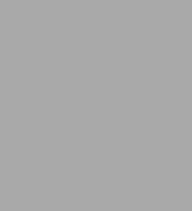
RxJS in Action / Edition 1
- ISBN-10:
- 1617293415
- ISBN-13:
- 9781617293412
- Pub. Date:
- 08/04/2017
- Publisher:
- Manning
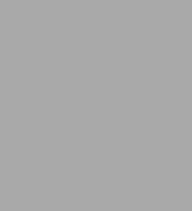
RxJS in Action / Edition 1
Buy New
$49.99-
PICK UP IN STORE
Your local store may have stock of this item.
Available within 2 business hours
Overview
RxJS in Action gives you the development skills you need to create reactive applications with RxJS. This book is full of theory and practical examples that build on each other and help you begin thinking in a reactive manner. Foreword by Ben Lesh, Project lead, RxJS 5.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the Technology
On the web, events and messages flow constantly between UI and server components. With RxJS, you can filter, merge, and transform these streams directly, opening the world of data flow programming to browser-based apps. This JavaScript implementation of the ReactiveX spec is perfect for on-the-fly tasks like autocomplete. Its asynchronous communication model makes concurrency much, much easier.
About the Book
RxJS in Action is your guide to building a reactive web UI using RxJS. You'll begin with an intro to stream-based programming as you explore the power of RxJS through practical examples. With the core concepts in hand, you'll tackle production techniques like error handling, unit testing, and interacting with frameworks like React and Redux. And because RxJS builds on ideas from the world of functional programming, you'll even pick up some key FP concepts along the way.
What's Inside
- Building clean, declarative, fault-tolerant applications
- Transforming and composing streams
- Taming asynchronous processes
- Integrating streams with third-party libraries
- Covers RxJS 5
About the Reader
This book is suitable for readers comfortable with JavaScript and standard web application architectures.
About the Author
Paul P. Daniels is a professional software engineer with experience in .NET, Java, and JavaScript. Luis Atencio is a software engineer working daily with Java, PHP, and JavaScript platforms, and author of Manning's Functional Programming in JavaScript.
Table of Contents
- PART 1 - UNDERSTANDING STREAMS
- Thinking reactively
- Reacting with RxJS
- Core operators
- It's about time you used RxJS PART 2 - OBSERVABLES IN PRACTICE
- Applied reactive streams
- Coordinating business processes
- Error handling with RxJS PART 3 MASTERING RXJS
- Heating up observables
- Toward testable, reactive programs
- RxJS in the wild
Product Details
ISBN-13: | 9781617293412 |
---|---|
Publisher: | Manning |
Publication date: | 08/04/2017 |
Edition description: | 1st Edition |
Pages: | 352 |
Product dimensions: | 7.30(w) x 9.10(h) x 0.80(d) |
About the Author
is a professional Software Engineer with a B.S.E in Computer Science and over 5 years of experience in software engineering, developing applications in .Net, Java, and JavaScript. A regular user of RxJS, he is active as a member and a contributor in the RxJS community.
Luis Atencio is a Staff Software Engineer for Citrix Systems. He has a B.S. and an M.S. in Computer Science and works full-time developing and architecting web applications using Java, PHP, and JavaScript platforms. Luis is also the author of Functional Programming in JavaScript.
Table of Contents
Foreword xiii
Preface xv
Acknowledgments xvii
About this book xix
About the authors xxiv
About the cover xxv
Part 1 Understanding Streams 1
1 Thinking reactively 3
1.1 Synchronous vs. asynchronous computing 5
Issues with blocking code 5
Non-blocking code with callback functions 6
Understanding time and space 7
Are callbacks out of the picture? 9
Event emitters 11
1.2 Better callbacks with Promises 12
1.3 The need for a different paradigm 14
1.4 The Reactive Extensions for JavaScript 17
Thinking in streams: data flows and propagation 17
Introducing the RxJS project 18
Everything is a stream 19
Abstracting the notion of time from your programs 21
Components of an Rx stream 23
1.5 Reactive and other programming paradigms 26
1.6 Summary 27
2 Reacting with RxJS 28
2.1 Functional programming as the pillar of reactive programming 29
Functional programming 30
The iterator pattern 38
2.2 Stream's data-driven approach 41
2.3 Wrapping data sources with Rx.Observable 43
Identifying different sources of data 43
Creating RxJS observables 44
When and where to use RxJS 46
To push or not to push 49
2.4 Consuming data with observers 53
The Observer API 53
Creating bare observables 55
Observable modules 57
2.5 Summary 60
3 Core operators 61
3.1 Evaluating and cancelling streams 62
Downside of eager allocation 62
Lazy allocation and subscribing to observables 64
Disposing of subscriptions: explicit cancellation 65
Cancellation mismatch between RxJS and other APIs 67
3.2 Popular RxJS observable operators 69
Introducing the core operators 70
3.3 Sequencing operator pipelines with aggregates 77
Self-contained pipelines and referential transparency 77
Performance advantages of sequencing with RxJS 80
3.4 Summary 83
4 It's about time you used RxJS 85
4.1 Why worry about time? 87
4.2 Understanding asynchronous timing with JavaScript 88
Implicit timing 88
Explicit timing 88
The JavaScript timing interfaces 90
4.3 Back to the future with RxJS 94
Propagation 98
Sequential time 99
4.4 Handling user input 101
Debouncing 101
Throttling 108
4.5 Buffering in RxJS 111
4.6 Summary 116
Part 2 Observables in Practice 119
5 Applied reactive streams 121
5.1 One for all, and all for one! 122
Interleave events by merging streams 124
Preserve order of events by concatenating streams 130
Switch to the latest observable data 133
5.2 Unwinding nested observables: the case of mergeMap 135
5.3 Mastering asynchronous streams 141
5.4 Drag and drop with concatMap 146
5.5 Summary 150
6 Coordinating business processes 151
6.1 Hooking into the observable lifecycle 152
Web hooks and the observer pattern 153
Hooked on observables 154
6.2 Joining parallel streams with combineLatest and forkJoin 159
Limitations of using Promises 162
Combining parallel streams 163
More coordination with forkJoin 168
6.3 Building a reactive database 170
Populating a database reactively 172
Writing bulk data 175
Joining related database operations 177
Reactive databases 180
6.4 Summary 181
7 Error handling with RxJS 182
7.1 Common error-handling techniques 183
Error handling with try/catch 183
Delegating errors to callbacks 184
Errors and Promises 186
7.2 Incompatibilities between imperative error-handling techniques and functional and reactive code bases 188
7.3 Understanding the functional error-handling approach 189
7.4 The RxJS way of dealing with failure 193
Errors propagated downstream to observers 193
Catching and reacting to errors 195
Retrying failed streams for a fixed number of times 197
Reacting to failed retries 199
7.5 Summary 208
Part 3 Mastering RxJS 209
8 Heating up observables 211
8.1 Introducing hot and cold observables 212
Cold observables 212
Hot observables 215
8.2 A new type of data source: WebSockets 217
A brief look at WebSocket 218
A simple WebSocket server in Node.js 219
WebSocket client 220
8.3 The impact of side effects on a resubscribe or a replay 221
Replay vs. resubscribe 222
Replaying the logic of a stream 222
Resubscribing to a stream 224
8.4 Changing the temperature of an observable 226
Producers as thermometers 227
Making a hot observable cold 228
Making a cold observable hot 230
Creating hot-by-operator streams 232
8.5 Connecting one observable to many observers 237
Publish 237
Publish with replay 240
Publish last 242
8.6 Summary 243
9 Toward testable, reactive programs 245
9.1 Testing is inherently built into functional programs 246
9.2 Testing asynchronous code and promises 250
Testing AJAX requests 250
Working with Promises 253
9.3 Testing reactive streams 255
9.4 Making streams testable 258
9.5 Scheduling values in RxJS 260
9.6 Augmenting virtual reality 263
Playing with marbles 264
Fake it 'til you make it 266
Refactoring your search stream for testability 267
9.7 Summary 270
10 RxJS in the wild 271
10.1 Building a basic banking application 273
10.2 Introduction to React and Redux 274
Rendering UI components with React 274
Stale management with Redux 284
10.3 Redux-ing application state 286
Actions and reducers 286
Redux store 287
10.4 Building a hot RxJS and Redux store adapter 290
10.5 Asynchronous middleware with RxJS Subject 291
RxJS subjects 294
Building epic, reactive middleware 296
10.6 Bringing it all home 302
10.7 Parting words 304
10.8 Summary 304
Appendix A Installation of libraries used in this book 305
Appendix B Choosing an operator 310
Index 315