By emphasizing practical knowledge and skills over theory, author Allen Downey shows you how to use data structures to implement efficient algorithms, and then analyze and measure their performance. You’ll explore the important classes in the Java collections framework (JCF), how they’re implemented, and how they’re expected to perform. Each chapter presents hands-on exercises supported by test code online.
- Use data structures such as lists and maps, and understand how they work
- Build an application that reads Wikipedia pages, parses the contents, and navigates the resulting data tree
- Analyze code to predict how fast it will run and how much memory it will require
- Write classes that implement the Map interface, using a hash table and binary search tree
- Build a simple web search engine with a crawler, an indexer that stores web page contents, and a retriever that returns user query results
Other books by Allen Downey include Think Java, Think Python, Think Stats, and Think Bayes.
By emphasizing practical knowledge and skills over theory, author Allen Downey shows you how to use data structures to implement efficient algorithms, and then analyze and measure their performance. You’ll explore the important classes in the Java collections framework (JCF), how they’re implemented, and how they’re expected to perform. Each chapter presents hands-on exercises supported by test code online.
- Use data structures such as lists and maps, and understand how they work
- Build an application that reads Wikipedia pages, parses the contents, and navigates the resulting data tree
- Analyze code to predict how fast it will run and how much memory it will require
- Write classes that implement the Map interface, using a hash table and binary search tree
- Build a simple web search engine with a crawler, an indexer that stores web page contents, and a retriever that returns user query results
Other books by Allen Downey include Think Java, Think Python, Think Stats, and Think Bayes.
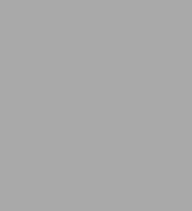
Think Data Structures: Algorithms and Information Retrieval in Java
155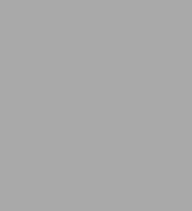
Think Data Structures: Algorithms and Information Retrieval in Java
155Product Details
ISBN-13: | 9781491972397 |
---|---|
Publisher: | O'Reilly Media, Incorporated |
Publication date: | 08/04/2017 |
Pages: | 155 |
Product dimensions: | 6.90(w) x 9.10(h) x 0.50(d) |