Subfiles in Free-Format RPG: Rules, Examples, Techniques, and Other Cool Stuff / Edition 2 available in Paperback
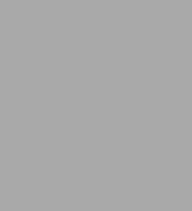
Subfiles in Free-Format RPG: Rules, Examples, Techniques, and Other Cool Stuff / Edition 2
- ISBN-10:
- 1583470948
- ISBN-13:
- 9781583470947
- Pub. Date:
- 08/09/2011
- Publisher:
- MC Press, LLC
- ISBN-10:
- 1583470948
- ISBN-13:
- 9781583470947
- Pub. Date:
- 08/09/2011
- Publisher:
- MC Press, LLC
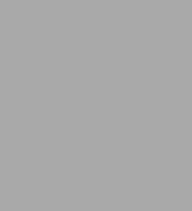
Subfiles in Free-Format RPG: Rules, Examples, Techniques, and Other Cool Stuff / Edition 2
Paperback
Buy New
$79.95Buy Used
-
-
SHIP THIS ITEM
Temporarily Out of Stock Online
Please check back later for updated availability.
-
Overview
Product Details
ISBN-13: | 9781583470947 |
---|---|
Publisher: | MC Press, LLC |
Publication date: | 08/09/2011 |
Edition description: | Second Edition, Second edition |
Pages: | 240 |
Product dimensions: | 6.90(w) x 8.90(h) x 0.60(d) |
About the Author
Kevin Vandever is director of systems development at Behr Process Corporation. He has been involved with the IBM mid-market platforms, including the IBM System i and all of its predecessors, for many years. A frequent and popular speaker at user groups and conferences, he is also a regular contributor to industry magazines. He lives in Huntington Beach, California.
Read an Excerpt
Subfiles in Free-Format RPG
Rules, Examples, Techniques, and Other Cool Stuff
By Kevin Vandever
MC Press
Copyright © 2011 Kevin VandeverAll rights reserved.
ISBN: 978-1-58347-094-7
CHAPTER 1
The Specifics on Subfiles
According to IBM, a subfile is a group of records that have the same record format and are read from and written to a display station in one operation. As this definition suggests, a subfile is not a file; rather, it is a temporary place to store data of the same format to be used by a display file program.
Why Use Subfiles?
Figure 1.1 shows the typical use of a subfile. As you can see, subfiles are useful when you want to list multiple, like records on a display. A major benefit of subfiles is that you can define them so the number of displayed records exceeds the number of lines available on the screen, allowing the user to scroll, or page, through the data. This is usually the reason you would decide to use subfiles in your display program. Because of their ease of use, however, I deploy subfiles even when I think I will never display more than one screen, because they are easy to change if the data someday requires more than one screen.
In addition to simply displaying multiple lines of data, you can use subfiles and their multiple-line capabilities to add, change, and delete records very effectively. You can also use subfiles in a non-display manner, to create self-extending data structures and arrays in your RPG program. They work like multiple-occurrence data structures and arrays, except that instead of having to hard-code a number of elements large enough to hold the maximum number of entries, you can use subfiles. You start with a small number of entries and allow the number to expand dynamically, as your data expands.
Why Should You Care?
Haven't programmers been able to display data to a screen for years, without subfiles? Yes, but subfiles allow you to display lists of like data that can extend beyond one screen. They make it easier for you to create display applications, which, in turn, makes you more productive. Subfile programs are easy to write and maintain because much of the work is done for you in the Data Definition Specifications (DDS). Most of the time, you can change the characteristics of a subfile program without having to modify the RPG code driving it.
If that isn't enough, keep in mind that the ability to code subfiles is often the measure of an RPG programmer's worth. As an added benefit, when you're talking with a group of programmers at a users' group or technical conference, you can hold your head high, knowing that you're right up there with the rest of the subfile- savvy programmers.
Two DDS Formats Are Better Than One
As I stated earlier, most of the work in a subfile application is accomplished in the DDS. For every subfile you describe in your DDS, you're required to use two format types: a subfile record format (SFL) and a subfile control record format (SFLCTL). The subfile record format is used much as a record format is for a physical file. It defines the fields contained in a row of a subfile, describes the definition and attributes of those fields, and holds the actual data.
Unlike a physical file, however, a subfile record format is used only in memory, and only for the duration of the job. Once the program using the subfile ends, the data in that subfile is gone. Individual subfile records are read from, written to, and updated to the subfile by accessing the subfile record format in your program. The SFL keyword is required in your DDS to define a subfile record, just as the RECORD keyword is used for a typical display record format.
The subfile control record format describes the heading information for the subfile and controls how the subfile is going to perform. It's here that you define the size of the subfile, as well as the conditions under which the subfile will be cleared, initialized, displayed, and ended. The subfile control record format is unique because it controls aspects of a subfile in a way that other files aren't controlled. For example, you control the size of a physical file during compilation or by using the Change Physical File (CHGPF) command. You do not determine it in the DDS. Your program will operate the subfile control record format directly when it performs functions to the whole subfile, not individual records. Actions such as initializing, clearing, and displaying are accomplished in this fashion.
Four keywords are required in the subfile control record format:
1. The subfile control (SFLCTL) keyword identifies the subfile control record format, much as the record (RECORD) keyword identifies a typical display record format. The SFLCTL keyword also identifies the subfile record format that must immediately precede it.
2. The subfile size (SFLSIZ) keyword specifies the initial number of records the subfile may contain.
3. The subfile page (SFLPAG) keyword specifies the number of records that one screen of data may contain.
4. The subfile display (SFLDSP) keyword specifies when the subfile is displayed.
Note that the first requirement states that the SFLCTL keyword identifies the subfile record format that precedes it. Therefore, the SFL record format must immediately precede the SFLCTL format. The individual keywords available in each format can be placed in any order, but they must exist at the top of the format, before any constant or field definitions.
DDS and RPG Working as One
Once you code and compile the DDS for a subfile, you are ready to use that subfile in your RPG program. Your first three actions will be initializing, loading, and displaying your subfile. There are other things you can, and will, do to a subfile, but let's start with that foundation, and build up from there.
Before loading a subfile, you might want to clear or initialize it for use. Clearing or initializing takes effect when you write to the subfile control record format with the appropriate conditioning indicator set on, using the RPG WRITE operation. This isn't a requirement for the introductory load in your program, but as you create more complex subfile applications, you'll need to know the difference between clearing and initializing a subfile.
Let's return to the DDS for a moment. To clear a subfile, use the subfile clear (SFLCLR) keyword. This removes all records from the subfile. However, it does not remove them from the display until the next time the subfile is written to the screen by your program. This is different from the subfile initialize (SFLINZ) keyword, which will set all the records in your subfile to their default value. If you have no default value set in your DDS, numeric fields will default to zeros and character fields will default to blanks. Suppose your subfile size keyword is set to 100. If you clear it, instead of having an empty subfile, you will have 100 records, each initialized with its default value. I will talk more about SFLINZ later on, so don't worry if you don't quite get it yet. In short, the decision about whether to use SFLCLR or SFLINZ is determined by the role of your program.
You can load your subfile in a few different ways. The most common way is to retrieve data from one or more data files and write that data to the subfile record format, using the RPG WRITE operation. You might also choose to write only enough records to fit on one page (as determined by SFLPAG), and write more only if the user requests more; write all the desired records to the subfile before displaying the screen to the user; or use a combination of both. Still another way to load your subfile is to initialize the subfile first using the SFLINZ keyword, display the subfile with its default values to the screen, and allow the user to type data into the subfile from the screen.
As we go along in this book, I will show you examples of all these techniques because they are coded quite differently. I will also explain when to use which method.
Once your subfile is loaded or initialized, you're ready to display it. Depending on how you created your DDS, your subfile can take on many looks. No matter which form it takes, however, you only need one line of RPG code to display it.
Let's look at the code for a basic subfile program that displays a list of names. These could be the names of customers, salespeople, or employees; for our purpose, we'll just use generic names. Take notice of how much work is done with very little RPG code. Be careful, though. Once you understand this code, there's no turning back — you're on your way to becoming a subfile programmer.
Oh Goody! Some Code
The complete source for this example at is available at http://www.mc-store.com/5104. html, including compile information for a physical file (SFL001PF), a logical file (SFL001LF), a display file (SFL001DF), and the RPG (SFL001RG). To make this example work, you need to compile the three source members in order: first SFL001PF, then SFL001DF, and finally SFL001RG. In real life, it might not always matter whether you compile the data file before the display file, since the data file will probably already exist. In this example, however, I reference the physical file field information in my display file. As a result, the physical file needs to be created first. Figure 1.2 shows the code for the physical file.
After creating the physical file, you'll need some sort of tool to get data into it. One way is to create a subfile program to enter the data, but that's what you'll learn later in this book. For now, you can use the IBM i operating system's (OS's) native Data File Utility (DFU) or any third-party package your shop already owns. To start DFU, run the command UPDDTA FILE(SFL001PF), or take Option 18 next to SFL001PF if you're using the Work with Objects Using Programming Development Manager (PDM) view.
The DDS for the physical file is pretty self-explanatory, so I will leave it alone. I'm going to start with the DDS for the display file. This is really where most of the work related to subfiles is accomplished. As I mentioned in the introduction, I'm assuming you have some basic knowledge of DDS. With that, let's jump to the subfile record format, signified by a record name of SFL1 and the record-level keyword of SFL.SFL1 is where you define the fields contained in each individual subfile record. It is also the format that will be written to in your RPG program. This is the format that will actually contain the subfile data.
The example in Figure 1.3 describes four fields: DBLNAM, DBFNAM, DBMINI, and DBNNAM. These fields are referenced from the physical file SFL001PF. It's important to notice here that these fields are defined as output only (indicated by the "O" in position 38), and they are set to start on row 5. That means the list of data to be displayed on the screen will start on row 5.
Each field has its own starting column number. DBLNAM starts in column 2, DBFNAM starts in column 26, and so on. The column settings let you place the fields across the row in whatever fashion you want, just as you would with a printed report. That's about it for the subfile record format.
You have defined your field's size and usage, and you have designed the layout of each field. The next record format is the subfile control record format, signified by the record name SF1CTL and the keyword SFLCTL. The subfile control format must come directly after the subfile record format. It's always distinguished by the SFLCTL keyword. Even though it has to come directly after the SFL format, you still must place the name of the subfile record format, in this case SFL1, within parentheses next to the SFLCTL keyword. The DDS for SF1CTL is shown in Figure 1.4. The subfile record format is where you define everything about the individual record, while the subfile control record format is where you define everything about the subfile as a whole. You have two steps to take in the subfile control record format:
1. Define the subfile characteristics with a series of keywords.
2. Define any fields that will exist in the heading of the subfile.
The fields usually include column headings for the subfile records, as well as a title, program name, and date and time. However, they can also include input-capable fields, discussed in the next chapter. It's important to note that fields defined in the subfile control format are not part of the scrollable, subfile records.
There is no requirement for the content of the fields in the subfile control record format, but there is a limit as to where they can be placed. Remember that the SFL record format started on row 5. That means that fields defined in the SFLCTL record format must not be placed anywhere below row 4. Now, looking at the example, let's examine the keywords used. They can be placed in any order within the SFLCTL record format, but they must also be entered before any fields or constants are defined.
The first thing I do in Figure 1.4 is define the subfile size (SFLSIZ) and subfile page size (SFLPAG). The SFLSIZ is set to 500. That means the initial number of records my subfile can contain is 500. I can extend the number contained in the SFLSIZ keyword up to a maximum of 9,999 records, but I will cover that in the next chapter.
The SFLPAG keyword determines how many records can fit on one page. I set mine to 17, meaning that each screen of data will contain a maximum of 17 records. You're limited on this parameter only by the number of records that can be physically displayed on a screen.
The OVERLAY keyword tells the display file to display the screen on top of what is already displayed — overlaying anything that is already there, but not erasing it. The subfile display (SFLDSP) keyword displays the subfile on the screen. It's conditioned, in this case, by N32, which means it will display only when indicator 32 is set off. You're not required to condition the SFLDSP keyword. I condition it in this example (and in most of my subfile programs, for that matter) because I want to stop the subfile from displaying if there are no records in it. You'll get a runtime error if you try to display a subfile with no records in it. There are a number of ways to handle the no-record situation, but I choose to set on indicator 32. This stops the subfile, and subsequent errors, from being displayed.
The next keyword is the subfile display control (SFLDSPCTL). This keyword allows you to display the control record format. The conditioning indicator on the SFLDSPCTL keyword, N31, conditions when the SFLCTL record format is to be displayed. In this case, the SFLCTL record format will be displayed only when it's told to do so by the RPG program, and when indicator 31 is off. This keyword isn't required if there are no fields to display or function keys to control in the format. Many keywords change the characteristics of the subfile, but these changes aren't seen until either the SFLDSP or the SFLDSPCTL keyword is activated.
The next keyword is the subfile clear (SFLCLR), which clears the subfile of its entries. It provides you with an empty subfile just waiting to have records written to it. Notice that I used the opposite conditioning indicator for the SFLDSPCTL keyword. I did this because the same RPG operation (WRITE) is used to display the control record format and clear the subfile. I probably don't want to clear the subfile at the same time I want to display it, so I use the same indicator. When indicator 31 is off, I want to display the subfile. When it's on, I want to clear it.
The subfile end (SFLEND) keyword tells the user there are more records in the subfile. A conditioning indicator is required when you're using the SFLEND keyword. In this case, indicator 90 conditions the SFLEND keyword. The *MORE parameter is just one of the valid parameters used with the SFLEND keyword. (The others will be discussed later.) It will cause the screen to show the word "More ..." at the bottom-right corner of the last subfile record on the page. When the subfile is on its last page, the word "Bottom" replaces "More ..." to indicate that this is the end of the subfile. SFLEND specified by itself, with no parameter, will cause the screen to display a plus sign ("+") in the lower-right corner when there are further pages to be displayed. When the subfile is on its last page, the plus sign is replaced by a blank.
Now that all the keywords have been defined, you can define any fields you need for your subfile control record format. In Figure 1.4, I describe some basic column headings: a title, the date, and the time. Because this is the same technique you would use to define headings on any display record format, it does not warrant discussion here.
Notice one particular field defined in the SFLCTL record format: RRN1, which will be used as my subfile relative record number. I define it as a 4-digit signed numeric, with no decimal places. (Remember that there is a maximum of 9,999 records in a subfile.) The "H" in position 38 indicates that this is a hidden field. I'm not going to display this field anywhere on the screen, but it's extremely important because it will keep track of which subfile record I'm working with.
The last record format defined in this DDS is the function key line. On line 23 of the display, I will list the function keys available to the user. This is the reason I use the OVERLAY keyword in the SFLCTL record format. I will first write the FKEY1 format, which will display on line 23, and then OVERLAY the FKEY1 format, but not erase it.
(Continues...)
Excerpted from Subfiles in Free-Format RPG by Kevin Vandever. Copyright © 2011 Kevin Vandever. Excerpted by permission of MC Press.
All rights reserved. No part of this excerpt may be reproduced or reprinted without permission in writing from the publisher.
Excerpts are provided by Dial-A-Book Inc. solely for the personal use of visitors to this web site.
Table of Contents
Contents
Acknowledgements,Preface,
Introduction,
About This Book,
What Does It Mean for Your Career?,
Chapter 1: The Specifics on Subfiles,
Chapter 2: A Subfile Type for Every Occasion,
Chapter 3: Modifying a Subfile (Change Is Good),
Chapter 4: Window Subfiles: Who Needs a PC to Have Scrollable Windows?,
Chapter 5: Message Subfiles: What's That Plus Sign Next to My Message?,
Chapter 6: Displaying Multiple Subfiles on a Screen,
Chapter 7: Subfiles and Data Queues — A Perfect Combination,
Chapter 8: Embedded SQL and Dynamic Sorting Subfiles,
Chapter 9: No Aversion to Recursion,
Index,