Spring Microservices in Action
Summary
Spring Microservices in Action teaches you how to build microservice-based applications using Java and the Spring platform.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the technology
Microservices break up your code into small, distributed, and independent services that require careful forethought and design. Fortunately, Spring Boot and Spring Cloud simplify your microservice applications, just as the Spring Framework simplifies enterprise Java development. Spring Boot removes the boilerplate code involved with writing a REST-based service. Spring Cloud provides a suite of tools for the discovery, routing, and deployment of microservices to the enterprise and the cloud.
About the Book
Spring Microservices in Action teaches you how to build microservice-based applications using Java and the Spring platform. You'll learn to do microservice design as you build and deploy your first Spring Cloud application. Throughout the book, carefully selected real-life examples expose microservice-based patterns for configuring, routing, scaling, and deploying your services. You'll see how Spring's intuitive tooling can help augment and refactor existing applications with micro services.
What's Inside
About the Reader
This book is written for developers with Java and Spring experience.
About the Author
John Carnell is a senior cloud engineer with twenty years of experience in Java.
Table of contents
1133085029
Spring Microservices in Action teaches you how to build microservice-based applications using Java and the Spring platform.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the technology
Microservices break up your code into small, distributed, and independent services that require careful forethought and design. Fortunately, Spring Boot and Spring Cloud simplify your microservice applications, just as the Spring Framework simplifies enterprise Java development. Spring Boot removes the boilerplate code involved with writing a REST-based service. Spring Cloud provides a suite of tools for the discovery, routing, and deployment of microservices to the enterprise and the cloud.
About the Book
Spring Microservices in Action teaches you how to build microservice-based applications using Java and the Spring platform. You'll learn to do microservice design as you build and deploy your first Spring Cloud application. Throughout the book, carefully selected real-life examples expose microservice-based patterns for configuring, routing, scaling, and deploying your services. You'll see how Spring's intuitive tooling can help augment and refactor existing applications with micro services.
What's Inside
- Core microservice design principles
- Managing configuration with Spring Cloud Config
- Client-side resiliency with Spring, Hystrix, and Ribbon
- Intelligent routing using Netflix Zuul
- Deploying Spring Cloud applications
About the Reader
This book is written for developers with Java and Spring experience.
About the Author
John Carnell is a senior cloud engineer with twenty years of experience in Java.
Table of contents
- Welcome to the cloud, Spring
- Building microservices with Spring Boot
- Controlling your configuration with Spring Cloud configuration server
- On service discovery
- When bad things happen: client resiliency patterns with Spring Cloud and Netflix Hystrix
- Service routing with Spring Cloud and Zuul
- Securing your microservices
- Event-driven architecture with Spring Cloud Stream
- Distributed tracing with Spring Cloud Sleuth and Zipkin
- Deploying your microservices
Spring Microservices in Action
Summary
Spring Microservices in Action teaches you how to build microservice-based applications using Java and the Spring platform.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the technology
Microservices break up your code into small, distributed, and independent services that require careful forethought and design. Fortunately, Spring Boot and Spring Cloud simplify your microservice applications, just as the Spring Framework simplifies enterprise Java development. Spring Boot removes the boilerplate code involved with writing a REST-based service. Spring Cloud provides a suite of tools for the discovery, routing, and deployment of microservices to the enterprise and the cloud.
About the Book
Spring Microservices in Action teaches you how to build microservice-based applications using Java and the Spring platform. You'll learn to do microservice design as you build and deploy your first Spring Cloud application. Throughout the book, carefully selected real-life examples expose microservice-based patterns for configuring, routing, scaling, and deploying your services. You'll see how Spring's intuitive tooling can help augment and refactor existing applications with micro services.
What's Inside
About the Reader
This book is written for developers with Java and Spring experience.
About the Author
John Carnell is a senior cloud engineer with twenty years of experience in Java.
Table of contents
Spring Microservices in Action teaches you how to build microservice-based applications using Java and the Spring platform.
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the technology
Microservices break up your code into small, distributed, and independent services that require careful forethought and design. Fortunately, Spring Boot and Spring Cloud simplify your microservice applications, just as the Spring Framework simplifies enterprise Java development. Spring Boot removes the boilerplate code involved with writing a REST-based service. Spring Cloud provides a suite of tools for the discovery, routing, and deployment of microservices to the enterprise and the cloud.
About the Book
Spring Microservices in Action teaches you how to build microservice-based applications using Java and the Spring platform. You'll learn to do microservice design as you build and deploy your first Spring Cloud application. Throughout the book, carefully selected real-life examples expose microservice-based patterns for configuring, routing, scaling, and deploying your services. You'll see how Spring's intuitive tooling can help augment and refactor existing applications with micro services.
What's Inside
- Core microservice design principles
- Managing configuration with Spring Cloud Config
- Client-side resiliency with Spring, Hystrix, and Ribbon
- Intelligent routing using Netflix Zuul
- Deploying Spring Cloud applications
About the Reader
This book is written for developers with Java and Spring experience.
About the Author
John Carnell is a senior cloud engineer with twenty years of experience in Java.
Table of contents
- Welcome to the cloud, Spring
- Building microservices with Spring Boot
- Controlling your configuration with Spring Cloud configuration server
- On service discovery
- When bad things happen: client resiliency patterns with Spring Cloud and Netflix Hystrix
- Service routing with Spring Cloud and Zuul
- Securing your microservices
- Event-driven architecture with Spring Cloud Stream
- Distributed tracing with Spring Cloud Sleuth and Zipkin
- Deploying your microservices
49.99
In Stock
5
1
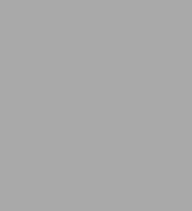
Spring Microservices in Action
384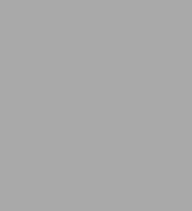
Spring Microservices in Action
384Paperback(1st Edition)
$49.99
49.99
In Stock
From the B&N Reads Blog