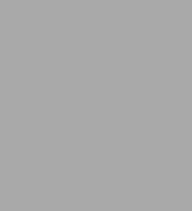
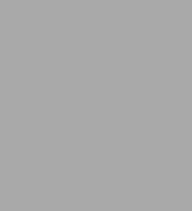
Paperback
-
PICK UP IN STORECheck Availability at Nearby Stores
Available within 2 business hours
Related collections and offers
Overview
This, the first volume in Randall Hyde's Write Great Code series, dives into machine organization without the extra overhead of learning assembly language programming. Written for high-level language programmers, Understanding the Machine fills in the low-level details of machine organization that are often left out of computer science and engineering courses.
Learn:
Great code is efficient code. But before you can write truly efficient code, you must understand how computer systems execute programs and how abstractions in programming languages map to the machine's low-level hardware. After all, compilers don't write the best machine code; programmers do. This book gives you the foundation upon which all great software is built.
NEW IN THIS EDITION, COVERAGE OF:
Product Details
ISBN-13: | 9781718500365 |
---|---|
Publisher: | No Starch Press |
Publication date: | 07/31/2020 |
Pages: | 472 |
Sales rank: | 490,147 |
Product dimensions: | 7.00(w) x 9.10(h) x 1.20(d) |
About the Author
Read an Excerpt
WHAT YOU NEED TO KNOW TO WRITE GREAT CODE
The Write Great Code (WGC) series will teach you how to write code you can be proud of; code that will impress other programmers, satisfy customers, and prove popular with users; and code that people (customers, your boss, and so on) won’t mind paying top dollar to obtain.
In general, the books in the WGC series will discuss how to write software that achieves legendary status, eliciting the awe and admiration of other programmers.
1.1 The Write Great Code Series
Write Great Code, Volume 1: Understanding the Machine (WGC1 hereafter) is the first of six books in the WGC series. Writing great code requires a combination of knowledge, experience, and skill that programmers usually obtain only after years of mistakes and discoveries. The purpose of this series is to share with both new and experienced programmers a few decades’ worth of observations and experience. I hope that these books will help reduce the time and frustration it takes to learn things “the hard way.”
This book, WGC1, fills in the low-level details that are often skimmed over in a typical computer science or engineering curriculum. These details are the foundation for the solutions to many problems, and you can’t write efficient code without this information. Though I’m attempting to keep each book independent, WGC1 might be considered a prerequisite for the subsequent volumes in the series.
Write Great Code, Volume 2: Thinking Low-Level, Writing High-Level (WGC2) immediately applies the knowledge from this book. WGC2 will teach you how to analyze code written in a high-level language to determine the quality of the machine code that a compiler would generate for it. Optimizing compilers don’t always generate the best machine code possible—the statements and data structures you choose in your source files can have a big impact on the efficiency of the compiler’s output. WGC2 will teach you how to write efficient code without resorting to assembly language.
There are many attributes of great code besides efficiency, and the third book in this series, Write Great Code, Volume 3: Engineering Software (WGC3), will cover some of those. WGC3 will discuss software development metaphors, development methologies, types of developers, system documentation, and the Unified Modeling Language (UML). WGC3 provides the basis for personal software engineering.
Great code begins with a great design. Write Great Code, Volume 4: Designing Great Code (WGC4), will describe the process of analysis and design (both structured and object-oriented). WGC4 will teach you how to translate an initial concept into a working design for your software systems.
Write Great Code, Volume 5: Great Coding (WGC5) will teach you how to create source code that others can easily read and maintain, as well as how to improve your productivity without the burden of the “busy work” that many software engineering books discuss.
Great code works. Therefore, I’d be remiss not to include a book on testing, debugging, and quality assurance. Few programmers properly test their code. This generally isn’t because they find testing boring or beneath them, but because they don’t know how to test their programs, eradicate defects, and ensure the quality of their code. To help overcome this problem, Write Great Code, Volume 6: Testing, Debugging, and Quality Assurance (WGC6) will describe how to efficiently test your applications without all the drudgery engineers normally associate with this task.
1.2 What This Book Covers
In order to write great code, you need to know how to write efficient code, and to write efficient code, you must understand how computer systems execute programs and how abstractions in programming languages map to the low-level hardware capabilities of the machine.
In the past, learning great coding techniques has required learning assembly language. While this isn’t a bad approach, it’s overkill. Learning assembly language involves learning two related subjects: machine organization, and programming in assembly language. The real benefits of learning assembly language come from the machine organization component. Thus, this book focuses solely on machine organization so you can learn to write great code without the overhead of also learning assembly language.
Machine organization is a subset of computer architecture that covers low-level data types, internal CPU organization, memory organization and access, low-level machine operations, mass storage organization, peripherals, and how computers communicate with the rest of the world. This book concentrates on those parts of computer architecture and machine organization that are visible to the programmer or are helpful for understanding why system architects chose a particular system design. The goal of learning machine organization, and of this book, is not to enable you to design your own CPU or computer system, but to equip you to make the most efficient use of existing computer designs. Let’s do a quick run-through of the specific topics we’ll cover.
Chapters 2, 4, and 5 deal with basic computer data representation— how computers represent signed and unsigned integer values, characters, strings, character sets, real values, fractional values, and other numeric and non-numeric quantities. Without a solid grasp of how computers represent these various data types internally, it’ll be difficult for you to understand why some operations that use these data types are so inefficient.
Chapter 3 discusses binary arithmetic and bit operations used by most modern computer systems. It also offers several insights into how you can write better code by using arithmetic and logical operations in ways not normally taught in beginning programming courses. Learning these kinds of standard “tricks” is part of how you become a great programmer.
Chapter 6 introduces memory, discussing how the computer accesses its memory and describing characteristics of memory performance. This chapter also covers various machine code addressing modes, which CPUs use to access different types of data structures in memory. In modern applications, poor performance often occurs because the programmer, unaware of the ramifications of memory access in their programs, creates bottlenecks. Chapter 6 addresses many of these ramifications.
Chapter 7 returns to data types and representation by covering composite data types and memory objects: pointers, arrays, records, structures, and unions. All too often, programmers use large composite data structures without even considering the memory and performance impact of doing so. The low-level description of these high-level composite data types will make clear their inherent costs, so you can use them sparingly and wisely.
Chapter 8 discusses Boolean logic and digital design. This chapter provides the mathematical and logical background you’ll need to under- stand the design of CPUs and other computer system components. In particular, this chapter discusses how to optimize Boolean expressions, such as those found in common high-level programming language statements like if and while.
Continuing the hardware discussion from Chapter 8, Chapter 9 discusses CPU architecture. A basic understanding of CPU design and operation is essential if you want to write great code. By writing your code in a manner consistent with how a CPU will execute it, you’ll get much better performance using fewer system resources.
Chapter 10 discusses CPU instruction set architecture. Machine instructions are the primitive units of execution on any CPU, and the duration of program execution is directly determined by the number and type of machine instructions the CPU must process. Learning how computer architects design machine instructions can provide valuable insight into why certain operations take longer to execute than others. Once you understand the limitations of machine instructions and how the CPU interprets them, you can use this information to turn mediocre code sequences into great ones.
Chapter 11 returns to the subject of memory, covering memory architecture and organization. This chapter is especially important for anyone wanting to write fast code. It describes the memory hierarchy and how to maximize the use of the cache and other fast memory components. You’ll learn about thrashing and how to avoid low-performance memory access in your applications.
Chapters 12 through 15 describe how computer systems communicate with the outside world. Many peripheral (input/output) devices operate at much lower speeds than the CPU and memory. You could write the fastest-executing sequence of instructions possible, and your application would still run slowly because you didn’t understand the limitations of the I/O devices in your system. These four chapters discuss generic I/O ports, system buses, buffering, handshaking, polling, and interrupts. They also explain how to efficiently use many popular PC peripheral devices, including keyboards, parallel (printer) ports, serial ports, disk drives, tape drives, flash storage, SCSI, IDE/ATA, USB, and sound cards.
1.3 Assumptions This Book Makes
This book was written with certain assumptions about your prior knowledge. You’ll reap the greatest benefit from this material if your skill set matches the following:
• You should be reasonably competent in at least one modern programming language. This includes C/C++, C#, Java, Swift, Python, Pascal/ Delphi (Object Pascal), BASIC, and assembly, as well as languages like Ada, Modula-2, and FORTRAN.
• Given a small problem description, you should be capable of working through the design and implementation of a software solution for that problem. A typical semester or quarter course at a college or university (or several months’ experience on your own) should be sufficient back- ground for this book.
At the same time, this book is not language specific; its concepts transcend whatever programming language(s) you’re using. Furthermore, this book does not assume that you use or know any particular language. To help make the examples more accessible, the programming examples rotate among several languages. This book explains exactly how the example code operates so that even if you’re unfamiliar with the specific programming language, you’ll be able to understand its operation by reading the accompanying description.
This book uses the following languages and compilers in various examples:
• C/C++: GCC, Microsoft’s Visual C++
• Pascal: Embarcadero’s Delphi, Free Pascal
• Assembly language: Microsoft’s MASM, HLA (High-Level Assembly), Gas (the Gnu Assembler; on the PowerPC and ARM)
• Swift 5 (Apple)
• Java (v6 or later)
• BASIC: Microsoft’s Visual Basic
Often, the examples appear in multiple languages, so it’s usually safe to ignore a specific example if you don’t understand the syntax of the language it uses.
Table of Contents
Chapter 1: What You Need to Know to Write Great CodeChapter 2: Numeric Representation
Chapter 3: Binary Arithmetic and Bit Operations
Chapter 4: Floating-Point Representation
Chapter 5: Character Representation
Chapter 6: Memory Organization and Access
Chapter 7: Composite Data Types and Memory Objects
Chapter 8: Boolean Logic and Digital Design
Chapter 9: CPU Architecture
Chapter 10: Instruction Set Architecture
Chapter 11: Memory Architecture and Organization
Chapter 12: Input and Output
Chapter 13: Computer Peripheral Buses
Chapter 14: Mass Storage Devices and Filesystems
Chapter 15: Miscellaneous Input and Output Devices
Afterword: Thinking Low-Level, Writing High-Level
Appendix A: ASCII Character Set
Glossary