Clustering in One Line 94
The Basics 94
The Code 97
How It Works 97
K-Nearest Neighbors in One Line 100
The Basics 100
The Code 101
How It Works 102
Neural Network Analysis in One Line 104
The Basics 104
The Code 108
How It Works 109
Decision-Tree Learning in One Line 111
The Basics 111
The Code 112
How It Works 113
Get Row with Minimal Variance in One Line 113
The Basics 113
The Code 114
How It Works 115
Basic Statistics in One Line 116
The Basics 116
The Code 118
How It Works 118
Classification with Support-Vector Machines in One Line 119
The Basics 120
The Code 121
How It Works 122
Classification with Random Forests in One Line 123
The Basics 123
The Code 124
How It Works 125
Summary 126
5 Regular Expressions 127
Finding Basic Textual Patterns in Strings 128
The Basics 128
The Code 130
How It Works 131
Writing Your First Web Scraper with Regular Expressions 132
The Basics 132
The Code 133
How It Works 133
Analyzing Hyperlinks of HTML Documents 134
The Basics 134
The Code 136
How It Works 137
Extracting Dollars from a String 137
The Basics 138
The Code 138
How It Works 139
Finding Nonsecure HTTP URLs 140
The Basics 140
The Code 140
How It Works 141
Validating the Time Format of User Input, Part 1 141
The Basics 142
The Code 142
How It Works 143
Validating Time Format of User input, Part 2 143
The Basics 143
The Code 144
How It Works 144
Duplicate Detection in Strings 145
The Basics 145
The Code 146
How It Works 146
Detecting Word Repetitions 147
The Basics 147
The Code 147
How It Works 148
Modifying Regex Patterns in a Multiline String 148
The Basics 149
The Code 149
How It Works 149
Summary 150
6 Algorithms 151
Finding Anagrams with Lambda Functions and Sorting 152
The Basics 152
The Code 153
How It Works 153
Finding Palindromes with Lambda Functions and Negative Slicing 154
The Basics 154
The Code 155
How It Works 155
Counting Permutations with Recursive Factorial Functions 156
The Basics 156
The Code 158
How It Works 158
Finding the Levenshtein Distance 159
The Basics 159
The Code 160
How It Works 160
Calculating the Powerset by Using Functional Programming 162
The Basics 162
The Code 164
How It Works 165
Caesar's Cipher Encryption Using Advanced Indexing and List Comprehension 165
The Basics 165
The Code 166
How It Works 167
Finding Prime Numbers with the Sieve of Eratosthenes 168
The Basics 168
The Code 169
How It Works 170
Calculating the Fibonacci Series with the reduce() Function 174
The Basics 174
The Code 175
How It Works 175
A Recursive Binary Search Algorithm 176
The Basics 177
The Code 178
How It Works 179
A Recursive Quicksort Algorithm 180
The Basics 180
The Code 181
How It Works 181
Summary 182
Afterword 183
Index 185