If you’re just learning how to program, Julia is an excellent JIT-compiled, dynamically typed language with a clean syntax. This hands-on guide uses Julia 1.0 to walk you through programming one step at a time, beginning with basic programming concepts before moving on to more advanced capabilities, such as creating new types and multiple dispatch.
Designed from the beginning for high performance, Julia is a general-purpose language ideal for not only numerical analysis and computational science but also web programming and scripting. Through exercises in each chapter, you’ll try out programming concepts as you learn them. Think Julia is perfect for students at the high school or college level as well as self-learners and professionals who need to learn programming basics.
- Start with the basics, including language syntax and semantics
- Get a clear definition of each programming concept
- Learn about values, variables, statements, functions, and data structures in a logical progression
- Discover how to work with files and databases
- Understand types, methods, and multiple dispatch
- Use debugging techniques to fix syntax, runtime, and semantic errors
- Explore interface design and data structures through case studies
If you’re just learning how to program, Julia is an excellent JIT-compiled, dynamically typed language with a clean syntax. This hands-on guide uses Julia 1.0 to walk you through programming one step at a time, beginning with basic programming concepts before moving on to more advanced capabilities, such as creating new types and multiple dispatch.
Designed from the beginning for high performance, Julia is a general-purpose language ideal for not only numerical analysis and computational science but also web programming and scripting. Through exercises in each chapter, you’ll try out programming concepts as you learn them. Think Julia is perfect for students at the high school or college level as well as self-learners and professionals who need to learn programming basics.
- Start with the basics, including language syntax and semantics
- Get a clear definition of each programming concept
- Learn about values, variables, statements, functions, and data structures in a logical progression
- Discover how to work with files and databases
- Understand types, methods, and multiple dispatch
- Use debugging techniques to fix syntax, runtime, and semantic errors
- Explore interface design and data structures through case studies
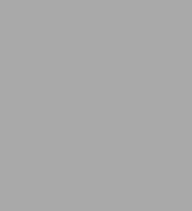
Think Julia: How to Think Like a Computer Scientist
298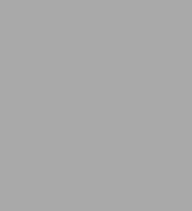
Think Julia: How to Think Like a Computer Scientist
298Related collections and offers
Product Details
ISBN-13: | 9781492044987 |
---|---|
Publisher: | O'Reilly Media, Incorporated |
Publication date: | 04/05/2019 |
Sold by: | Barnes & Noble |
Format: | eBook |
Pages: | 298 |
File size: | 11 MB |
Note: | This product may take a few minutes to download. |